
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
multicast_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2012-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 #ifndef MULTICAST_API_H_ 00015 #define MULTICAST_API_H_ 00016 /** 00017 * \file multicast_api.h 00018 * \brief Multicast Trickle Forwarding API. 00019 * \section multi-init Init API: 00020 * - multicast_set_parameters(), Set trickle parameters. 00021 * \section multi-cnf Configure API: 00022 * - multicast_add_address(), Add new address to a multicast group and control trickle forwarding. 00023 * - multicast_free_address(), Remove supported multicast address from list. 00024 * 00025 * \section ZigBeeIP Trickle Setups for Multicast Init 00026 * 00027 * | Parameter | VALUE | 00028 * | :---------------: | :---: | 00029 * | imin | 10 | 00030 * | imax | 0 | 00031 * | k | 20 | 00032 * | timer_expirations | 3 | 00033 * | window_expiration | 75 | 00034 * 00035 */ 00036 00037 #include "ns_types.h" 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /** 00044 * \brief Set new parameters for trickle multicast. 00045 * \deprecated 00046 * 00047 * \param i_min Minimum trickle timer interval in 50ms resolution: Imin = i_min * 50ms. 00048 * \param i_doublings Maximum trickle timer interval expressed as number of doublings of the minimum interval. 00049 * \param k Redundancy constant. 00050 * \param timer_expirations Number of trickle timer expirations before terminating the trickle process. 00051 * \param window_expiration The time window for keeping the state after the end of trickle process in 50ms resolution. 00052 * NOTE: If window_expiration value is set too small an infinite retransmission loop may occur when using the trickle multicast. 00053 */ 00054 void multicast_set_parameters(uint8_t i_min, uint8_t i_doublings, uint8_t k, uint8_t timer_expirations, uint16_t window_expiration); 00055 00056 00057 /** 00058 * \brief Add new address to multicast group. 00059 * 00060 * \deprecated Use setsockopt(SOCKET_IPV6_JOIN_GROUP) to join a group. Use 00061 * multicast_mpl_subscribe_domain() to control MPL behaviour. 00062 * 00063 * \param address_ptr Pointer to a 16-byte array that includes the address to be added. 00064 * \param use_trickle 0 = no trickle multicast forwarding, all other values = trickle multicast forwarding will be used with this address. 00065 * 00066 * \return 0 General error. 00067 * \return 1 Address updated. 00068 * \return 2 Address added. 00069 * \return 255 Link local not allowed when using multicast. 00070 * 00071 */ 00072 uint8_t multicast_add_address(const uint8_t *address_ptr, uint8_t use_trickle); 00073 00074 00075 00076 /** 00077 * \brief Free address from multicast group. 00078 * \deprecated Use setsockopt(SOCKET_IPV6_LEAVE_GROUP) 00079 * 00080 * \param address_ptr Pointer to a 16-byte array that includes the address to be removed. 00081 * 00082 * \return 0 will be returned on successful execution, other values indicate an error on removing the address. 00083 */ 00084 uint8_t multicast_free_address(const uint8_t *address_ptr); 00085 00086 /** 00087 * \brief Treat all Realm-local Domains as one 00088 * 00089 * If enabled on an interface, then all Realm-local scope multicasts will 00090 * be treated as if belonging to the All-MPL-Forwarders domain, rather than 00091 * the domain indicated by their destination address. This is non-standard 00092 * behaviour, not covered by the real MPL specification, but required by the 00093 * Thread specification. 00094 * 00095 * All devices in a realm should have this setting set the same. With the 00096 * setting on, reactive forwarding and control messages will not work. 00097 * 00098 * With this setting on, Realm-scope domains other than All-MPL-Forwarders 00099 * cannot be joined. 00100 * 00101 * This must be set before bringing up an interface, and then not be modified. 00102 * 00103 * \param interface_id interface id 00104 * \param enable true to enable domain unification 00105 * 00106 * \return 0 for success, negative on failure 00107 */ 00108 int_fast8_t multicast_mpl_treat_realm_domains_as_one(int8_t interface_id, bool enable); 00109 00110 /** 00111 * \brief Set default MPL Domain parameters 00112 * 00113 * Modifies the default parameters for MPL Domains using the specified 00114 * interface. 00115 * 00116 * This must be set before subscribing to any MPL Domains with default 00117 * parameters on that interface. 00118 * 00119 * Initial defaults are as specified by RFC 7731. 