
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
netifapi.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * netif API (to be used from non-TCPIP threads) 00004 */ 00005 00006 /* 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 * 00029 * This file is part of the lwIP TCP/IP stack. 00030 * 00031 */ 00032 #ifndef LWIP_HDR_NETIFAPI_H 00033 #define LWIP_HDR_NETIFAPI_H 00034 00035 #include "lwip/opt.h" 00036 00037 #if LWIP_NETIF_API /* don't build if not configured for use in lwipopts.h */ 00038 00039 #include "lwip/sys.h" 00040 #include "lwip/netif.h" 00041 #include "lwip/dhcp.h" 00042 #include "lwip/autoip.h" 00043 #include "lwip/priv/tcpip_priv.h" 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 #if LWIP_MPU_COMPATIBLE 00050 #define NETIFAPI_IPADDR_DEF(type, m) type m 00051 #else /* LWIP_MPU_COMPATIBLE */ 00052 #define NETIFAPI_IPADDR_DEF(type, m) const type * m 00053 #endif /* LWIP_MPU_COMPATIBLE */ 00054 00055 typedef void (*netifapi_void_fn)(struct netif *netif); 00056 typedef err_t (*netifapi_errt_fn)(struct netif *netif); 00057 00058 struct netifapi_msg { 00059 struct tcpip_api_call_data call; 00060 struct netif *netif; 00061 union { 00062 struct { 00063 #if LWIP_IPV4 00064 NETIFAPI_IPADDR_DEF(ip4_addr_t, ipaddr); 00065 NETIFAPI_IPADDR_DEF(ip4_addr_t, netmask); 00066 NETIFAPI_IPADDR_DEF(ip4_addr_t, gw); 00067 #endif /* LWIP_IPV4 */ 00068 void *state; 00069 netif_init_fn init; 00070 netif_input_fn input; 00071 } add; 00072 struct { 00073 netifapi_void_fn voidfunc; 00074 netifapi_errt_fn errtfunc; 00075 } common; 00076 } msg; 00077 }; 00078 00079 00080 /* API for application */ 00081 err_t netifapi_netif_add(struct netif *netif, 00082 #if LWIP_IPV4 00083 const ip4_addr_t *ipaddr, const ip4_addr_t *netmask, const ip4_addr_t *gw, 00084 #endif /* LWIP_IPV4 */ 00085 void *state, netif_init_fn init, netif_input_fn input); 00086 00087 #if LWIP_IPV4 00088 err_t netifapi_netif_set_addr(struct netif *netif, const ip4_addr_t *ipaddr, 00089 const ip4_addr_t *netmask, const ip4_addr_t *gw); 00090 #endif /* LWIP_IPV4*/ 00091 00092 err_t netifapi_netif_common(struct netif *netif, netifapi_void_fn voidfunc, 00093 netifapi_errt_fn errtfunc); 00094 00095 /** @ingroup netifapi_netif */ 00096 #define netifapi_netif_remove(n) netifapi_netif_common(n, netif_remove, NULL) 00097 /** @ingroup netifapi_netif */ 00098 #define netifapi_netif_set_up(n) netifapi_netif_common(n, netif_set_up, NULL) 00099 /** @ingroup netifapi_netif */ 00100 #define netifapi_netif_set_down(n) netifapi_netif_common(n, netif_set_down, NULL) 00101 /** @ingroup netifapi_netif */ 00102 #define netifapi_netif_set_default(n) netifapi_netif_common(n, netif_set_default, NULL) 00103 /** @ingroup netifapi_netif */ 00104 #define netifapi_netif_set_link_up(n) netifapi_netif_common(n, netif_set_link_up, NULL) 00105 /** @ingroup netifapi_netif */ 00106 #define netifapi_netif_set_link_down(n) netifapi_netif_common(n, netif_set_link_down, NULL) 00107 00108 /** 00109 * @defgroup netifapi_dhcp4 DHCPv4 00110 * @ingroup netifapi 00111 * To be called from non-TCPIP threads 00112 */ 00113 /** @ingroup netifapi_dhcp4 */ 00114 #define netifapi_dhcp_start(n) netifapi_netif_common(n, NULL, dhcp_start) 00115 /** @ingroup netifapi_dhcp4 */ 00116 #define netifapi_dhcp_stop(n) netifapi_netif_common(n, dhcp_stop, NULL) 00117 /** @ingroup netifapi_dhcp4 */ 00118 #define netifapi_dhcp_inform(n) netifapi_netif_common(n, dhcp_inform, NULL) 00119 /** @ingroup netifapi_dhcp4 */ 00120 #define netifapi_dhcp_renew(n) netifapi_netif_common(n, NULL, dhcp_renew) 00121 /** @ingroup netifapi_dhcp4 */ 00122 #define netifapi_dhcp_release(n) netifapi_netif_common(n, NULL, dhcp_release) 00123 00124 /** 00125 * @defgroup netifapi_autoip AUTOIP 00126 * @ingroup netifapi 00127 * To be called from non-TCPIP threads 00128 */ 00129 /** @ingroup netifapi_autoip */ 00130 #define netifapi_autoip_start(n) netifapi_netif_common(n, NULL, autoip_start) 00131 /** @ingroup netifapi_autoip */ 00132 #define netifapi_autoip_stop(n) netifapi_netif_common(n, NULL, autoip_stop) 00133 00134 #ifdef __cplusplus 00135 } 00136 #endif 00137 00138 #endif /* LWIP_NETIF_API */ 00139 00140 #endif /* LWIP_HDR_NETIFAPI_H */
Generated on Sun Jul 17 2022 08:25:28 by
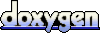