
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mbedrpc.py
00001 # mbedRPC.py - mbed RPC interface for Python 00002 # 00003 ##Copyright (c) 2010 ARM Ltd 00004 ## 00005 ##Permission is hereby granted, free of charge, to any person obtaining a copy 00006 ##of this software and associated documentation files (the "Software"), to deal 00007 ##in the Software without restriction, including without limitation the rights 00008 ##to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 ##copies of the Software, and to permit persons to whom the Software is 00010 ##furnished to do so, subject to the following conditions: 00011 ## 00012 ##The above copyright notice and this permission notice shall be included in 00013 ##all copies or substantial portions of the Software. 00014 ## 00015 ##THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 ##IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 ##FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 ##AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 ##LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 ##OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 ##THE SOFTWARE. 00022 # 00023 # Example: 00024 # >from mbedRPC import* 00025 # >mbed = SerialRPC("COM5",9600) 00026 # >myled = DigitalOut(mbed,"myled") <--- Where the text in quotations matches your RPC pin definition's second parameter, in this case it could be RpcDigitalOut myled(LED1,"myled"); 00027 # >myled.write(1) 00028 # > 00029 00030 import serial, urllib2, time 00031 00032 # mbed super class 00033 class mbed: 00034 def __init__(self): 00035 print("This will work as a demo but no transport mechanism has been selected") 00036 00037 def rpc(self, name, method, args): 00038 print("Superclass method not overridden") 00039 00040 00041 # Transport mechanisms, derived from mbed 00042 class SerialRPC(mbed): 00043 def __init__(self, port, baud): 00044 self.ser = serial.Serial(port) 00045 self.ser.setBaudrate(baud) 00046 00047 def rpc(self, name, method, args): 00048 # creates the command to be sent serially - /name/method arg1 arg2 arg3 ... argN 00049 str = "/" + name + "/" + method + " " + " ".join(args) + "\n" 00050 # prints the command being executed 00051 print str 00052 # writes the command to serial 00053 self.ser.write(str) 00054 # strips trailing characters from the line just written 00055 ret_val = self.ser.readline().strip() 00056 return ret_val 00057 00058 00059 class HTTPRPC(mbed): 00060 def __init__(self, ip): 00061 self.host = "http://" + ip 00062 00063 def rpc(self, name, method, args): 00064 response = urllib2.urlopen(self.host + "/rpc/" + name + "/" + method + "%20" + "%20".join(args)) 00065 return response.read().strip() 00066 00067 00068 # generic mbed interface super class 00069 class mbed_interface(): 00070 # initialize an mbed interface with a transport mechanism and pin name 00071 def __init__(self, this_mbed, mpin): 00072 self.mbed = this_mbed 00073 if isinstance(mpin, str): 00074 self.name = mpin 00075 00076 def __del__(self): 00077 r = self.mbed.rpc(self.name, "delete", []) 00078 00079 def new(self, class_name, name, pin1, pin2 = "", pin3 = ""): 00080 args = [arg for arg in [pin1,pin2,pin3,name] if arg != ""] 00081 r = self.mbed.rpc(class_name, "new", args) 00082 00083 # generic read 00084 def read(self): 00085 r = self.mbed.rpc(self.name, "read", []) 00086 return int(r) 00087 00088 00089 # for classes that need write functionality - inherits from the generic reading interface 00090 class mbed_interface_write(mbed_interface): 00091 def __init__(self, this_mbed, mpin): 00092 mbed_interface.__init__(self, this_mbed, mpin) 00093 00094 # generic write 00095 def write(self, value): 00096 r = self.mbed.rpc(self.name, "write", [str(value)]) 00097 00098 00099 # mbed interfaces 00100 class DigitalOut(mbed_interface_write): 00101 def __init__(self, this_mbed, mpin): 00102 mbed_interface_write.