
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_polling_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2013-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file net_polling_api.h 00017 * \brief ZigBeeIP Sleepy Host Data Poll API. 00018 * 00019 * - arm_nwk_host_mode_set(), Set sleepy host new state. 00020 * - arm_nwk_host_mode_get(), Read current host state. 00021 * - net_host_enter_sleep_state_set(), Enable deep sleep state. 00022 * 00023 * The sleepy host default state is NET_HOST_FAST_POLL_MODE after bootstrap. 00024 * The stack always disables radio automatically between data polls. 00025 * The stack can also enable deep sleep when application net_host_enter_sleep_state_set(). 00026 * 00027 * A sleepy host can change the host state to normal, which saves time and resources when the client is waiting for a large amount of data. 00028 * - nwk_host_mode_set(NET_HOST_RX_ON_IDLE,0) A function call to trigger MLE handshake update automatically. 00029 * From NET_HOST_RX_ON_IDLE state back to polling state causes an MLE handshake with parent: 00030 * - nwk_host_mode_set (NET_HOST_FAST_POLL_MODE,0), Enter fast mode. 00031 * - nwk_host_mode_set (NET_HOST_SLOW_POLL_MODE,10), Enter slow poll mode by 10 seconds max data poll period. 00032 * 00033 * The stack will tell max sleepy time to the application by selecting min values from the following cases: 00034 * - Next active system timer trigger 00035 * - ND protocol next state trigger 00036 * - MLE handshake trigger 00037 * - PANA key pull trigger 00038 * - Next data poll period 00039 * 00040 */ 00041 00042 #ifndef NET_POLLING_H_ 00043 #define NET_POLLING_H_ 00044 00045 #include "ns_types.h" 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 /*! 00052 * \enum net_host_mode_t 00053 * \brief Sleepy host states. 00054 */ 00055 typedef enum net_host_mode { 00056 NET_HOST_FAST_POLL_MODE, /**< Sleepy host fast poll state, max poll period 400ms. */ 00057 NET_HOST_SLOW_POLL_MODE, /**< Sleepy host slow poll state, user-defined max period in seconds. */ 00058 NET_HOST_RX_ON_IDLE, /**< Disable polling and enable direct data flow from parent to host. */ 00059 NET_HOST_MODE_NOT_ACTIVE, /**< Host mode is not active. */ 00060 } net_host_mode_t; 00061 00062 /** 00063 * \brief Set new host state. 00064 * 00065 * \param interface_id Network interface ID. 00066 * \param mode New host state. 00067 * \param poll_time Poll time in seconds, only handled when NET_HOST_SLOW_POLL_MODE is enabled. 00068 * 00069 * Valid poll time for NET_HOST_SLOW_POLL_MODE is 0 < poll_time poll_time < 864001 (1 Day) 00070 * 00071 * \return 0, State update OK. 00072 * \return -1, Unknown state. 00073 * \return -2, Invalid time. 00074 * \return -3 MLE handshake trigger failure. 00075 * 00076 */ 00077 00078 extern int8_t arm_nwk_host_mode_set(int8_t interface_id, net_host_mode_t mode, uint32_t poll_time); 00079 /** 00080 * \brief Read current host state. 00081 * 00082 * \param interface_id Network interface ID. 00083 * \param mode A pointer to the location for saving the host state. 00084 00085 * \return 0, State read update OK. 00086 * \return -1, The host role is router or the stack is idle. 00087 * 00088 */ 00089 extern int8_t arm_nwk_host_mode_get(int8_t interface_id, net_host_mode_t *mode); 00090 00091 /** 00092 * \brief Host sleep state control. 00093 * 00094 * \param interface_id Network interface ID. 00095 * \param state >0 enables power saving between data polling. Otherwise, only radio is disabled. 00096 * 00097 * When the application wants to save more power it can call net_host_enter_sleep_state_set(nwk_interface_id, 1). 00098 * When the application wants to disable sleep it just calls net_host_enter_sleep_state_set(nwk_interface_id, 0). 00099 */ 00100 extern void arm_net_host_enter_sleep_state_set(int8_t interface_id, uint8_t state); 00101 00102 #ifdef __cplusplus 00103 } 00104 #endif 00105 00106 #endif /* NET_POLLING_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
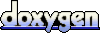