00120 * 00121 * \param interface_id interface id 00122 * \param proactive_forwarding true to forward Data Messages when first received (default true) 00123 * \param seed_set_entry_lifetime minimum seed set lifetime (seconds, default 1800 = 30 minutes) 00124 * \param data_message_imin minimum Trickle timer for Data Messages (ms, default ~= 10 * expected link latency) 00125 * \param data_message_imax maximum Trickle timer for Data Messages (ms, default = Imin) 00126 * \param data_message_k Trickle redundancy constant for Data Messages (default = 1) 00127 * \param data_message_timer_expirations controls termination of retransmissions (default = 3) 00128 * \param control_message_imin minimum Trickle timer for Control Messages (ms, default ~= 10 * worst-case link latency) 00129 * \param control_message_imax maximum Trickle timer for Control Messages (ms, default = 5 minutes) 00130 * \param control_message_k Trickle redundancy constant for Control Messages (default = 1) 00131 * \param control_message_timer_expirations controls termination of retransmissions (default = 10); 0 disables control messages 00132 * 00133 * \return 0 for success, negative on failure 00134 */ 00135 int_fast8_t multicast_mpl_set_default_parameters(int8_t interface_id, 00136 bool proactive_forwarding, 00137 uint16_t seed_set_entry_lifetime, 00138 uint32_t data_message_imin, 00139 uint32_t data_message_imax, 00140 uint8_t data_message_k, 00141 uint8_t data_message_timer_expirations, 00142 uint32_t control_message_imin, 00143 uint32_t control_message_imax, 00144 uint8_t control_message_k, 00145 uint8_t control_message_timer_expirations); 00146 00147 /** Control selection of MPL Seed Identifier for packets we originate */ 00148 typedef enum { 00149 MULTICAST_MPL_SEED_ID_DEFAULT = -256, /** Default selection (used to make a domain use the interface's default) */ 00150 MULTICAST_MPL_SEED_ID_MAC_SHORT = -1, /** Use short MAC address if available (eg IEEE 802.15.4 interface's macShortAddress (16-bit)), else full MAC */ 00151 MULTICAST_MPL_SEED_ID_MAC = -2, /** Use MAC padded to 64-bit (eg IEEE 802.15.4 interface's macExtendedAddress, or 48-bit Ethernet MAC followed by 2 zero pad bytes) */ 00152 MULTICAST_MPL_SEED_ID_IID_EUI64 = -3, /** Use 64-bit IPv6 IID based on EUI-64 (eg 02:11:22:ff:fe:00:00:00 for an Ethernet interface with MAC 00:11:22:00:00:00) */ 00153 MULTICAST_MPL_SEED_ID_IID_SLAAC = -4, /** Use 64-bit IPv6 IID that would be used for SLAAC */ 00154 MULTICAST_MPL_SEED_ID_IPV6_SRC_FOR_DOMAIN = 0, /** Use IPv6 source address selection to choose 128-bit Seed ID based on MPL Domain Address as destination */ 00155 MULTICAST_MPL_SEED_ID_16_BIT = 2, /** Use a manually-specified 16-bit ID */ 00156 MULTICAST_MPL_SEED_ID_64_BIT = 8, /** Use a manually-specified 64-bit ID */ 00157 MULTICAST_MPL_SEED_ID_128_BIT = 16, /** Use a manually-specified 128-bit ID */ 00158 } multicast_mpl_seed_id_mode_e; 00159 00160 /** 00161 * \brief Set default MPL Seed Identifier 00162 * 00163 * Sets the default MPL Seed Identifier used when acting as an MPL Seed. 00164 * 00165 * \param interface_id interface id 00166 * \param seed_id_mode Seed ID selection mode 00167 * \param seed_id For positive (constant) types, pointer to Seed ID data 00168 * 00169 * \return 0 for success, negative on failure 00170 */ 00171 int_fast8_t multicast_mpl_set_default_seed_id(int8_t interface_id, 00172 multicast_mpl_seed_id_mode_e seed_id_mode, 00173 const void *seed_id); 00174 00175 /** 00176 * \brief Subscribe to an MPL Domain (RFC 7731) 00177 * 00178 * This subscribes to an MPL Domain with default parameters on the 00179 * specified interface. (At present there is no support for subscribing to 00180 * the same Domain on multiple interfaces) 00181 * 00182 * If the ALL_MPL_FORWARDERS Domain ff03::fc has not already been subscribed 00183 * to, this will automatically also subscribe to it with default parameters. 00184 * 00185 * Once 1 or more MPL Domains have been subscribed to on an interface, 00186 * multicast transmissions sent to a group with scope greater than link-local 00187 * on that interface will be sent using MPL. 00188 * 00189 * If the destination corresponds to a subscribed MPL Domain, it will be sent 00190 * to that MPL Domain (and hence forwarded only by other subscribers to that 00191 * domain). 00192 * 00193 * If the destination does not correspond to a subscribed MPL Domain, it will 00194 * be tunnelled, with the outer IP packet sent to the ALL_MPL_FORWARDERS Domain. 00195 * 00196 * Typical behaviour for ZigBee IP and Thread networks would be achieved by 00197 * subscribing to ff03::1, and enabling realm auto-subscription - [this is 00198 * done automatically when calling multicast_add_address?] 00199 * 00200 * \param interface_id interface id 00201 * \param address MPL Domain Address (IPv6 multicast address) 00202 * \param seed_id_mode Seed ID selection mode 00203 * \param seed_id For positive (constant) types, pointer to Seed ID data 00204 * 00205 * \return 0 for success, negative on failure 00206 */ 00207 int8_t multicast_mpl_domain_subscribe(int8_t interface_id, 00208 const uint8_t address[16], 00209 multicast_mpl_seed_id_mode_e seed_id_mode, 00210 const void *seed_id); 00211 00212 /** 00213 * \brief Subscribe to an MPL Domain (RFC 7731) 00214 * 00215 * This subscribes to an MPL Domain with default parameters on the 00216 * specified interface. 