
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_interface.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 #ifndef NET_INTERFACE_H_ 00016 #define NET_INTERFACE_H_ 00017 00018 #include "ns_types.h" 00019 #include "platform/arm_hal_phy.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 struct mac_api_s; 00026 struct eth_mac_api_s; 00027 00028 /** 00029 * \file net_interface.h 00030 * \brief Network API 00031 */ 00032 00033 /** Network Interface Status */ 00034 typedef enum arm_nwk_interface_status_type_e { 00035 ARM_NWK_BOOTSTRAP_READY = 0, /**< Interface configured Bootstrap is ready.*/ 00036 ARM_NWK_RPL_INSTANCE_FLOODING_READY, /**< RPL instance has been flooded. */ 00037 ARM_NWK_SET_DOWN_COMPLETE, /**< Interface DOWN command completed successfully. */ 00038 ARM_NWK_NWK_SCAN_FAIL, /**< Interface has not detected any valid network. */ 00039 ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL, /**< IP address allocation failure (ND, DHCPv4 or DHCPv6). */ 00040 ARM_NWK_DUPLICATE_ADDRESS_DETECTED, /**< User-specific GP16 was not valid. */ 00041 ARM_NWK_AUHTENTICATION_START_FAIL, /**< No valid authentication server detected behind the access point. */ 00042 ARM_NWK_AUHTENTICATION_FAIL, /**< Network authentication failed by handshake. */ 00043 ARM_NWK_NWK_CONNECTION_DOWN, /**< No connection between access point and default router. */ 00044 ARM_NWK_NWK_PARENT_POLL_FAIL, /**< Sleepy host poll failed 3 times. Interface is shut down. */ 00045 ARM_NWK_PHY_CONNECTION_DOWN, /**< Interface PHY cable off or serial port interface not responding anymore. */ 00046 } arm_nwk_interface_status_type_e; 00047 00048 /** Event library type. */ 00049 typedef enum arm_library_event_type_e { 00050 ARM_LIB_TASKLET_INIT_EVENT = 0, /**< Tasklet init occurs always when generating a tasklet. */ 00051 ARM_LIB_NWK_INTERFACE_EVENT, /**< Interface bootstrap or state update event. */ 00052 ARM_LIB_SYSTEM_TIMER_EVENT, /*!*< System timer event. */ 00053 APPLICATION_EVENT, /**< Application-specific event. */ 00054 } arm_library_event_type_e; 00055 00056 00057 /* 00058 * Socket event description: 00059 * 00060 * 8-bit variable where four MSB bits describes the event type and 00061 * four LSB bits describes the socket that has received the event. 00062 * 00063 * Type Socket ID 00064 * ---- ---- 00065 * xxxx xxxx 00066 * 00067 */ 00068 00069 00070 /** \name Socket type exceptions. 00071 * @{ 00072 */ 00073 /** Socket event mask. */ 00074 #define SOCKET_EVENT_MASK 0xF0 00075 /** Data received. */ 00076 #define SOCKET_DATA (0 << 4) 00077 /** TCP connection ready. */ 00078 #define SOCKET_CONNECT_DONE (1 << 4) 00079 /** TCP connection failure. */ 00080 #define SOCKET_CONNECT_FAIL (2 << 4) 00081 /** TCP connection authentication failed. */ 00082 #define SOCKET_CONNECT_AUTH_FAIL (3 << 4) 00083 /** TCP incoming connection on listening socket */ 00084 #define SOCKET_INCOMING_CONNECTION (4 << 4) 00085 /** Socket data send failure. */ 00086 #define SOCKET_TX_FAIL (5 << 4) 00087 /** TCP connection closed (received their FIN and ACK of our FIN). */ 00088 #define SOCKET_CONNECT_CLOSED (6 << 4) 00089 /** TCP connection reset */ 00090 #define SOCKET_CONNECTION_RESET (7 << 4) 00091 /** No route available to the destination. */ 00092 #define SOCKET_NO_ROUTE (8 << 4) 00093 /** Socket TX done. */ 00094 #define SOCKET_TX_DONE (9 << 4) 00095 /** Out of memory failure. */ 00096 #define SOCKET_NO_RAM (10 << 4) 00097 /** TCP connection problem indication (RFC 1122 R1) */ 00098 #define SOCKET_CONNECTION_PROBLEM (11 << 4) 00099 00100 #define SOCKET_BIND_DONE SOCKET_CONNECT_DONE /**< Backward compatibility */ 00101 #define SOCKET_BIND_FAIL SOCKET_CONNECT_FAIL /**< Backward compatibility */ 00102 #define SOCKET_BIND_AUTH_FAIL SOCKET_CONNECT_AUTH_FAIL /**< Backward compatibility */ 00103 /* @} */ 00104 00105 /** Network security levels. */ 00106 typedef enum net_security_t { 00107 NW_NO_SECURITY = 0, /**< No Security. */ 00108 NW_SECURITY_LEVEL_MIC32 = 1, /**< 32-bit MIC verify, no encoding. */ 00109 NW_SECURITY_LEVEL_MIC64 = 2, /**< 64-bit MIC verify, no encoding. */ 00110 NW_SECURITY_LEVEL_MIC128 = 3, /**< 128-bit MIC verify, no encoding. */ 00111 NW_SECURITY_LEVEL_ENC = 4, /**< AES encoding without MIC. */ 00112 NW_SECURITY_LEVEL_ENC_MIC32 = 5, /**< 32-bit MIC verify with encoding. */ 00113 NW_SECURITY_LEVEL_ENC_MIC64 = 6, /**< 64-bit MIC verify with encoding. */ 00114 NW_SECURITY_LEVEL_ENC_MIC128 = 7 /**< 128-bit MIC verify with encoding. */ 00115 } net_security_t; 00116 00117 /** Ipv6 address type.*/ 00118 typedef enum net_address_t { 00119 ADDR_IPV6_GP, /**< Node default global address. */ 00120 ADDR_IPV6_GP_SEC, /**< Node secondary global address. */ 00121 ADDR_IPV6_LL /**< Node default link local address. */ 00122 } net_address_t; 00123 00124 /** MAC address type. */ 00125 typedef enum net_mac_address_t { 00126 ADDR_MAC_SHORT16, /**< Nodes 16-bit short address. */ 00127 ADDR_MAC_LONG64, /**< IP layer EUID64 based on MAC layer 64-bit long address after U/I -bit conversion. */ 00128 } net_mac_address_t; 00129 00130 /** TLS cipher type */ 00131 typedef enum { 00132 NET_TLS_PSK_CIPHER, /**< Network authentication support, only PSK. */ 00133 NET_TLS_ECC_CIPHER, /**< Network authentication support, only ECC. */ 00134 NET_TLS_PSK_AND_ECC_CIPHER, /**< Network authentication support, PSK & ECC. */ 00135 } net_tls_cipher_e; 00136 00137 /** PANA session