
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ns_sha256.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2006-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 /** 00015 * \file ns_sha256.h 00016 * 00017 * \brief SHA-256 cryptographic hash function 00018 * 00019 * This file is derived from sha256.h in mbed TLS 2.3.0. 00020 * 00021 * This file provides an API very similar to mbed TLS, either implemented 00022 * locally, or by calling mbed TLS, depending on NS_USE_EXTERNAL_MBED_TLS. 00023 * 00024 * Differences from mbed TLS: 00025 * 00026 * a) ns_ prefix instead of mbedtls_; 00027 * b) Pointers are void * instead of unsigned char * to avoid type clashes; 00028 * c) SHA-224 not supported; 00029 * d) Ability to output truncated hashes. 00030 */ 00031 00032 00033 #ifndef NS_SHA256_H_ 00034 #define NS_SHA256_H_ 00035 00036 #ifdef NS_USE_EXTERNAL_MBED_TLS 00037 00038 #include <string.h> 00039 #include "mbedtls/sha256.h" 00040 00041 typedef mbedtls_sha256_context ns_sha256_context; 00042 00043 static inline void ns_sha256_init(ns_sha256_context *ctx) 00044 { 00045 mbedtls_sha256_init(ctx); 00046 } 00047 00048 static inline void ns_sha256_free(ns_sha256_context *ctx) 00049 { 00050 mbedtls_sha256_free(ctx); 00051 } 00052 00053 static inline void ns_sha256_clone(ns_sha256_context *dst, 00054 const ns_sha256_context *src) 00055 { 00056 mbedtls_sha256_clone(dst, src); 00057 } 00058 00059 static inline void ns_sha256_starts(ns_sha256_context *ctx) 00060 { 00061 mbedtls_sha256_starts(ctx, 0); 00062 } 00063 00064 static inline void ns_sha256_update(ns_sha256_context *ctx, const void *input, 00065 size_t ilen) 00066 { 00067 mbedtls_sha256_update(ctx, input, ilen); 00068 } 00069 00070 static inline void ns_sha256_finish(ns_sha256_context *ctx, void *output) 00071 { 00072 mbedtls_sha256_finish(ctx, output); 00073 } 00074 00075 static inline void ns_sha256(const void *input, size_t ilen, void *output) 00076 { 00077 mbedtls_sha256(input, ilen, output, 0); 00078 } 00079 00080 /* Extensions to standard mbed TLS - output the first bits of a hash only */ 00081 /* Number of bits must be a multiple of 32, and <=256 */ 00082 static inline void ns_sha256_finish_nbits(ns_sha256_context *ctx, void *output, unsigned obits) 00083 { 00084 if (obits == 256) { 00085 mbedtls_sha256_finish(ctx, output); 00086 } else { 00087 uint8_t sha256[32]; 00088 mbedtls_sha256_finish(ctx, sha256); 00089 memcpy(output, sha256, obits / 8); 00090 } 00091 } 00092 00093 static inline void ns_sha256_nbits(const void *input, size_t ilen, void *output, unsigned obits) 00094 { 00095 if (obits == 256) { 00096 mbedtls_sha256(input, ilen, output, 0); 00097 } else { 00098 uint8_t sha256[32]; 00099 mbedtls_sha256(input, ilen, sha256, 0); 00100 memcpy(output, sha256, obits / 8); 00101 } 00102 } 00103 00104 #else /* NS_USE_EXTERNAL_MBED_TLS */ 00105 00106 #include <stddef.h> 00107 #include <stdint.h> 00108 00109 /** 00110 * \brief SHA-256 context structure 00111 */ 00112 typedef struct 00113 { 00114 uint32_t total[2]; /*!< number of bytes processed */ 00115 uint32_t state[8]; /*!< intermediate digest state */ 00116 unsigned char buffer[64]; /*!< data block being processed */ 00117 } 00118 ns_sha256_context; 00119 00120 /** 00121 * \brief Initialize SHA-256 context 00122 * 00123 * \param ctx SHA-256 context to be initialized 00124 */ 00125 void ns_sha256_init( ns_sha256_context *ctx ); 00126 00127 /** 00128 * \brief Clear SHA-256 context 00129 * 00130 * \param ctx SHA-256 context to be cleared 00131 */ 00132 void ns_sha256_free( ns_sha256_context *ctx ); 00133 00134 /** 00135 * \brief Clone (the state of) a SHA-256 context 00136 * 00137 * \param dst The destination context 00138 * \param src The context to be cloned 00139 */ 00140 void ns_sha256_clone( ns_sha256_context *dst, 00141 const ns_sha256_context *src ); 00142 00143 /** 00144 * \brief SHA-256 context setup 00145 * 00146 * \param ctx context to be initialized 00147 */ 00148 void ns_sha256_starts( ns_sha256_context *ctx ); 00149 00150 /** 00151 * \brief SHA-256 process buffer 00152 * 00153 * \param ctx SHA-256 context 00154 * \param input buffer holding the data 00155 * \param ilen length of the input data 00156 */ 00157 void ns_sha256_update( ns_sha256_context *ctx, const void *input, 00158 size_t ilen ); 00159 00160 /** 00161 * \brief SHA-256 final digest 00162 * 00163 * \param ctx SHA-256 context 00164 * \param output SHA-256 checksum result 00165 */ 00166 void ns_sha256_finish( ns_sha256_context *ctx, void *output ); 00167 00168 /** 00169 * \brief SHA-256 final digest 00170 * 00171 * \param ctx SHA-256 context 00172 * \param output SHA-256 checksum result 00173 * \param obits Number of bits of to output - must be multiple of 32 00174 */ 00175 void ns_sha256_finish_nbits( ns_sha256_context *ctx, 00176 void *output, unsigned obits ); 00177 00178 /** 00179 * \brief Output = SHA-256( input buffer ) 00180 * 00181 * \param input buffer holding the data 00182 * \param ilen length of the input data 00183 * \param output SHA-256 checksum result 00184 */ 00185 void ns_sha256( const void *input, size_t ilen, 00186 void *output ); 00187 00188 /** 00189 * \brief Output = SHA-256( input buffer ) 00190 * 00191 * \param input buffer holding the data 00192 * \param ilen length of the input data 00193 * \param output SHA-256 checksum result 00194 * \param obits Number of bits of to output - must be multiple of 32 00195 */ 00196 void ns_sha256_nbits( const void *input, size_t ilen, 00197 void *output, unsigned obits ); 00198 00199 #endif /* NS_USE_EXTERNAL_MBED_TLS */ 00200 00201 00202 #endif /* NS_SHA256_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
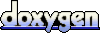