
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ns_nvm_helper.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 */ 00004 00005 /** 00006 * NanoStack NVM helper functions to read, write and delete key-value pairs to platform NVM. 00007 * 00008 * Client can use following methods: 00009 * -ns_nvm_data_write to write data to a key in platform NVM 00010 * -ns_nvm_data_read to read data from key in platform NVM 00011 * -ns_nvm_key_delete to delete a key from platform NVM 00012 * 00013 * If a API call returns NS_NVM_OK then a provided callback function will be called 00014 * and status argument indicates success or failure of the operation. If API call 00015 * returns error then callback will not be called. 00016 * 00017 * When client writes data this module will: 00018 * -initialize the NVM if not already initialized 00019 * -(re)create the key with a given size 00020 * -write data to the key 00021 * -flush data to the NVM 00022 * 00023 * When client reads data this module will: 00024 * -initialize the NVM if not initialized 00025 * -read data from the key 00026 * 00027 * When client deletes a key this module will: 00028 * -initialize the NVM if not initialized 00029 * -delete the key from NVM 00030 */ 00031 00032 /* 00033 * API function and callback function return statuses 00034 */ 00035 #define NS_NVM_OK 0 00036 #define NS_NVM_DATA_NOT_FOUND -1 00037 #define NS_NVM_ERROR -2 00038 #define NS_NVM_MEMORY -3 00039 00040 /** 00041 * callback type for NanoStack NVM 00042 */ 00043 typedef void (ns_nvm_callback)(int status, void *context); 00044 00045 /** 00046 * \brief Delete key from NVM 00047 * 00048 * \param callback function to be called when key deletion is ready 00049 * \param key_name Name of the key to be deleted from NVM 00050 * \param context argument will be provided as an argument when callback is called 00051 * 00052 * \return NS_NVM_OK if key deletion is in progress and callback will be called 00053 * \return NS_NVM_ERROR in error case, callback will not be called 00054 * \return provided callback function will be called with status indicating success or failure. 00055 */ 00056 int ns_nvm_key_delete(ns_nvm_callback *callback, const char *key_name, void *context); 00057 00058 /** 00059 * \brief Read data from NVM 00060 * 00061 * \param callback function to be called when data is read 00062 * \param key_name Name of the key whose data will be read 00063 * \param buf buffer where data will be stored 00064 * \param buf_len address of variable containing provided buffer length 00065 * \param context argument will be provided as an argument when callback is called 00066 * 00067 * \return NS_NVM_OK if read is in progress and callback will be called 00068 * \return NS_NVM_ERROR in error case, callback will not be called 00069 * \return provided callback function will be called with status indicating success or failure. 00070 */ 00071 int ns_nvm_data_read(ns_nvm_callback *callback, const char *key_name, uint8_t *buf, uint16_t *buf_len, void *context); 00072 00073 /** 00074 * \brief Write data to NVM 00075 * 00076 * \param callback function to be called when data writing is completed 00077 * \param key_name Name of the key whose data will be read 00078 * \param buf buffer where data will be stored 00079 * \param buf_len address of variable containing provided buffer length 00080 * \param context argument will be provided as an argument when callback is called 00081 * 00082 * \return NS_NVM_OK if read is in progress and callback will be called 00083 * \return NS_NVM_ERROR in error case, callback will not be called 00084 * \return provided callback function will be called with status indicating success or failure. 00085 */ 00086 int ns_nvm_data_write(ns_nvm_callback *callback, const char *key_name, uint8_t *buf, uint16_t *buf_len, void *context);
Generated on Sun Jul 17 2022 08:25:28 by
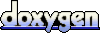