type. */ 00138 typedef enum { 00139 NET_PANA_SINGLE_SESSION, /**< Client tracks only one PANA session data, default use case. */ 00140 NET_PANA_MULTI_SESSION, /**< Client supports many Start network coordinator session data */ 00141 } net_pana_session_mode_e; 00142 00143 /** 6LoWPAN network security & authentication modes. */ 00144 typedef enum { 00145 NET_SEC_MODE_NO_LINK_SECURITY, /**< Security disabled at link layer, DEFAULT. */ 00146 NET_SEC_MODE_PSK_LINK_SECURITY, /**< Link security by PSK key. */ 00147 NET_SEC_MODE_PANA_LINK_SECURITY, /**< PANA network authentication defined link KEY. */ 00148 } net_6lowpan_link_layer_sec_mode_e; 00149 00150 00151 /** Bootstrap modes */ 00152 typedef enum { 00153 NET_6LOWPAN_BORDER_ROUTER, /**< Root device for 6LoWPAN ND. */ 00154 NET_6LOWPAN_ROUTER, /**< Router device. */ 00155 NET_6LOWPAN_HOST, /**< Host device DEFAULT setting. */ 00156 NET_6LOWPAN_SLEEPY_HOST, /**< Sleepy host device. */ 00157 NET_6LOWPAN_NETWORK_DRIVER, /**< 6LoWPAN radio host device, no bootstrap. */ 00158 NET_6LOWPAN_SNIFFER /**< Sniffer device, no bootstrap. */ 00159 } net_6lowpan_mode_e; 00160 00161 /** 6LoWPAN Extension modes. */ 00162 typedef enum { 00163 NET_6LOWPAN_ND_WITHOUT_MLE, /**< **UNSUPPORTED** */ 00164 NET_6LOWPAN_ND_WITH_MLE, /**< 6LoWPAN ND with MLE. */ 00165 NET_6LOWPAN_THREAD, /**< 6LoWPAN Thread with MLE attached. */ 00166 NET_6LOWPAN_ZIGBEE_IP /**< **UNSUPPORTED** */ 00167 } net_6lowpan_mode_extension_e; 00168 00169 00170 /** IPv6 bootstrap modes */ 00171 typedef enum { 00172 NET_IPV6_BOOTSTRAP_STATIC, /**< Application defines the IPv6 prefix. */ 00173 NET_IPV6_BOOTSTRAP_AUTONOMOUS /**< Interface gets IPv6 address automatically from network using ICMP and DHCP. */ 00174 } net_ipv6_mode_e; 00175 00176 /** IPv6 accept RA behaviour */ 00177 typedef enum { 00178 NET_IPV6_RA_ACCEPT_IF_AUTONOMOUS, /**<Accept Router Advertisements when using autonomous IPv6 address allocation. Ignore when using a static address. This is the default value for the setting. */ 00179 NET_IPV6_RA_ACCEPT_ALWAYS /**<Accept Router Advertisements always, even when using static IPv6 address allocation. */ 00180 } net_ipv6_accept_ra_e; 00181 00182 /** Network coordinator parameter list. 00183 * Structure is used to read network parameter for warm start. 00184 */ 00185 typedef struct link_layer_setups_s { 00186 uint16_t PANId; /**< Network PAN-ID. */ 00187 uint8_t LogicalChannel; /**< Network logical channel. */ 00188 net_mac_address_t addr_mode; /**< Coordinator address mode. */ 00189 uint8_t address[8]; /**< Coordinator address. */ 00190 uint8_t sf; /**< Network superframe setup. */ 00191 } link_layer_setups_s; 00192 00193 /** Network MAC address info. */ 00194 typedef struct link_layer_address_s { 00195 uint16_t PANId; /**< Network PAN-ID. */ 00196 uint16_t mac_short; /**< MAC short address, if <0xfffe then is valid. */ 00197 uint8_t mac_long[8]; /**< MAC long address (EUI-48 for Ethernet; EUI-64 for IEEE 802.15.4). */ 00198 uint8_t iid_eui64[8]; /**< IPv6 interface identifier based on EUI-64. */ 00199 } link_layer_address_s; 00200 00201 /** Network layer parent address info. */ 00202 typedef struct network_layer_address_s { 00203 uint8_t border_router[16]; /**< ND Border Router Address. */ 00204 uint8_t prefix[8]; /**< Long 64-bit network ID. */ 00205 } network_layer_address_s; 00206 00207 /** Different addressing modes for a network interface. */ 00208 typedef enum { 00209 NET_6LOWPAN_GP64_ADDRESS, /**< Interface registers only GP64 address. */ 00210 NET_6LOWPAN_GP16_ADDRESS, /**< Interface registers only GP16 address. */ 00211 NET_6LOWPAN_MULTI_GP_ADDRESS, /**< Interface registers GP16 & GP64 addresses. */ 00212 } net_6lowpan_gp_address_mode_e; 00213 00214 /** TLS PSK info */ 00215 typedef struct net_tls_psk_info_s { 00216 uint32_t key_id; /**< PSK Key ID can be 0x01-0xffff, storage size is intentionally 32 bits. */ 00217 uint8_t key[16]; /**< 128-bit PSK Key. */ 00218 } net_tls_psk_info_s; 00219 00220 /** NETWORK PSK link key structure. */ 00221 typedef struct { 00222 uint8_t key_id; /**< Link layer PSK Key ID, can be 0x01-0xff. */ 00223 uint8_t security_key[16]; /**< Link layer 128-bit PSK Key. */ 00224 } net_link_layer_psk_security_info_s; 00225 00226 /** Certificate chain structure. */ 00227 typedef struct { 00228 uint8_t chain_length; /**< Certificate chain length, indicates the chain length. */ 00229 const uint8_t *cert_chain[4]; /**< Certificate chain pointer list. */ 00230 uint16_t cert_len[4]; /**< Certificate length. */ 00231 const uint8_t *key_chain[4]; /**< Certificate private key. */ 00232 } arm_certificate_chain_entry_s; 00233 00234 /** Structure for the network keys used by net_network_key_get */ 00235 typedef struct ns_keys_t 00236 00237 { 00238 uint8_t previous_active_network_key[16]; /**< The key that is currently active when a new key is generated and activated. */ 00239 uint8_t previous_active_key_index; /**< The index associated to the current_active_network_key. */ 00240 uint8_t current_active_network_key[16]; /**< Last generated and activated key. */ 00241 uint8_t current_active_key_index; /**< The index associated to the current_active_network_key. */ 00242 } ns_keys_t; 00243 00244 /** 6LoWPAN border router information structure. */ 00245 typedef struct { 00246 uint16_t mac_panid; /**< Link layer PAN-ID, accepts only < 0xfffe. */ 00247 uint16_t mac_short_adr; /**< Defines 