
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
module_copy_smart.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import os 00019 import sys 00020 from os.path import join, basename, exists, abspath, dirname 00021 from time import sleep 00022 from host_test_plugins import HostTestPluginBase 00023 00024 sys.path.append(abspath(join(dirname(__file__), "../../../"))) 00025 import tools.test_api 00026 00027 class HostTestPluginCopyMethod_Smart(HostTestPluginBase): 00028 00029 # Plugin interface 00030 name = 'HostTestPluginCopyMethod_Smart' 00031 type = 'CopyMethod' 00032 stable = True 00033 capabilities = ['smart'] 00034 required_parameters = ['image_path', 'destination_disk', 'target_mcu'] 00035 00036 def setup(self, *args, **kwargs): 00037 """ Configure plugin, this function should be called before plugin execute() method is used. 00038 """ 00039 return True 00040 00041 def execute(self, capability, *args, **kwargs): 00042 """ Executes capability by name. 00043 Each capability may directly just call some command line 00044 program or execute building pythonic function 00045 """ 00046 result = False 00047 if self.check_parameters(capability, *args, **kwargs) is True: 00048 image_path = kwargs['image_path'] 00049 destination_disk = kwargs['destination_disk'] 00050 target_mcu = kwargs['target_mcu'] 00051 # Wait for mount point to be ready 00052 self.check_mount_point_ready(destination_disk) # Blocking 00053 # Prepare correct command line parameter values 00054 image_base_name = basename(image_path) 00055 destination_path = join(destination_disk, image_base_name) 00056 if capability == 'smart': 00057 if os.name == 'posix': 00058 cmd = ['cp', image_path, destination_path] 00059 result = self.run_command(cmd, shell=False) 00060 00061 cmd = ['sync'] 00062 result = self.run_command(cmd, shell=False) 00063 elif os.name == 'nt': 00064 cmd = ['copy', image_path, destination_path] 00065 result = self.run_command(cmd, shell=True) 00066 00067 # Give the OS and filesystem time to settle down 00068 sleep(3) 00069 00070 platform_name_filter = [target_mcu] 00071 muts_list = {} 00072 00073 remount_complete = False 00074 00075 for i in range(0, 60): 00076 print('Looking for %s with MBEDLS' % target_mcu) 00077 muts_list = tools.test_api.get_autodetected_MUTS_list(platform_name_filter=platform_name_filter) 00078 00079 if 1 in muts_list: 00080 mut = muts_list[1] 00081 destination_disk = mut['disk'] 00082 destination_path = join(destination_disk, image_base_name) 00083 00084 if mut['mcu'] == 'LPC1768' or mut['mcu'] == 'LPC11U24': 00085 if exists(destination_disk) and exists(destination_path): 00086 remount_complete = True 00087 break; 00088 else: 00089 if exists(destination_disk) and not exists(destination_path): 00090 remount_complete = True 00091 break; 00092 00093 sleep(1) 00094 00095 if remount_complete: 00096 print('Remount complete') 00097 else: 00098 print('Remount FAILED') 00099 00100 if exists(destination_disk): 00101 print('Disk exists') 00102 else: 00103 print('Disk does not exist') 00104 00105 if exists(destination_path): 00106 print('Image exists') 00107 else: 00108 print('Image does not exist') 00109 00110 result = None 00111 00112 00113 return result 00114 00115 def load_plugin (): 00116 """ Returns plugin available in this module 00117 """ 00118 return HostTestPluginCopyMethod_Smart()
Generated on Sun Jul 17 2022 08:25:28 by
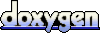