00217 * 00218 * If the ALL_MPL_FORWARDERS Domain ff03::fc has not already been subscribed 00219 * to, this will automatically also subscribe to it with default parameters. 00220 * 00221 * See multicast_mpl_subscribe_domain and multicast_set_default_parameters 00222 * for more information on parameters. 00223 */ 00224 int8_t multicast_mpl_domain_subscribe_with_parameters 00225 (int8_t interface_id, 00226 const uint8_t address[16], 00227 multicast_mpl_seed_id_mode_e seed_id_mode, 00228 const void *seed_id, 00229 bool proactive_forwarding, 00230 uint16_t seed_set_entry_lifetime, 00231 uint32_t data_message_imin, 00232 uint32_t data_message_imax, 00233 uint8_t data_message_k, 00234 uint8_t data_message_timer_expirations, 00235 uint32_t control_message_imin, 00236 uint32_t control_message_imax, 00237 uint8_t control_message_k, 00238 uint8_t control_message_timer_expirations); 00239 00240 /** 00241 * \brief Unsubscribe from an MPL Domain 00242 * 00243 * This subscribes from a previously-subscribed MPL Domain 00244 * 00245 * \param interface_id interface id 00246 * \param address MPL Domain Address (IPv6 multicast address) 00247 * 00248 * \return 0 for success, negative on failure 00249 */ 00250 int8_t multicast_mpl_domain_unsubscribe(int8_t interface_id, 00251 const uint8_t address[16]); 00252 00253 /** 00254 * \brief Add multicast forwarding record to an interface 00255 * 00256 * This adds a group to the forwarding list on the specified interface. 00257 * Received multicast packets sent to the specified group will be forwarded onto 00258 * the specified interface from other interfaces, if IP forwarding is enabled on 00259 * both incoming and outgoing interfaces, subject to a Reverse Path Forwarding 00260 * check on the source address, and usual scope rules. 00261 * 00262 * If a finite lifetime is set, the record will be removed after that many seconds. 00263 * 00264 * If an entry for the specified group already exists, its lifetime will 00265 * increased if it is lower than the value passed. 00266 * 00267 * \param interface_id interface id 00268 * \param group IPv6 multicast group address 00269 * \param lifetime The time in seconds to maintain the forwarding entry - 0xFFFFFFFF means infinite 00270 * 00271 * \return 0 for success, negative on failure 00272 */ 00273 int8_t multicast_fwd_add(int8_t interface_id, const uint8_t group[16], uint32_t lifetime); 00274 00275 /** 00276 * \brief Remove multicast forwarding record from an interface 00277 * 00278 * Delete a a group from the forwarding list on the specified interface. 00279 * 00280 * \param interface_id interface id 00281 * \param group IPv6 multicast group address 00282 * 00283 * \return 0 for success, negative on failure 00284 */ 00285 int8_t multicast_fwd_remove(int8_t interface_id, const uint8_t group[16]); 00286 00287 /** 00288 * \brief Set full multicast forwarding 00289 * 00290 * If enabled, all multicast packets of specified scope or greater will be 00291 * forwarded onto the specified interface from other interfaces, if IP 00292 * forwarding is enabled on both incoming and outgoing interfaces, subject to a 00293 * Reverse Path Forwarding check on the source address. 00294 * 00295 * Setting this is equivalent to "multicast_fwd_add" being called for all 00296 * addresses with scope >= min_scope. 00297 * 00298 * This functionality is disabled by setting min_scope to 0x10 or greater (so 00299 * no packets can match). 00300 * 00301 * \param interface_id interface id 00302 * \param min_scope minimum IPv6 scope value for forwarding (see RFC 4291) 00303 * 00304 * \return 0 for success, negative on failure 00305 */ 00306 int8_t multicast_fwd_full_for_scope(int8_t interface_id, uint_fast8_t min_scope); 00307 00308 /** 00309 * \brief Set upstream interface for MLD proxying 00310 * 00311 * This sets the upstream interface for MLD proxying. If set, the stack will 00312 * report group membership on that interface according to the forwarding lists 00313 * of the other interfaces (ie it will send MLD reports or equivalent on that 00314 * upstream interface). 00315 * 00316 * \param interface_id interface id, or -1 to disable 00317 * 00318 * \return 0 for success, negative on failure 00319 */ 00320 int8_t multicast_fwd_set_proxy_upstream(int8_t interface_id); 00321 00322 #ifdef __cplusplus 00323 } 00324 #endif 00325 #endif /* MULTICAST_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
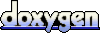