802.15.4 short address. If the value is <0xfffe it indicates that GP16 is activated. */ 00248 uint8_t beacon_protocol_id; /**< ZigBeeIP uses always 2. */ 00249 uint8_t network_id[16]; /**< Network ID 16-bytes, will be used at beacon payload. */ 00250 uint8_t lowpan_nd_prefix[8]; /**< Define ND default prefix, ABRO, DODAG ID, GP address. */ 00251 uint16_t ra_life_time; /**< Define ND router lifetime in seconds, recommend value 180+. */ 00252 uint32_t abro_version_num; /**< ND ABRO version number (0 when starting a new ND setup). */ 00253 } border_router_setup_s; 00254 00255 /** Channel list */ 00256 typedef struct channel_list_s 00257 { 00258 channel_page_e channel_page; /**< Channel page */ 00259 uint32_t channel_mask[8]; /**< Channel mask. Each bit defining one channel */ 00260 } channel_list_s; 00261 00262 /** 6LoWPAN radio interface setup. */ 00263 typedef struct { 00264 uint16_t mac_panid; /**< Link layer PAN-ID, accepts only < 0xfffe. */ 00265 uint16_t mac_short_adr; /**< Defines 802.15.4 short address. If the value is <0xfffe it indicates that GP16 is activated. */ 00266 uint8_t beacon_protocol_id; /**< ZigBeeIP uses always 2. */ 00267 uint8_t network_id[16]; /**< Network ID 16-bytes, will be used at beacon payload. */ 00268 uint8_t beacon_payload_tlv_length; /**< Optional steering parameter length. */ 00269 uint8_t *beacon_payload_tlv_ptr; /**< Optional steering parameters. */ 00270 } network_driver_setup_s; 00271 00272 /** 00273 * Init 6LoWPAN library 00274 * 00275 * \return 0, Init OK. 00276 */ 00277 extern int8_t net_init_core(void); 00278 00279 /** 00280 * \brief Create network interface base to IDLE state. 00281 * \param api Generates interface with ethernet MAC. 00282 * \param interface_name_ptr String pointer to interface name. Need to end to '\0' character. 00283 * Max length 32 characters including NULL at end. Note: the given name is not copied, 00284 * so it must remain valid as long as the interface is. 00285 * 00286 * \return >=0 Interface ID (0-127). Application needs to save this information. 00287 * \return -1 api was NULL. 00288 * \return -2 Ethernet is not supported at this build. 00289 * \return -3 No memory for the interface. 00290 */ 00291 extern int8_t arm_nwk_interface_ethernet_init(struct eth_mac_api_s *api, const char *interface_name_ptr); 00292 00293 /** 00294 * \brief Create network interface base to IDLE state. 00295 * \param api Generates interface with 802.15.4 MAC. 00296 * \param interface_name_ptr String pointer to interface name. Need to end to '\0' character. 00297 * Max length 32 characters including NULL at end. Note: the given name is not copied, 00298 * so it must remain valid as long as the interface is. 00299 * 00300 * \return >=0 Interface ID (0-127). Application needs to save this information. 00301 * \return -1 api was NULL. 00302 * \return -3 No memory for the interface. 00303 */ 00304 extern int8_t arm_nwk_interface_lowpan_init(struct mac_api_s *api, char *interface_name_ptr); 00305 00306 /** 00307 * \brief Set IPv6 interface setup. 00308 * 00309 * \param interface_id Network interface ID. 00310 * \param bootstrap_mode Selected bootstrap mode: 00311 * * NET_IPV6_BOOTSTRAP_STATIC, Application defines the IPv6 prefix. 00312 * \param ipv6_prefix_pointer Pointer to 64 bit IPv6 prefix. The data is copied, so it can be invalidated after function call. 00313 * 00314 * \return >=0 Bootstrap mode set OK. 00315 * \return -1 Unknown network ID. 00316 */ 00317 extern int8_t arm_nwk_interface_configure_ipv6_bootstrap_set(int8_t interface_id, net_ipv6_mode_e bootstrap_mode, const uint8_t *ipv6_prefix_pointer); 00318 00319 /** 00320 * \brief Accept Router Advertisements setting. 00321 * 00322 * Accept Router Advertisements setting. Setting can be changed after an interface is created. 00323 * If setting is changed it must be done before the bootstrap is started. 00324 * 00325 * \param interface_id The network interface ID. 00326 * \param accept_ra Router Advertisements handling mode. 00327 * \return 0 Setting done. 00328 * \return <0 Failed (for example an invalid interface ID). 00329 */ 00330 extern int8_t arm_nwk_interface_accept_ipv6_ra(int8_t interface_id, net_ipv6_accept_ra_e accept_ra); 00331 00332 /** 00333 * \brief Set network interface bootstrap setup. 00334 * 00335 * \param interface_id Network interface ID. 00336 * \param bootstrap_mode Selected bootstrap mode: 00337 * * NET_6LOWPAN_BORDER_ROUTER, Initialize border router basic setup. 00338 * * NET_6LOWPAN_ROUTER, Enable normal 6LoWPAN ND and RPL to bootstrap. 00339 * * NET_6LOWPAN_HOST, Enable normal 6LoWPAN ND only to bootstrap. 00340 * * NET_6LOWPAN_SLEEPY_HOST, Enable normal 6LoWPAN ND only to bootstrap. 00341 * * NET_6LOWPAN_NETWORK_DRIVER, 6LoWPAN radio host device no bootstrap. 00342 * * NET_6LOWPAN_SNIFFER, 6LoWPAN sniffer device no bootstrap. 00343 * 00344 * \param net_6lowpan_mode_extension Define 6LoWPAN MLE and mode as ZigBeeIP or Thread. 00345 * 00346 * \return >=0 Bootstrap mode set OK. 00347 * \return -1 Unknown network ID. 00348 * \return -2 Unsupported bootstrap type in this library. 00349 * \return -3 No memory for 6LoWPAN stack. 00350 * \return -4 Null pointer parameter. 00351 */ 00352 extern int8_t arm_nwk_interface_configure_6lowpan_bootstrap_set(int8_t interface_id, net_6lowpan_mode_e bootstrap_mode, net_6lowpan_mode_extension_e net_6lowpan_mode_extension); 00353 00354 /** 00355 * \brief Set network interface link layer parameters. 