
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mppe.h
00001 /* 00002 * mppe.h - Definitions for MPPE 00003 * 00004 * Copyright (c) 2008 Paul Mackerras. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name(s) of the authors of this software must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. 00021 * 00022 * 4. Redistributions of any form whatsoever must retain the following 00023 * acknowledgment: 00024 * "This product includes software developed by Paul Mackerras 00025 * <paulus@samba.org>". 00026 * 00027 * THE AUTHORS OF THIS SOFTWARE DISCLAIM ALL WARRANTIES WITH REGARD TO 00028 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00029 * AND FITNESS, IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY 00030 * SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00031 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00032 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00033 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00034 */ 00035 00036 #include "netif/ppp/ppp_opts.h" 00037 #if PPP_SUPPORT && MPPE_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00038 00039 #ifndef MPPE_H 00040 #define MPPE_H 00041 00042 #include "netif/ppp/pppcrypt.h" 00043 00044 #define MPPE_PAD 4 /* MPPE growth per frame */ 00045 #define MPPE_MAX_KEY_LEN 16 /* largest key length (128-bit) */ 00046 00047 /* option bits for ccp_options.mppe */ 00048 #define MPPE_OPT_40 0x01 /* 40 bit */ 00049 #define MPPE_OPT_128 0x02 /* 128 bit */ 00050 #define MPPE_OPT_STATEFUL 0x04 /* stateful mode */ 00051 /* unsupported opts */ 00052 #define MPPE_OPT_56 0x08 /* 56 bit */ 00053 #define MPPE_OPT_MPPC 0x10 /* MPPC compression */ 00054 #define MPPE_OPT_D 0x20 /* Unknown */ 00055 #define MPPE_OPT_UNSUPPORTED (MPPE_OPT_56|MPPE_OPT_MPPC|MPPE_OPT_D) 00056 #define MPPE_OPT_UNKNOWN 0x40 /* Bits !defined in RFC 3078 were set */ 00057 00058 /* 00059 * This is not nice ... the alternative is a bitfield struct though. 00060 * And unfortunately, we cannot share the same bits for the option 00061 * names above since C and H are the same bit. We could do a u_int32 00062 * but then we have to do a lwip_htonl() all the time and/or we still need 00063 * to know which octet is which. 00064 */ 00065 #define MPPE_C_BIT 0x01 /* MPPC */ 00066 #define MPPE_D_BIT 0x10 /* Obsolete, usage unknown */ 00067 #define MPPE_L_BIT 0x20 /* 40-bit */ 00068 #define MPPE_S_BIT 0x40 /* 128-bit */ 00069 #define MPPE_M_BIT 0x80 /* 56-bit, not supported */ 00070 #define MPPE_H_BIT 0x01 /* Stateless (in a different byte) */ 00071 00072 /* Does not include H bit; used for least significant octet only. */ 00073 #define MPPE_ALL_BITS (MPPE_D_BIT|MPPE_L_BIT|MPPE_S_BIT|MPPE_M_BIT|MPPE_H_BIT) 00074 00075 /* Build a CI from mppe opts (see RFC 3078) */ 00076 #define MPPE_OPTS_TO_CI(opts, ci) \ 00077 do { \ 00078 u_char *ptr = ci; /* u_char[4] */ \ 00079 \ 00080 /* H bit */ \ 00081 if (opts & MPPE_OPT_STATEFUL) \ 00082 *ptr++ = 0x0; \ 00083 else \ 00084 *ptr++ = MPPE_H_BIT; \ 00085 *ptr++ = 0; \ 00086 *ptr++ = 0; \ 00087 \ 00088 /* S,L bits */ \ 00089 *ptr = 0; \ 00090 if (opts & MPPE_OPT_128) \ 00091 *ptr |= MPPE_S_BIT; \ 00092 if (opts & MPPE_OPT_40) \ 00093 *ptr |= MPPE_L_BIT; \ 00094 /* M,D,C bits not supported */ \ 00095 } while (/* CONSTCOND */ 0) 00096 00097 /* The reverse of the above */ 00098 #define MPPE_CI_TO_OPTS(ci, opts) \ 00099 do { \ 00100 const u_char *ptr = ci; /* u_char[4] */ \ 00101 \ 00102 opts = 0; \ 00103 \ 00104 /* H bit */ \ 00105 if (!