
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mesh_system.c
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <stdlib.h> 00018 #include "eventOS_scheduler.h" 00019 #include "eventOS_event.h" 00020 #include "net_interface.h" 00021 #include "nsdynmemLIB.h" 00022 #include "randLIB.h" 00023 #include "platform/arm_hal_timer.h" 00024 #include "ns_hal_init.h" 00025 #include "include/mesh_system.h" 00026 #include "mbed_assert.h" 00027 // For tracing we need to define flag, have include and define group 00028 #define HAVE_DEBUG 1 00029 #include "ns_trace.h" 00030 #define TRACE_GROUP "m6-mesh-system" 00031 00032 /* Heap for NanoStack */ 00033 #if !MBED_CONF_MBED_MESH_API_USE_MALLOC_FOR_HEAP 00034 static uint8_t app_stack_heap[MBED_CONF_MBED_MESH_API_HEAP_SIZE + 1]; 00035 #else 00036 static uint8_t *app_stack_heap; 00037 #endif 00038 static bool mesh_initialized = false; 00039 00040 /* 00041 * Heap error handler, called when heap problem is detected. 00042 * Function is for-ever loop. 00043 */ 00044 static void mesh_system_heap_error_handler(heap_fail_t event) 00045 { 00046 tr_error("Heap error, mesh_system_heap_error_handler() %d", event); 00047 switch (event) { 00048 case NS_DYN_MEM_NULL_FREE: 00049 case NS_DYN_MEM_DOUBLE_FREE: 00050 case NS_DYN_MEM_ALLOCATE_SIZE_NOT_VALID: 00051 case NS_DYN_MEM_POINTER_NOT_VALID: 00052 case NS_DYN_MEM_HEAP_SECTOR_CORRUPTED: 00053 case NS_DYN_MEM_HEAP_SECTOR_UNITIALIZED: 00054 break; 00055 default: 00056 break; 00057 } 00058 while (1); 00059 } 00060 00061 void mesh_system_init(void) 00062 { 00063 if (mesh_initialized == false) { 00064 #if MBED_CONF_MBED_MESH_API_USE_MALLOC_FOR_HEAP 00065 app_stack_heap = malloc(MBED_CONF_MBED_MESH_API_HEAP_SIZE+1); 00066 MBED_ASSERT(app_stack_heap); 00067 #endif 00068 ns_hal_init(app_stack_heap, MBED_CONF_MBED_MESH_API_HEAP_SIZE, 00069 mesh_system_heap_error_handler, NULL); 00070 eventOS_scheduler_mutex_wait(); 00071 net_init_core(); 00072 eventOS_scheduler_mutex_release(); 00073 mesh_initialized = true; 00074 } 00075 } 00076 00077 void mesh_system_send_connect_event(uint8_t receiver) 00078 { 00079 arm_event_s event = { 00080 .sender = receiver, 00081 .event_id = APPL_EVENT_CONNECT, 00082 .receiver = receiver, 00083 .event_type = APPLICATION_EVENT, 00084 .priority = ARM_LIB_LOW_PRIORITY_EVENT, 00085 }; 00086 eventOS_event_send(&event); 00087 }
Generated on Sun Jul 17 2022 08:25:28 by
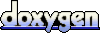