00356 * 00357 * \param interface_id Network interface ID 00358 * \param nwk_channel_list Defines network channel page and channel. 00359 * \param link_setup Link layer parameters for NET_6LOWPAN_NETWORK_DRIVER defines NetworkID, PAN-ID Short Address. 00360 * 00361 * \return >=0 Configuration set OK. 00362 * \return -1 Unknown network ID. 00363 * \return -2 Interface is active, bootsrap mode not selected or is not NET_6LOWPAN_NETWORK_DRIVER or NET_6LOWPAN_SNIFFER. 00364 * \return -3 No memory for 6LoWPAN stack. 00365 * \return -4 Null pointer parameter. 00366 */ 00367 extern int8_t arm_nwk_interface_network_driver_set(int8_t interface_id, const channel_list_s *nwk_channel_list, network_driver_setup_s *link_setup); 00368 00369 /** 00370 * \brief Set configured network interface global address mode (border router bootstrap mode cannot set this). 00371 * 00372 * \param interface_id Network interface ID. 00373 * \param mode Define 6LoWPAN Global Address register mode: 00374 * * NET_6LOWPAN_GP64_ADDRESS, Interface registers only GP64 00375 * * NET_6LOWPAN_GP16_ADDRESS, Interface registers only GP16 00376 * * NET_6LOWPAN_MULTI_GP_ADDRESS, Interface registers GP16 and GP64 addresses. GP16 is primary address and GP64 is secondary. 00377 * 00378 * \param short_address_base Short address base. If the application defines value 0-0xfffd, 6LoWPAN tries to register GP16 address 00379 * using that address. 0xfffe and 0xffff generate random 16-bit short address. 00380 * 00381 * \param define_new_short_address_at_DAD This parameter is only checked when mode is not NET_6LOWPAN_GP64_ADDRESS and 00382 * short_address_base is 0-0xfffd. Recommended value is 1. It enables automatic new address definition at 00383 * Duplicate Address Detection (DAD). Value 0 generates a DAD error for the interface bootstrap. 00384 * Border router device will not check that part. 00385 * 00386 * \return >=0 Bootstrap mode set OK. 00387 * \return -1 Unknown network ID. 00388 * \return -2 Illegal for border router. 00389 * \return -3 No memory for 6LoWPAN stack. 00390 */ 00391 extern int8_t arm_nwk_6lowpan_gp_address_mode(int8_t interface_id, net_6lowpan_gp_address_mode_e mode, uint16_t short_address_base, uint8_t define_new_short_address_at_DAD); 00392 00393 /** 00394 * \brief Set the channel list configuration to be used on the network interface. 00395 * 00396 * \param interface_id Network interface ID. 00397 * \param nwk_channel_list Channel list to be used. 00398 * 00399 * \return >=0 Channel configuration OK. 00400 * \return -1 Unknown network interface ID. 00401 * \return -2 Empty channel list, no channels enabled. 00402 * \return -4 If network interface is already active and cannot be re-configured. 00403 */ 00404 extern int8_t arm_nwk_set_channel_list(int8_t interface_id, const channel_list_s *nwk_channel_list); 00405 00406 /** 00407 * \brief Set the link scan time used on network interface. 00408 * 00409 * \param interface_id Network interface ID. 00410 * \param scan_time Value 0-14, scan duration/channel. 00411 * 00412 * \return >=0 Scan configuration OK. 00413 * \return -1 Unknown network interface ID. 00414 * \return -4 If network interface is already active and cannot be re-configured. 00415 * \return -5 Invalid scan time. 00416 */ 00417 extern int8_t arm_nwk_6lowpan_link_scan_parameter_set(int8_t interface_id, uint8_t scan_time); 00418 00419 /** 00420 * \brief A function to set the PAN ID filter. 00421 * 00422 * \param interface_id Network interface ID. 00423 * \param pan_id_filter Enable filter for specific PAN ID. 0xffff disables the filter. 00424 * 00425 * \return 0 Filter set OK. 00426 * \return -1 Unknown Network interface ID. 00427 * \return -2 Interface is active. 00428 * 00429 */ 00430 extern int8_t arm_nwk_6lowpan_link_panid_filter_for_nwk_scan(int8_t interface_id, uint16_t pan_id_filter); 00431 00432 /** 00433 * \brief Get current used channel. 00434 * 00435 * \param interface_id Network interface ID. 00436 * 00437 * \return Active channel. 00438 * \return -1 = Radio is closed. 00439 */ 00440 extern int16_t arm_net_get_current_channel(int8_t interface_id); 00441 00442 /** 00443 * \brief A function to read the PAN ID filter. 00444 * 00445 * \param interface_id Network interface ID. 00446 * 00447 * \return 16-bit value indicating a PAN ID filter. 00448 */ 00449 extern uint16_t arm_net_get_nwk_pan_id_filter(int8_t interface_id); 00450 00451 /** 00452 * \brief Enable/Disable network ID filter. 00453 * 00454 * \param interface_id Network interface ID. 00455 * \param nwk_id_filter A pointer to a new network ID filter, NULL disable filter. 00456 * 00457 * \return 0 On success. 00458 * \return -1 Unknown network ID. 00459 * \return -2 Interface active. 00460 */ 00461 extern int8_t arm_nwk_6lowpan_link_nwk_id_filter_for_nwk_scan(int8_t interface_id, const uint8_t *nwk_id_filter); 00462 00463 /** 00464 * \brief Enable/Disable network protocol ID filter. 00465 * 00466 * \param interface_id Network interface ID. 00467 * \param protocol_ID A value that filters only supported network protocols (0= Zigbee1.x, 2= ZigBeeIP). 00468 * 00469 * \return 0 On success. 00470 * \return -1 Unknown network ID. 00471 * \return -2 Interface active. 00472 */ 00473 extern int8_t arm_nwk_6lowpan_link_protocol_id_filter_for_nwk_scan(int8_t interface_id, uint8_t protocol_ID); 00474 00475 /** 00476 * \brief Beacon join priority transmit callback. 