(ptr[0] & MPPE_H_BIT)) \ 00106 opts |= MPPE_OPT_STATEFUL; \ 00107 \ 00108 /* S,L bits */ \ 00109 if (ptr[3] & MPPE_S_BIT) \ 00110 opts |= MPPE_OPT_128; \ 00111 if (ptr[3] & MPPE_L_BIT) \ 00112 opts |= MPPE_OPT_40; \ 00113 \ 00114 /* M,D,C bits */ \ 00115 if (ptr[3] & MPPE_M_BIT) \ 00116 opts |= MPPE_OPT_56; \ 00117 if (ptr[3] & MPPE_D_BIT) \ 00118 opts |= MPPE_OPT_D; \ 00119 if (ptr[3] & MPPE_C_BIT) \ 00120 opts |= MPPE_OPT_MPPC; \ 00121 \ 00122 /* Other bits */ \ 00123 if (ptr[0] & ~MPPE_H_BIT) \ 00124 opts |= MPPE_OPT_UNKNOWN; \ 00125 if (ptr[1] || ptr[2]) \ 00126 opts |= MPPE_OPT_UNKNOWN; \ 00127 if (ptr[3] & ~MPPE_ALL_BITS) \ 00128 opts |= MPPE_OPT_UNKNOWN; \ 00129 } while (/* CONSTCOND */ 0) 00130 00131 /* Shared MPPE padding between MSCHAP and MPPE */ 00132 #define SHA1_PAD_SIZE 40 00133 00134 static const u8_t mppe_sha1_pad1[SHA1_PAD_SIZE] = { 00135 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00136 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00137 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00138 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 00139 }; 00140 static const u8_t mppe_sha1_pad2[SHA1_PAD_SIZE] = { 00141 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 00142 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 00143 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 00144 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2, 0xf2 00145 }; 00146 00147 /* 00148 * State for an MPPE (de)compressor. 00149 */ 00150 typedef struct ppp_mppe_state { 00151 lwip_arc4_context arc4; 00152 u8_t master_key[MPPE_MAX_KEY_LEN]; 00153 u8_t session_key[MPPE_MAX_KEY_LEN]; 00154 u8_t keylen; /* key length in bytes */ 00155 /* NB: 128-bit == 16, 40-bit == 8! 00156 * If we want to support 56-bit, the unit has to change to bits 00157 */ 00158 u8_t bits; /* MPPE control bits */ 00159 u16_t ccount; /* 12-bit coherency count (seqno) */ 00160 u16_t sanity_errors; /* take down LCP if too many */ 00161 unsigned int stateful :1; /* stateful mode flag */ 00162 unsigned int discard :1; /* stateful mode packet loss flag */ 00163 } ppp_mppe_state; 00164 00165 void mppe_set_key(ppp_pcb *pcb, ppp_mppe_state *state, u8_t *key); 00166 void mppe_init(ppp_pcb *pcb, ppp_mppe_state *state, u8_t options); 00167 void mppe_comp_reset(ppp_pcb *pcb, ppp_mppe_state *state); 00168 err_t mppe_compress(ppp_pcb *pcb, ppp_mppe_state *state, struct pbuf **pb, u16_t protocol); 00169 void mppe_decomp_reset(ppp_pcb *pcb, ppp_mppe_state *state); 00170 err_t mppe_decompress(ppp_pcb *pcb, ppp_mppe_state *state, struct pbuf **pb); 00171 00172 #endif /* MPPE_H */ 00173 #endif /* PPP_SUPPORT && MPPE_SUPPORT */
Generated on Sun Jul 17 2022 08:25:28 by
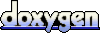