
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nsdynmemLIB_stub.c
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "nsdynmemLIB_stub.h" 00005 #include <stdint.h> 00006 #include <string.h> 00007 #include <nsdynmemLIB.h> 00008 #include "platform/arm_hal_interrupt.h" 00009 #include <stdlib.h> 00010 00011 nsdynmemlib_stub_data_t nsdynmemlib_stub; 00012 00013 #if NSDYNMEMLIB_API_VERSION < 2 00014 typedef int16_t ns_mem_block_size_t; 00015 typedef int16_t ns_mem_heap_size_t; 00016 void ns_dyn_mem_init(uint8_t *heap, uint16_t h_size, void (*passed_fptr)(heap_fail_t), mem_stat_t *info_ptr) 00017 { 00018 } 00019 #else 00020 void ns_dyn_mem_init(void *heap, uint16_t h_size, void (*passed_fptr)(heap_fail_t), mem_stat_t *info_ptr) 00021 { 00022 } 00023 #endif 00024 00025 void *ns_dyn_mem_alloc(ns_mem_block_size_t alloc_size) 00026 { 00027 if (nsdynmemlib_stub.returnCounter > 0) 00028 { 00029 nsdynmemlib_stub.returnCounter--; 00030 return malloc(alloc_size); 00031 } 00032 else 00033 { 00034 return(nsdynmemlib_stub.expectedPointer); 00035 } 00036 } 00037 00038 void *ns_dyn_mem_temporary_alloc(ns_mem_block_size_t alloc_size) 00039 { 00040 if (nsdynmemlib_stub.returnCounter > 0) 00041 { 00042 nsdynmemlib_stub.returnCounter--; 00043 return malloc(alloc_size); 00044 } 00045 else 00046 { 00047 return(nsdynmemlib_stub.expectedPointer); 00048 } 00049 } 00050 00051 void ns_dyn_mem_free(void *block) 00052 { 00053 free(block); 00054 }
Generated on Sun Jul 17 2022 08:25:29 by
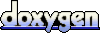