__init__(self, this_mbed, mpin) 00103 00104 00105 class AnalogIn(mbed_interface): 00106 def __init__(self, this_mbed, mpin): 00107 mbed_interface.__init__(self, this_mbed, mpin) 00108 00109 def read_u16(self): 00110 r = self.mbed.rpc(self.name, "read_u16", []) 00111 return int(r) 00112 00113 00114 class AnalogOut(mbed_interface_write): 00115 def __init__(self, this_mbed, mpin): 00116 mbed_interface_write.__init__(self, this_mbed, mpin) 00117 00118 def write_u16(self, value): 00119 self.mbed.rpc(self.name, "write_u16", [str(value)]) 00120 00121 def read(self): 00122 r = self.mbed.rpc(self.name, "read", []) 00123 return float(r) 00124 00125 00126 class DigitalIn(mbed_interface): 00127 def __init__(self, this_mbed, mpin): 00128 mbed_interface.__init__(self, this_mbed, mpin) 00129 00130 00131 class PwmOut(mbed_interface_write): 00132 def __init__(self, this_mbed, mpin): 00133 mbed_interface_write.__init__(self, this_mbed, mpin) 00134 00135 def read(self): 00136 r = self.mbed.rpc(self.name, "read", []) 00137 return r 00138 00139 def period(self, value): 00140 self.mbed.rpc(self.name, "period", [str(value)]) 00141 00142 def period_ms(self, value): 00143 self.mbed.rpc(self.name, "period_ms", [str(value)]) 00144 00145 def period_us(self, value): 00146 self.mbed.rpc(self.name, "period_us", [str(value)]) 00147 00148 def pulsewidth(self, value): 00149 self.mbed.rpc(self.name, "pulsewidth", [str(value)]) 00150 00151 def pulsewidth_ms(self, value): 00152 self.mbed.rpc(self.name, "pulsewidth_ms", [str(value)]) 00153 00154 def pulsewidth_us(self, value): 00155 self.mbed.rpc(self.name, "pulsewidth_us", [str(value)]) 00156 00157 00158 class RPCFunction(mbed_interface): 00159 def __init__(self, this_mbed, name): 00160 mbed_interface.__init__(self, this_mbed, name) 00161 00162 def run(self, input): 00163 r = self.mbed.rpc(self.name, "run", [input]) 00164 return r 00165 00166 00167 class RPCVariable(mbed_interface_write): 00168 def __init__(self, this_mbed, name): 00169 mbed_interface_write.__init__(self, this_mbed, name) 00170 00171 def read(self): 00172 r = self.mbed.rpc(self.name, "read", []) 00173 return r 00174 00175 class Timer(mbed_interface): 00176 def __init__(self, this_mbed, name): 00177 mbed_interface.__init__(self, this_mbed, name) 00178 00179 def start(self): 00180 r = self.mbed.rpc(self.name, "start", []) 00181 00182 def stop(self): 00183 r = self.mbed.rpc(self.name, "stop", []) 00184 00185 def reset(self): 00186 r = self.mbed.rpc(self.name, "reset", []) 00187 00188 def read(self): 00189 r = self.mbed.rpc(self.name, "read", []) 00190 return float(re.search('\d+\.*\d*', r).group(0)) 00191 00192 def read_ms(self): 00193 r = self.mbed.rpc(self.name, "read_ms", []) 00194 return float(re.search('\d+\.*\d*', r).group(0)) 00195 00196 def read_us(self): 00197 r = self.mbed.rpc(self.name, "read_us", []) 00198 return float(re.search('\d+\.*\d*', r).group(0)) 00199 00200 # Serial 00201 class Serial(): 00202 def __init__(self, this_mbed, tx, rx=""): 00203 self.mbed = this_mbed 00204 if isinstance(tx, str): 00205 self.name = tx 00206 00207 def __del__(self): 00208 r = self.mbed.rpc(self.name, "delete", []) 00209 00210 def baud(self, value): 00211 r = self.mbed.rpc(self.name, "baud", [str(value)]) 00212 00213 def putc(self, value): 00214 r = self.mbed.rpc(self.name, "putc", [str(value)]) 00215 00216 def puts(self, value): 00217 r = self.mbed.rpc(self.name, "puts", ["\"" + str(value) + "\""]) 00218 00219 def getc(self): 00220 r = self.mbed.rpc(self.name, "getc", []) 00221 return int(r) 00222 00223 00224 def wait(s): 00225 time.sleep(s)
Generated on Sun Jul 17 2022 08:25:27 by
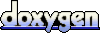