
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
module_copy_silabs.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 from host_test_plugins import HostTestPluginBase 00019 from time import sleep 00020 00021 00022 class HostTestPluginCopyMethod_Silabs(HostTestPluginBase): 00023 00024 # Plugin interface 00025 name = 'HostTestPluginCopyMethod_Silabs' 00026 type = 'CopyMethod' 00027 capabilities = ['eACommander', 'eACommander-usb'] 00028 required_parameters = ['image_path', 'destination_disk', 'program_cycle_s'] 00029 00030 def setup(self, *args, **kwargs): 00031 """ Configure plugin, this function should be called before plugin execute() method is used. 00032 """ 00033 self.EACOMMANDER_CMD = 'eACommander.exe' 00034 return True 00035 00036 def execute(self, capabilitity, *args, **kwargs): 00037 """ Executes capability by name. 00038 Each capability may directly just call some command line 00039 program or execute building pythonic function 00040 """ 00041 result = False 00042 if self.check_parameters(capabilitity, *args, **kwargs) is True: 00043 image_path = kwargs['image_path'] 00044 destination_disk = kwargs['destination_disk'] 00045 program_cycle_s = kwargs['program_cycle_s'] 00046 if capabilitity == 'eACommander': 00047 cmd = [self.EACOMMANDER_CMD, 00048 '--serialno', destination_disk, 00049 '--flash', image_path, 00050 '--resettype', '2', '--reset'] 00051 result = self.run_command(cmd) 00052 elif capabilitity == 'eACommander-usb': 00053 cmd = [self.EACOMMANDER_CMD, 00054 '--usb', destination_disk, 00055 '--flash', image_path] 00056 result = self.run_command(cmd) 00057 00058 # Allow mbed to cycle 00059 sleep(program_cycle_s) 00060 00061 return result 00062 00063 00064 def load_plugin (): 00065 """ Returns plugin available in this module 00066 """ 00067 return HostTestPluginCopyMethod_Silabs()
Generated on Sun Jul 17 2022 08:25:28 by
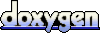