00477 * 00478 * Callback defines join priority that is transmitted in beacon. Join priority is 00479 * 8 bit field in beacon that can be set e.g. based on RPL protocol rank data. 00480 * 00481 * \param interface_id The network interface ID. 00482 * 00483 * \return Join priority to be transmitted in beacon. 0 to 255. 00484 */ 00485 typedef uint8_t beacon_join_priority_tx_cb(int8_t interface_id); 00486 00487 /** 00488 * \brief Compare received beacon callback. 00489 * 00490 * Callback defines how preferred the node that has sent beacon is for connecting 00491 * to the network. 00492 * 00493 * \param interface_id The network interface ID. 00494 * \param join_priority Join priority that has been received in beacon. 0 to 255. 00495 * \param link_quality Link quality. 0 to 255. 255 is best quality. 00496 * 00497 * \return Connect to preference. 0 to 255. 255 is highest connect to preference. 00498 */ 00499 typedef uint8_t beacon_compare_rx_cb(int8_t interface_id, uint8_t join_priority, uint8_t link_quality); 00500 00501 /** 00502 * \brief Set callback for beacon join priority transmit 00503 * 00504 * Sets callback that defines join priority that is transmitted in beacon. 00505 * If callback is not set default functionality is used. On default functionality 00506 * join priority is combined from RPL DAGRank and RPL DODAG preference. 00507 * 00508 * \param interface_id The network interface ID. 00509 * \param beacon_join_priority_tx_cb_ptr Function pointer. 00510 * 00511 * \return 0 on success. 00512 * \return -1 Unknown network ID. 00513 * \return -2 Other error. 00514 */ 00515 extern int8_t arm_nwk_6lowpan_beacon_join_priority_tx_callback_set(int8_t interface_id, beacon_join_priority_tx_cb *beacon_join_priority_tx_cb_ptr); 00516 00517 /** 00518 * \brief Set callback for comparing received beacon 00519 * 00520 * Sets callback that defines how preferred the node that has sent beacon is for 00521 * connecting to the network. If callback is not set default functionality is used. 00522 * On default functionality connecting priority is defined based on join priority 00523 * received in beacon and link quality. 00524 * 00525 * \param interface_id Network interface ID. 00526 * \param beacon_compare_rx_cb_ptr Function pointer. 00527 * 00528 * \return 0 on success. 00529 * \return -1 Unknown network ID. 00530 * \return -2 Other error. 00531 */ 00532 extern int8_t arm_nwk_6lowpan_beacon_compare_rx_callback_set(int8_t interface_id, beacon_compare_rx_cb *beacon_compare_rx_cb_ptr); 00533 00534 /** 00535 * \brief Initialize and configure the interface security mode. 00536 * 00537 * \param interface_id Network interface ID. 00538 * \param mode Defines link layer security mode. 00539 * NET_SEC_MODE_NO_LINK_SECURITY, No security. 00540 * NET_SEC_MODE_PSK_LINK_SECURITY, Predefined PSK link layer key and ID. 00541 * NET_SEC_MODE_PANA_LINK_SECURITY, PANA bootstrap network authentication. 00542 * 00543 * \param sec_level Defined security level is checked only when the mode is not NET_SEC_MODE_NO_LINK_SECURITY. 00544 * \param psk_key_info Pointer for PSK link layer keys. Checked only when the mode is NET_SEC_MODE_PSK_LINK_SECURITY. 00545 * 00546 * \return 0 on success. 00547 */ 00548 00549 extern int8_t arm_nwk_link_layer_security_mode(int8_t interface_id, net_6lowpan_link_layer_sec_mode_e mode, uint8_t sec_level, const net_link_layer_psk_security_info_s *psk_key_info); 00550 00551 /** 00552 * \brief Initialize and configure interface PANA network client. 00553 * 00554 * \param interface_id Network interface ID. 00555 * \param cipher_mode Defines TLS 1.2 Cipher mode PSK, ECC or both. 00556 * \param psk_key_id PSK KEY id for PSK Setup 00557 * 00558 * \return 0 on success. 00559 * \return -1 Unknown network ID or PANA is not supported at this library. 00560 * \return -2 Interface active. 00561 */ 00562 extern int8_t arm_pana_client_library_init(int8_t interface_id, net_tls_cipher_e cipher_mode, uint32_t psk_key_id); 00563 00564 /** 00565 * \brief Initialize and Configure interface PANA network server. 00566 * 00567 * \param interface_id Network interface ID. 00568 * \param cipher_mode Defines TLS 1.2 Cipher mode PSK, ECC or both. 00569 * \param key_material A pointer to 128-bit key material or NULL when the PANA server generates the random key. 00570 * \param time_period_before_activate_key Guard period after a succesful key delivery phase before the key will be activated by server. 00571 * 00572 * \return 0 On success. 00573 * \return -1 Unknown network ID. 00574 * \return -2 Interface active. 00575 */ 00576 extern int8_t arm_pana_server_library_init(int8_t interface_id, net_tls_cipher_e cipher_mode, const uint8_t *key_material, uint32_t time_period_before_activate_key); 00577 00578 /** 00579 * \brief Manually initiate a PANA client key pull. For test purposes only. 00580 * 00581 * \param interface_id Network interface ID. 00582 * 00583 * \return 0 On success. 00584 * \return -1 Unknown network ID. 00585 */ 00586 extern int8_t arm_pana_client_key_pull(int8_t interface_id); 00587 00588 /** 00589 * \brief Trigger network key update process 00590 * 00591 * This function delivers a new network key to all ZigBee routers that have registered a GP address to server. 00592 * The function call always trig new key-id. Key delivery is started in 300ms interval between nodes. 