
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mbed_sleep_manager.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed_sleep.h" 00018 #include "mbed_critical.h" 00019 #include "sleep_api.h" 00020 #include "mbed_error.h" 00021 #include <limits.h> 00022 00023 #if DEVICE_SLEEP 00024 00025 // deep sleep locking counter. A target is allowed to deep sleep if counter == 0 00026 static uint16_t deep_sleep_lock = 0U; 00027 00028 void sleep_manager_lock_deep_sleep(void) 00029 { 00030 core_util_critical_section_enter(); 00031 if (deep_sleep_lock == USHRT_MAX) { 00032 core_util_critical_section_exit(); 00033 error("Deep sleep lock would overflow (> USHRT_MAX)"); 00034 } 00035 core_util_atomic_incr_u16(&deep_sleep_lock, 1); 00036 core_util_critical_section_exit(); 00037 } 00038 00039 void sleep_manager_unlock_deep_sleep(void) 00040 { 00041 core_util_critical_section_enter(); 00042 if (deep_sleep_lock == 0) { 00043 core_util_critical_section_exit(); 00044 error("Deep sleep lock would underflow (< 0)"); 00045 } 00046 core_util_atomic_decr_u16(&deep_sleep_lock, 1); 00047 core_util_critical_section_exit(); 00048 } 00049 00050 bool sleep_manager_can_deep_sleep(void) 00051 { 00052 return deep_sleep_lock == 0 ? true : false; 00053 } 00054 00055 void sleep_manager_sleep_auto(void) 00056 { 00057 core_util_critical_section_enter(); 00058 // debug profile should keep debuggers attached, no deep sleep allowed 00059 #ifdef MBED_DEBUG 00060 hal_sleep(); 00061 #else 00062 if (sleep_manager_can_deep_sleep()) { 00063 hal_deepsleep(); 00064 } else { 00065 hal_sleep(); 00066 } 00067 #endif 00068 core_util_critical_section_exit(); 00069 } 00070 00071 #else 00072 00073 // locking is valid only if DEVICE_SLEEP is defined 00074 // we provide empty implementation 00075 00076 void sleep_manager_lock_deep_sleep(void) 00077 { 00078 00079 } 00080 00081 void sleep_manager_unlock_deep_sleep(void) 00082 { 00083 00084 } 00085 00086 bool sleep_manager_can_deep_sleep(void) 00087 { 00088 // no sleep implemented 00089 return false; 00090 } 00091 00092 #endif
Generated on Sun Jul 17 2022 08:25:27 by
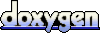