
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
module_reset_mps2.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import os 00019 from host_test_plugins import HostTestPluginBase 00020 from time import sleep 00021 00022 # Note: This plugin is not fully functional, needs improvements 00023 00024 class HostTestPluginResetMethod_MPS2 (HostTestPluginBase): 00025 """ Plugin used to reset ARM_MPS2 platform 00026 Supports: 00027 reboot.txt - startup from standby state, reboots when in run mode. 00028 shutdown.txt - shutdown from run mode. 00029 reset.txt - reset FPGA during run mode. 00030 """ 00031 def touch_file (self, file): 00032 """ Touch file and set timestamp to items 00033 """ 00034 tfile = file+'.tmp' 00035 fhandle = open(tfile, 'a') 00036 try: 00037 fhandle.close() 00038 finally: 00039 os.rename(tfile, file) 00040 return True 00041 00042 # Plugin interface 00043 name = 'HostTestPluginResetMethod_MPS2' 00044 type = 'ResetMethod' 00045 capabilities = ['mps2-reboot', 'mps2-reset'] 00046 required_parameters = ['disk'] 00047 00048 def setup (self, *args, **kwargs): 00049 """ Prepare / configure plugin to work. 00050 This method can receive plugin specific parameters by kwargs and 00051 ignore other parameters which may affect other plugins. 00052 """ 00053 return True 00054 00055 def execute (self, capabilitity, *args, **kwargs): 00056 """ Executes capability by name. 00057 Each capability may directly just call some command line 00058 program or execute building pythonic function 00059 """ 00060 return True 00061 result = False 00062 if self.check_parameters(capabilitity, *args, **kwargs) is True: 00063 disk = kwargs['disk'] 00064 00065 if capabilitity == 'mps2-reboot' and self.touch_file (disk + 'reboot.txt'): 00066 sleep(20) 00067 result = True 00068 00069 elif capabilitity == 'mps2-reset' and self.touch_file (disk + 'reboot.txt'): 00070 sleep(20) 00071 result = True 00072 00073 return result 00074 00075 def load_plugin (): 00076 """ Returns plugin available in this module 00077 """ 00078 return HostTestPluginResetMethod_MPS2()
Generated on Sun Jul 17 2022 08:25:28 by
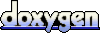