00593 * This function does not cause any traffic if the server does not have any router device sessions. 00594 * 00595 * \param interface_id Network interface ID. 00596 * \param network_key_material Pointer to new 128-bit key material, NULL generates a random key. 00597 * 00598 * \return 0 Key Update process OK. 00599 * \return -1 PANA server is not initialized yet. 00600 * \return -2 Old key update still active. 00601 * \return -3 Memory allocation fails. 00602 */ 00603 extern int8_t arm_pana_server_key_update(int8_t interface_id, const uint8_t *network_key_material); 00604 00605 /** 00606 * \brief Activate new key material before standard timeout. 00607 * 00608 * This function is only for testing. 00609 * 00610 * \param interface_id Network interface ID. 00611 * 00612 * \return 0 Key activate process OK. 00613 * \return -1 No Pending key update. 00614 * \return -2 PANA server is not initialized or PANA server API is disabled with this library. 00615 */ 00616 extern int8_t arm_pana_activate_new_key(int8_t interface_id); 00617 00618 /** 00619 * \brief Read PANA server security key material. 00620 * 00621 * previous_active_network_key Only valid when current_active_key_index is bigger than 1. 00622 * 00623 * \param interface_id Interface 00624 * \param key Pointer for key material information store. 00625 * 00626 * \return 0 Key read OK. 00627 * \return -1 PANA server key material not available. 00628 */ 00629 extern int8_t arm_network_key_get(int8_t interface_id, ns_keys_t *key); 00630 00631 /** 00632 * \brief Start network interface bootstrap. 00633 * 00634 * \param interface_id Network interface ID. 00635 * 00636 * 00637 * \return >=0 Bootstrap start OK. 00638 * \return -1 Unknown network ID. 00639 * \return -2 Not configured. 00640 * \return -3 Active. 00641 */ 00642 extern int8_t arm_nwk_interface_up(int8_t interface_id); 00643 00644 /** 00645 * \brief Stop and set interface to idle. 00646 * 00647 * \param interface_id Network interface ID 00648 * 00649 * \return >=0 Process OK. 00650 * \return -1 Unknown network ID. 00651 * \return -3 Not Active. 00652 */ 00653 extern int8_t arm_nwk_interface_down(int8_t interface_id); 00654 00655 /** 00656 * \brief Define border router MAC and 6LoWPAN ND setup for selected interface. 00657 * 00658 * \param interface_id Network interface ID. 00659 * \param border_router_setup_ptr Pointer to MAC and 6LoWPAN ND setup. 00660 * 00661 * \return 0 on success, negative value on error case. 00662 */ 00663 extern int8_t arm_nwk_6lowpan_border_router_init(int8_t interface_id, const border_router_setup_s *border_router_setup_ptr); 00664 00665 /** 00666 * \brief Add context at 6LoWPAN interface configure state. 00667 * 00668 * \param interface_id Network interface ID. 00669 * \param c_id_flags Bit 4 indicates compress support and bit 0-3 context ID. 00670 * \param context_len Context length in bits need to be 64-128. 00671 * \param ttl Context time to live, value in minutes. 00672 * \param context_ptr Pointer to full 128-bit memory area. 00673 * 00674 * \return 0 Context update OK. 00675 * \return -1 No memory for new context. 00676 * \return -2 Border router base not allocated. 00677 * \return -3 Given parameter fails (c_id_flags > 0x1f or contex_len < 64). 00678 * \ 00679 */ 00680 extern int8_t arm_nwk_6lowpan_border_router_context_update(int8_t interface_id, uint8_t c_id_flags, uint8_t context_len, uint16_t ttl, const uint8_t *context_ptr); 00681 00682 /** 00683 * \brief Update runtime configured context. 00684 * 00685 * This function can change the value of context compress state or time to live. 00686 * It triggers a new ABRO version number, indicating that ND parameters are updated. 00687 * 00688 * \param interface_id Network interface ID. 00689 * \param c_id Context ID stack checks first 4 bits, supported values 0-15. 00690 * \param compress_mode 0 = Compress disabled, otherwise compress enabled. 00691 * \param ttl Context time to live value in minutes. 00692 * 00693 * \return 0 Update OK. 00694 * \return -1 Update fail by router state. 00695 * 00696 */ 00697 extern int8_t arm_nwk_6lowpan_border_router_context_parameter_update(int8_t interface_id, uint8_t c_id, uint8_t compress_mode, uint16_t ttl); 00698 00699 /** 00700 * \brief Delete allocated context by ID. 00701 * 00702 * \param interface_id Network interface ID. 00703 * \param c_id 4-bit Context ID to be deleted. 00704 * 00705 * \return 0 Context delete OK. 00706 * \return -1 Delete process fails. 00707 */ 00708 extern int8_t arm_nwk_6lowpan_border_router_context_remove_by_id(int8_t interface_id, uint8_t c_id); 00709 00710 /** 00711 * \brief Update ND ABRO version number. 00712 * 00713 * \param interface_id Network interface ID 00714 * 00715 * \return 0 ABRO version update OK. 00716 * \return -1 ABRO update fails (Interface is not up yet or the border router base is not allocated). 00717 */ 00718 extern int8_t arm_nwk_6lowpan_border_router_configure_push(int8_t interface_id); 00719 00720 /** 00721 * Set timeout for default prefix on cache. 00722 * Requires arm_nwk_6lowpan_border_router_configure_push() be called to settings be taken into use. 00723 * \param interface_id mesh interface. 00724 * \param time seconds 00725 * \return 0 on success, negative value on failure. 00726 */ 00727 extern int8_t arm_nwk_6lowpan_border_route_nd_default_prefix_timeout_set(int8_t interface_id, uint32_t time); 00728 00729 /** 00730 * \brief A function to read network layer configurations. 