
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
module_copy_shell.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import os 00019 from os.path import join, basename 00020 from host_test_plugins import HostTestPluginBase 00021 from time import sleep 00022 00023 00024 class HostTestPluginCopyMethod_Shell(HostTestPluginBase): 00025 00026 # Plugin interface 00027 name = 'HostTestPluginCopyMethod_Shell' 00028 type = 'CopyMethod' 00029 stable = True 00030 capabilities = ['shell', 'cp', 'copy', 'xcopy'] 00031 required_parameters = ['image_path', 'destination_disk', 'program_cycle_s'] 00032 00033 def setup(self, *args, **kwargs): 00034 """ Configure plugin, this function should be called before plugin execute() method is used. 00035 """ 00036 return True 00037 00038 def execute(self, capability, *args, **kwargs): 00039 """ Executes capability by name. 00040 Each capability may directly just call some command line 00041 program or execute building pythonic function 00042 """ 00043 result = False 00044 if self.check_parameters(capability, *args, **kwargs) is True: 00045 image_path = kwargs['image_path'] 00046 destination_disk = kwargs['destination_disk'] 00047 program_cycle_s = kwargs['program_cycle_s'] 00048 # Wait for mount point to be ready 00049 self.check_mount_point_ready(destination_disk) # Blocking 00050 # Prepare correct command line parameter values 00051 image_base_name = basename(image_path) 00052 destination_path = join(destination_disk, image_base_name) 00053 if capability == 'shell': 00054 if os.name == 'nt': capability = 'copy' 00055 elif os.name == 'posix': capability = 'cp' 00056 if capability == 'cp' or capability == 'copy' or capability == 'copy': 00057 copy_method = capability 00058 cmd = [copy_method, image_path, destination_path] 00059 if os.name == 'posix': 00060 result = self.run_command(cmd, shell=False) 00061 result = self.run_command(["sync"]) 00062 else: 00063 result = self.run_command(cmd) 00064 00065 # Allow mbed to cycle 00066 sleep(program_cycle_s) 00067 00068 return result 00069 00070 00071 def load_plugin (): 00072 """ Returns plugin available in this module 00073 """ 00074 return HostTestPluginCopyMethod_Shell()
Generated on Sun Jul 17 2022 08:25:28 by
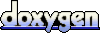