
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_load_balance_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file net_load_balance_api.h 00017 * \brief 6LoWPAN network load balance control API. 00018 */ 00019 00020 #ifndef NET_LOAD_BALANCE_API_H_ 00021 #define NET_LOAD_BALANCE_API_H_ 00022 00023 #include "ns_types.h" 00024 00025 /** 00026 * \brief load_balance_network_switch_notify This function is called by the load balancer when it has detected a better network to switch to. 00027 * 00028 * \return true The network can be switched immediately. 00029 * \return false The load balancer will ask later a time for network switching if a better network is still available at that time. 00030 */ 00031 typedef bool net_load_balance_network_switch_notify(void); 00032 00033 /** 00034 * \brief Set user callback for accepting the network switch. 00035 * \param interface_id Interface ID. 00036 * \param network_switch_notify User callback. 00037 * 00038 * \return 0 Set OK. 00039 * \return -1 unknown Interface. 00040 */ 00041 int8_t net_load_balance_network_switch_cb_set(int8_t interface_id, net_load_balance_network_switch_notify *network_switch_notify); 00042 00043 /** 00044 * \brief Create and enable load balance to the selected interface. 00045 * \param interface_id Interface ID. 00046 * \param enable_periodic_beacon_interval Set True when you want to activate load balance periodic beacon; false will work only properly with the FHSS system. 00047 * 00048 * \return 0 Enable OK. 00049 * \return -1 unknown Interface or parameter error. 00050 * \return -2 Out of memory. 00051 * \return -3 Load balance already configured to this interface. 00052 */ 00053 int8_t net_load_balance_create(int8_t interface_id, bool enable_periodic_beacon_interval); 00054 00055 /** 00056 * \brief Disable and delete load balancing from the interface. 00057 * \param interface_id Interface ID. 00058 * 00059 * \return 0 Process OK. 00060 * \return -1 Unknown interface. 00061 */ 00062 int8_t net_load_balance_delete(int8_t interface_id); 00063 00064 /** 00065 * \brief Set load balance threshold min and max. 00066 * 00067 * Network switch: diff_priority >= randLIB_get_random_in_range(threshold_min, threshold_max) --> switch network if true. 00068 * For border router: Disable network compare by setting threshold_min and threshold_max to 0. 00069 * 00070 * \param interface_id Interface ID. 00071 * \param threshold_min Min value defines a random minimum value for compare (must be bigger than 0). 00072 * \param threshold_max Max value defines a random maximum value for compare (must be bigger than 0). 00073 * 00074 * \return 0 Process OK, -1 Unknown interface ID. 00075 */ 00076 int8_t net_load_balance_threshold_set(int8_t interface_id, uint8_t threshold_min, uint8_t threshold_max); 00077 00078 00079 /** 00080 * \brief Set the network probability percent when the new network is better than threshold max. 00081 * 00082 * \param interface_id Interface ID. 00083 * \param max_p Probability percent to switch the network. Default is 25%. Accepted values are [1,100], recommend values are 10-25. 00084 * 00085 * \return 0 Process OK, -1 Unknown interface ID or parameter fail. 00086 */ 00087 int8_t net_load_balance_set_max_probability(int8_t interface_id , uint8_t max_p); 00088 00089 /** 00090 * \brief Set load balance expected device count and enable automatic network load level update. 00091 * 00092 * This feature is just for RPL DoDAG root device! 00093 * 00094 * \param interface_id Interface ID. 00095 * \param expected_device_count Device count that sets the max load level. 00096 * - If the count is not divisible by 8, the function rounds the number up to reach that. 00097 * - It is not hard limit it define max DoDAG preference. 00098 * - For hard limit, check whiteboard_api.h. 00099 * 00100 * \return 0 Process OK, -1 Unknown interface ID, -2 Out of memory. 00101 */ 00102 int8_t net_load_balance_load_level_update_enable(int8_t interface_id, uint16_t expected_device_count); 00103 00104 /** 00105 * \brief Disable the automatic network load level update. 00106 * 00107 * \param interface_id Interface ID. 00108 * 00109 * \return 0 Process OK, -1 Unknown interface ID. 00110 */ 00111 int8_t net_load_balance_load_level_update_disable(int8_t interface_id); 00112 00113 00114 00115 #endif /* NET_LOAD_BALANCE_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
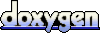