00731 * \param interface_id Network interface ID. 00732 * \param network_params A pointer to the structure where the network layer configs are written. 00733 * \return 0 On success. 00734 * \return Negative value if interface is not known. 00735 */ 00736 int8_t arm_nwk_param_read(int8_t interface_id, link_layer_setups_s *network_params); 00737 00738 /** 00739 * \brief A function to read MAC PAN-ID, Short address and EUID64. 00740 * \param interface_id Network interface ID. 00741 * \param mac_params A pointer to the structure where the MAC addresses are written. 00742 * \return 0 On success. 00743 * \return Negative value if interface is not known. 00744 */ 00745 int8_t arm_nwk_mac_address_read(int8_t interface_id, link_layer_address_s *mac_params); 00746 00747 /** 00748 * \brief A function to read 6LoWPAN ND border router address and NWK prefix. 00749 * \param interface_id Network interface ID. 00750 * \param nd_addr_info Pointer to the structure where the address is written. 00751 * \return 0 On success. 00752 * \return Negative value if network interface is not known or if the interface 00753 * is not in active or ready state. 00754 */ 00755 int8_t arm_nwk_nd_address_read(int8_t interface_id, network_layer_address_s *nd_addr_info); 00756 00757 /** 00758 * \brief A function to read the networking address information. 00759 * \param interface_id Network interface ID. 00760 * \param addr_id The address information type to be read. 00761 * \param address A pointer to a structure where the address information is written. 00762 * \return 0 On success, -1 on failure. 00763 */ 00764 extern int8_t arm_net_address_get(int8_t interface_id, net_address_t addr_id, uint8_t *address); 00765 00766 /** 00767 * \brief A function to read networking addresses one by one. 00768 * \param interface_id Network interface ID. 00769 * \param n A pointer that is incremented every call. Start looping with n=0. 00770 * \param address_buffer A pointer to buffer where address is copied. 00771 * \return 0 On success. 00772 * \return -1 No more addresses available. 00773 */ 00774 extern int8_t arm_net_address_list_get_next(int8_t interface_id, int *n, uint8_t address_buffer[16]); 00775 00776 /** 00777 * \brief A function to read network interface address count. 00778 * \param interface_id Network interface ID. 00779 * \param address_count A pointer to the structure where the address count is saved. 00780 * 00781 * \return 0 On success, -1 on errors. 00782 */ 00783 extern int8_t arm_net_interface_address_list_size(int8_t interface_id, uint16_t *address_count); 00784 00785 /** 00786 * \brief A function to set interface metric. 00787 * \param interface_id Network interface ID. 00788 * \param metric Used to rank otherwise-equivalent routes. Lower is preferred and default is 0. The metric value is added to metric provided by the arm_net_route_add() function. 00789 * 00790 * \return 0 On success, -1 on errors. 00791 */ 00792 extern int8_t arm_net_interface_set_metric(int8_t interface_id, uint16_t metric); 00793 00794 /** 00795 * \brief A function to read the interface metric value on an interface. 00796 * \param interface_id Network interface ID. 00797 * \param metric A pointer to the variable where the interface metric value is saved. 00798 * 00799 * \return 0 On success, -1 on errors. 00800 */ 00801 extern int8_t arm_net_interface_get_metric(int8_t interface_id, uint16_t *metric); 00802 00803 /** 00804 * \brief A function to read the network interface. 00805 * \param interface_id Network interface ID. 00806 * \param address_buf_size Buffer size in bytes, minimum 16 bytes. 00807 * \param address_buffer A pointer to a structure where the addresses are saved one by one. 00808 * \param writed_address_count A pointer to the structure where the number of addresses saved is written. 00809 * 00810 * \return 0 on success, -1 on errors. 00811 */ 00812 extern int8_t arm_net_address_list_get(int8_t interface_id, uint8_t address_buf_size, uint8_t *address_buffer, int *writed_address_count); 00813 00814 /** 00815 * \brief A function to add an address to an interface. 00816 * \param interface_id Network interface ID. 00817 * \param address The address to be added to the interface. 00818 * \param prefix_len The length of the address prefix. 00819 * \param valid_lifetime The time in seconds until the address becomes invalid and is removed from the interface. Value 0xffffffff represents infinity. 00820 * \param preferred_lifetime The time in seconds until the address becomes deprecated. Value 0xffffffff represents infinity. The preferred lifetime should not be longer than a valid lifetime. 00821 * \return 0 on success, -1 on errors. 00822 */ 00823 extern int8_t arm_net_address_add_to_interface(int8_t interface_id, const uint8_t address[16], uint8_t prefix_len, uint32_t valid_lifetime, uint32_t preferred_lifetime); 00824 00825 /** 00826 * \brief A function to remove an address from an interface. 00827 * \param interface_id Network interface ID. 00828 * \param address The address to be removed from the interface. 00829 * 00830 * \return 0 on success, -1 on errors. 00831 */ 00832 extern int8_t arm_net_address_delete_from_interface(int8_t interface_id, const uint8_t address[16]); 00833 00834 /** 00835 * \brief A function to add a route to the routing table. 00836 * \param prefix Destination prefix for the route to be added. 