
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
memap_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2017 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 import sys 00018 from os.path import isfile, join 00019 import json 00020 00021 import pytest 00022 00023 from tools.memap import MemapParser 00024 from copy import deepcopy 00025 00026 """ 00027 Tests for test_api.py 00028 """ 00029 00030 @pytest.fixture 00031 def memap_parser (): 00032 """ 00033 Called before each test case 00034 00035 :return: 00036 """ 00037 memap_parser = MemapParser() 00038 00039 memap_parser.modules = { 00040 "mbed-os/targets/TARGET/TARGET_MCUS/api/pinmap.o": { 00041 ".text": 1, 00042 ".data": 2, 00043 ".bss": 3, 00044 ".heap": 0, 00045 ".stack": 0, 00046 ".interrupts_ram":0, 00047 ".init":0, 00048 ".ARM.extab":0, 00049 ".ARM.exidx":0, 00050 ".ARM.attributes":0, 00051 ".eh_frame":0, 00052 ".init_array":0, 00053 ".fini_array":0, 00054 ".jcr":0, 00055 ".stab":0, 00056 ".stabstr":0, 00057 ".ARM.exidx":0, 00058 ".ARM":0, 00059 ".interrupts":0, 00060 ".flash_config":0, 00061 "unknown":0, 00062 "OUTPUT":0, 00063 }, 00064 "[lib]/libc.a/lib_a-printf.o": { 00065 ".text": 4, 00066 ".data": 5, 00067 ".bss": 6, 00068 ".heap": 0, 00069 ".stack": 0, 00070 ".interrupts_ram":0, 00071 ".init":0, 00072 ".ARM.extab":0, 00073 ".ARM.exidx":0, 00074 ".ARM.attributes":0, 00075 ".eh_frame":0, 00076 ".init_array":0, 00077 ".fini_array":0, 00078 ".jcr":0, 00079 ".stab":0, 00080 ".stabstr":0, 00081 ".ARM.exidx":0, 00082 ".ARM":0, 00083 ".interrupts":0, 00084 ".flash_config":0, 00085 "unknown":0, 00086 "OUTPUT":0, 00087 }, 00088 "main.o": { 00089 ".text": 7, 00090 ".data": 8, 00091 ".bss": 0, 00092 ".heap": 0, 00093 ".stack": 0, 00094 ".interrupts_ram":0, 00095 ".init":0, 00096 ".ARM.extab":0, 00097 ".ARM.exidx":0, 00098 ".ARM.attributes":0, 00099 ".eh_frame":0, 00100 ".init_array":0, 00101 ".fini_array":0, 00102 ".jcr":0, 00103 ".stab":0, 00104 ".stabstr":0, 00105 ".ARM.exidx":0, 00106 ".ARM":0, 00107 ".interrupts":0, 00108 ".flash_config":0, 00109 "unknown":0, 00110 "OUTPUT":0, 00111 }, 00112 "test.o": { 00113 ".text": 0, 00114 ".data": 0, 00115 ".bss": 0, 00116 ".heap": 0, 00117 ".stack": 0, 00118 ".interrupts_ram":0, 00119 ".init":0, 00120 ".ARM.extab":0, 00121 ".ARM.exidx":0, 00122 ".ARM.attributes":0, 00123 ".eh_frame":0, 00124 ".init_array":0, 00125 ".fini_array":0, 00126 ".jcr":0, 00127 ".stab":0, 00128 ".stabstr":0, 00129 ".ARM.exidx":0, 00130 ".ARM":0, 00131 ".interrupts":0, 00132 ".flash_config":0, 00133 "unknown":0, 00134 "OUTPUT":0, 00135 }, 00136 } 00137 return memap_parser 00138 00139 00140 def generate_test_helper (memap_parser, format, depth, file_output=None): 00141 """ 00142 Helper that tests that the member variables "modules" is 00143 unchanged after calling "generate_output" 00144 00145 :param memap_parser: the parser object 00146 :param depth: how much detail to put in the report 00147 :param format: the file type to output 00148 :param file_output: the file to output to 00149 """ 00150 00151 old_modules = deepcopy(memap_parser.modules) 00152 00153 memap_parser.generate_output(format, depth, file_output=file_output) 00154 00155 assert memap_parser.modules == old_modules,\ 00156 "generate_output modified the 'modules' property" 00157 00158 00159 @pytest.mark.parametrize("depth", [1, 2, 20]) 00160 def test_report_computed (memap_parser, depth): 00161 """ 00162 Test that a report and summary are computed 00163 00164 :param memap_parser: Mocked parser 00165 :param depth: the detail of the output 00166 """ 00167 00168 memap_parser.generate_output('table', depth) 00169 00170 # Report is created after generating output 00171 assert memap_parser.mem_summary 00172 assert memap_parser.mem_report 00173 00174 00175 @pytest.mark.parametrize("depth", [1, 2, 20]) 00176 def test_generate_output_table (memap_parser, depth): 00177 """ 00178 Test that an output of type "table" can be generated correctly 00179 :param memap_parser: Mocked parser 00180 :param depth: the detail of the output 00181 """ 00182 generate_test_helper(memap_parser, 'table', depth) 00183 00184 00185 @pytest.mark.parametrize("depth", [1, 2, 20]) 00186 def test_generate_output_json (memap_parser, tmpdir, depth): 00187 """ 00188 Test that an output of type "json" can be generated correctly 00189 :param memap_parser: Mocked parser 00190 :param tmpdir: a unique location to place an output file 00191 :param depth: the detail of the output 00192 """ 00193 file_name = str(tmpdir.join('output.json').realpath()) 00194 generate_test_helper(memap_parser, 'json', depth, file_name) 00195 assert isfile(file_name), "Failed to create json file" 00196 json.load(open(file_name)) 00197 00198 00199 @pytest.mark.parametrize("depth", [1, 2, 20]) 00200 def test_generate_output_csv_ci (memap_parser, tmpdir, depth): 00201 """ 00202 Test ensures that an output of type "csv-ci" can be generated correctly 00203 00204 :param memap_parser: Mocked parser 00205 :param tmpdir: a unique location to place an output file 00206 :param depth: the detail of the output 00207 """ 00208 file_name = str(tmpdir.join('output.csv').realpath()) 00209 generate_test_helper(memap_parser, 'csv-ci', depth, file_name) 00210 assert isfile(file_name), "Failed to create csv-ci file"
Generated on Sun Jul 17 2022 08:25:28 by
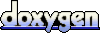