
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_6lowpan_parameter_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 00016 #ifndef NET_6LOWPAN_PARAMETER_API_H_ 00017 #define NET_6LOWPAN_PARAMETER_API_H_ 00018 00019 #include "ns_types.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 /** 00026 * \file net_6lowpan_parameter_api.h 00027 * \brief API for setting up 6LoWPAN network parameters 00028 * 00029 * \section tim-para-def 6LoWPAN Default timer values 00030 * - Timer values are specified in ticks 00031 * - Default 6LoWPAN ND Bootstrap tick is 1 tick = 100ms 00032 * 00033 * - Default Random value 0x1f = [0..3.1] seconds. 00034 * - Default RS retry counter 3. 00035 * - Default RS retry interval is 15 + random --> [1.5..4.6] seconds. 00036 * - Default NS retry counter 5. 00037 * - Default NS retry interval is 100 + random + backoff --> [10.0..13.1+10.0*retry] seconds. 00038 * - Default NS retry linear backoff is 100. 00039 * 00040 * - Default RA transmit interval is 150, exponentially backed off --> 15.0, 30.0, 60.0 seconds. 00041 * - Default RA transmit counter is 5. 00042 * 00043 * - Default NS forward timeout is 300 --> 30.0 seconds. 00044 * 00045 * \section Changing random and interval values 00046 * - Random parameter + NS or RS minimum interval must sum to less than 0xFFFF. 00047 * - Random maximums are manipulated as bit masks, so must be (2^n)-1. 00048 */ 00049 00050 /*! 00051 * \struct nd_parameters_s 00052 * \brief 6LoWPAN Neighbor Discovery parameters 00053 */ 00054 typedef struct nd_parameters_s { 00055 uint8_t rs_retry_max; /**< Define Bootstrap RS max retry count. */ 00056 uint8_t ns_retry_max; /**< Define Bootstrap NS max retry count. */ 00057 uint16_t timer_random_max; /**< Define Interval random in 6LoWPAN bootstrap timer ticks for RS, NS and starting NS - NA process. */ 00058 uint16_t rs_retry_interval_min; /**< Define Retry interval in 6LoWPAN bootstrap timer ticks waiting for RA. */ 00059 uint16_t ns_retry_interval_min; /**< Define Retry interval in 6LoWPAN bootstrap timer ticks waiting for NA. */ 00060 uint16_t ns_retry_linear_backoff; /**< Define Retry interval linear backoff in bootstrap timer ticks. */ 00061 bool multihop_dad; /**< Define whether to perform duplicate address detection with border router or locally. */ 00062 bool iids_map_to_mac; /**< Define whether IPv6 IIDs can be assumed to be based on MAC address (so no address resolution by routers). */ 00063 bool send_nud_probes; /**< Define whether IPv6 NUD probes are enabled (disabling may limit fault detection). */ 00064 uint16_t ra_interval_min; /**< Define initial transmission interval for Router Advertisements in standard timer ticks. */ 00065 uint8_t ra_transmits; /**< Define number of RA transmissions. */ 00066 uint8_t ra_cur_hop_limit; /**< Define the value of current hop limit in RAs. */ 00067 uint32_t ra_link_mtu; /**< Define the value of link MTU in RAs. */ 00068 uint32_t ra_reachable_time; /**< Define the value of reachable time in RAs (in milliseconds). */ 00069 uint32_t ra_retrans_timer; /**< Define the value of retrans timer in RAs (in milliseconds). */ 00070 uint16_t ns_forward_timeout; /**< Define timeout when forwarding NS messages - if reached, our own address discovery process is restarted. */ 00071 } nd_parameters_s; 00072 00073 /** 00074 * \brief Function to change 6LoWPAN ND bootstrap parameters. 00075 * 00076 * Note: This function should be called after net_init_core() and definitely 00077 * before creating any 6LoWPAN interface. 00078 * 00079 * For future compatibility, to support extensions to this structure, read 00080 * the current parameters using net_6lowpan_timer_parameter_read(), 00081 * modify known fields, then set. 00082 * 00083 * \param parameter_ptr Pointer for ND parameters. 00084 * 00085 * \return 0, Change OK. 00086 * \return -1, Invalid values. 00087 * \return -2, 6LoWPAN interface already active. 00088 * 00089 */ 00090 extern int8_t net_6lowpan_nd_parameter_set(const nd_parameters_s *parameter_ptr); 00091 00092 /** 00093 * \brief Function to change 6LoWPAN bootstrap base tick 100ms multiplier. 00094 * 00095 * Note: This function MUST be called after net_init_core(). Do not change this 00096 * unless you really want 6LoWPAN bootstrap working slower than normally. 00097 * 00098 * This only affects the bootstrap timers. 00099 * 00100 * \param base_tick_x_100ms Tick resolution in 100ms units. 00101 * Max value 10 --> 10 times slower functionality 00102 * 00103 * \return 0, Change OK. 00104 * \return -1, Invalid value (<1 or >10). 00105 * 00106 */ 00107 extern int8_t net_6lowpan_nd_timer_base_tick_set(uint8_t base_tick_x_100ms); 00108 00109 /** 00110 * \brief Function to read 6LoWPAN ND bootstrap parameters. 00111 * 00112 * \param parameter_ptr Output pointer for ND parameters. 00113 * 00114 */ 00115 extern void net_6lowpan_nd_parameter_read(nd_parameters_s *parameter_ptr); 00116 00117 #ifdef __cplusplus 00118 } 00119 #endif 00120 00121 #endif /* NET_6LOWPAN_DEFAULT_PARAMETER_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
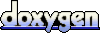