00837 * \param prefix_len The length of the prefix. 00838 * \param next_hop Link-local address of the next hop (e.g. router); if NULL the route is marked as on-link. 00839 * \param lifetime The time in seconds until the route is removed from the routing table. Value 0xffffffff means infinite. 00840 * \param metric Used to rank otherwise-equivalent routes. Lower is preferred. Normally 128. 00841 * \param interface_id Network interface ID. 00842 * \return 0 on success, -1 on add failure, -2 on invalid function parameters. 00843 */ 00844 extern int8_t arm_net_route_add(const uint8_t *prefix, uint8_t prefix_len, const uint8_t *next_hop, uint32_t lifetime, uint8_t metric, int8_t interface_id); 00845 00846 /** 00847 * \brief A function to remove a route from the routing table. 00848 * \param prefix The prefix to be removed. 00849 * \param prefix_len The length of the prefix. 00850 * \param next_hop Link-local address of the next hop. 00851 * \param interface_id Network interface ID. 00852 * \return 0 on success, -1 on delete failure, -2 on invalid function parameters. 00853 */ 00854 extern int8_t arm_net_route_delete(const uint8_t *prefix, uint8_t prefix_len, const uint8_t *next_hop, int8_t interface_id); 00855 00856 /** Border Router ND NVM update types. */ 00857 /** ND context update, 20 bytes data behind pointer. */ 00858 #define ND_PROXY_CONTEXT_NVM_UPDATE 0 00859 /** ND context update flags update. */ 00860 #define ND_PROXY_CONTEXT_FLAGS_NVM_UPDATE 1 00861 /** ND context remove. */ 00862 #define ND_PROXY_CONTEXT_NVM_REMOVE 2 00863 /** ND prefix update. */ 00864 #define ND_PROXY_PREFIX_NVM_UPDATE 3 00865 /** ND ABRO version update. */ 00866 #define ND_PROXY_ABRO_VERSION_NVM_UPDATE 4 00867 00868 /** 00869 * \brief Load context from NVM at ZigBeeIP interface configure state. 00870 * 00871 * \param interface_id Network Interface ID 00872 * \param contex_data A pointer to properly built 20 bytes update array. 00873 * 00874 * \return 0 Context reload OK. 00875 * \return <0 Load fail. 00876 */ 00877 extern int8_t arm_nwk_6lowpan_border_router_nd_context_load(int8_t interface_id, uint8_t *contex_data); //NVM 00878 00879 /** 00880 * Set certificate chain for PANA 00881 * \param chain_info Certificate chain. 00882 * \return 0 on success, negative on failure. 00883 */ 00884 extern int8_t arm_network_certificate_chain_set(const arm_certificate_chain_entry_s *chain_info); 00885 00886 /** 00887 * \brief Add PSK key to TLS library. 00888 * 00889 * \param key_ptr A pointer to 16 bytes long key array. 00890 * \param key_id PSK key ID. 00891 * 00892 * \return 0 = success 00893 * \return -1 = failure 00894 */ 00895 extern int8_t arm_tls_add_psk_key(const uint8_t *key_ptr, uint16_t key_id); 00896 00897 /** 00898 * \brief Remove PSK key from TLS library. 00899 * 00900 * \param key_id PSK key ID. 00901 * 00902 * \return 0 = success 00903 * \return -1 = failure 00904 */ 00905 extern int8_t arm_tls_remove_psk_key(uint16_t key_id); 00906 00907 /** 00908 * \brief Check if PSK key ID exists. 00909 * 00910 * \param key_id PSK key ID 00911 * 00912 * \return 0 = success 00913 * \return -1 = failure 00914 */ 00915 extern int8_t arm_tls_check_key(uint16_t key_id); 00916 00917 /** 00918 * \brief Print routing table 00919 * 00920 * Prints the routing table to the command line 00921 */ 00922 void arm_print_routing_table(void); 00923 00924 /** 00925 * \brief Print routing table 00926 * 00927 * Outputs the routing table using the given printf style function 00928 * 00929 * \param print_fn pointer to a printf style output function 00930 */ 00931 void arm_print_routing_table2(void (*print_fn)(const char *fmt, ...)); 00932 00933 /** 00934 * \brief Flush neighbor cache 00935 * 00936 * Flushes the neighbor cache 00937 */ 00938 void arm_ncache_flush(void); 00939 00940 /** 00941 * \brief Print neighbor cache 00942 * 00943 * Prints neighbor cache to the command line 00944 */ 00945 void arm_print_neigh_cache(void); 00946 00947 /** 00948 * \brief Print neighbor cache 00949 * 00950 * Outputs the neighbor cache using the given printf style function 00951 * 00952 * \param print_fn pointer to a printf style output function 00953 */ 00954 void arm_print_neigh_cache2(void (*print_fn)(const char *fmt, ...)); 00955 00956 /** 00957 * \brief Print PCB list 00958 * 00959 * Prints Protocol Control Block list to the command line 00960 */ 00961 void arm_print_protocols(void); 00962 00963 /** 00964 * \brief Print PCB list 00965 * 00966 * Prints Protocol Control Block list using the given printf style function 00967 * 00968 * \param print_fn pointer to a printf style output function 00969 * \param sep column separator character 00970 */ 00971 void arm_print_protocols2(void (*print_fn)(const char *fmt, ...), char sep); 00972 00973 /** 00974 * \brief Get the library version information. 00975 * 00976 * \param *ptr Pointer to data location. Required size is 20 bytes. 00977 * 00978 * 00979 * The array containing the version information has the following structure. 00980 * 00981 * | Platform type | Version | Build ID | 00982 * | :-----------: | :----------------: | 00983 * | 1 byte | 1 byte | 4 bytes | 00984 * 00985 */ 00986 extern void net_get_version_information(uint8_t *ptr); 00987 #ifdef __cplusplus 00988 } 00989 #endif 00990 #endif /* NET_INTERFACE_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
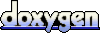