
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
module_copy_mps2.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import re 00019 import os, shutil 00020 from os.path import join 00021 from host_test_plugins import HostTestPluginBase 00022 from time import sleep 00023 00024 00025 class HostTestPluginCopyMethod_MPS2(HostTestPluginBase): 00026 00027 # MPS2 specific flashing / binary setup funcitons 00028 def mps2_set_board_image_file(self, disk, images_cfg_path, image0file_path, image_name='images.txt'): 00029 """ This function will alter image cfg file. 00030 Main goal of this function is to change number of images to 1, comment all 00031 existing image entries and append at the end of file new entry with test path. 00032 @return True when all steps succeed. 00033 """ 00034 MBED_SDK_TEST_STAMP = 'test suite entry' 00035 image_path = join(disk, images_cfg_path, image_name) 00036 new_file_lines = [] # New configuration file lines (entries) 00037 00038 # Check each line of the image configuration file 00039 try: 00040 with open(image_path, 'r') as file: 00041 for line in file: 00042 if re.search('^TOTALIMAGES', line): 00043 # Check number of total images, should be 1 00044 new_file_lines.append(re.sub('^TOTALIMAGES:[\t ]*[\d]+', 'TOTALIMAGES: 1', line)) 00045 elif re.search('; - %s[\n\r]*$'% MBED_SDK_TEST_STAMP, line): 00046 # Look for test suite entries and remove them 00047 pass # Omit all test suite entries 00048 elif re.search('^IMAGE[\d]+FILE', line): 00049 # Check all image entries and mark the ';' 00050 new_file_lines.append(';' + line) # Comment non test suite lines 00051 else: 00052 # Append line to new file 00053 new_file_lines.append(line) 00054 except IOError as e: 00055 return False 00056 00057 # Add new image entry with proper commented stamp 00058 new_file_lines.append('IMAGE0FILE: %s ; - %s\r\n'% (image0file_path, MBED_SDK_TEST_STAMP)) 00059 00060 # Write all lines to file 00061 try: 00062 with open(image_path, 'w') as file: 00063 for line in new_file_lines: 00064 file.write(line), 00065 except IOError: 00066 return False 00067 00068 return True 00069 00070 def mps2_select_core(self, disk, mobo_config_name=""): 00071 """ Function selects actual core 00072 """ 00073 # TODO: implement core selection 00074 pass 00075 00076 def mps2_switch_usb_auto_mounting_after_restart(self, disk, usb_config_name=""): 00077 """ Function alters configuration to allow USB MSD to be mounted after restarts 00078 """ 00079 # TODO: implement USB MSD restart detection 00080 pass 00081 00082 def copy_file(self, file, disk): 00083 if not file: 00084 return 00085 00086 _, ext = os.path.splitext(file) 00087 ext = ext.lower() 00088 dfile = disk + "/SOFTWARE/mbed" + ext 00089 00090 if os.path.isfile(dfile): 00091 print('Remove old binary %s' % dfile) 00092 os.remove(dfile) 00093 00094 shutil.copy(file, dfile) 00095 return True 00096 00097 def touch_file(self, file): 00098 """ Touch file and set timestamp to items 00099 """ 00100 tfile = file+'.tmp' 00101 fhandle = open(tfile, 'a') 00102 try: 00103 fhandle.close() 00104 finally: 00105 os.rename(tfile, file) 00106 return True 00107 00108 # Plugin interface 00109 name = 'HostTestPluginCopyMethod_MPS2' 00110 type = 'CopyMethod' 00111 capabilities = ['mps2-copy'] 00112 required_parameters = ['image_path', 'destination_disk'] 00113 00114 def setup(self, *args, **kwargs): 00115 """ Configure plugin, this function should be called before plugin execute() method is used. 00116 """ 00117 return True 00118 00119 def execute(self, capabilitity, *args, **kwargs): 00120 """ Executes capability by name. 00121 Each capability may directly just call some command line 00122 program or execute building pythonic function 00123 """ 00124 result = False 00125 if self.check_parameters(capabilitity, *args, **kwargs) is True: 00126 file = kwargs['image_path'] 00127 disk = kwargs['destination_disk'] 00128 00129 """ Add a delay in case there a test just finished 00130 Prevents interface firmware hiccups 00131 """ 00132 sleep(20) 00133 if capabilitity == 'mps2-copy' and self.copy_file(file, disk): 00134 sleep(3) 00135 if self.touch_file(disk + 'reboot.txt'): 00136 """ Add a delay after the board was rebooted. 00137 The actual reboot time is 20 seconds, but using 15 seconds 00138 allows us to open the COM port and save a board reset. 00139 This also prevents interface firmware hiccups. 00140 """ 00141 sleep(7) 00142 result = True 00143 00144 return result 00145 00146 00147 def load_plugin (): 00148 """ Returns plugin available in this module 00149 """ 00150 return HostTestPluginCopyMethod_MPS2()
Generated on Sun Jul 17 2022 08:25:28 by
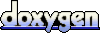