
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "test_env.h" 00004 #include "rtos.h" 00005 00006 #if defined(MBED_RTOS_SINGLE_THREAD) 00007 #error [NOT_SUPPORTED] test not supported 00008 #endif 00009 00010 DigitalOut led2(LED2); 00011 00012 #define SIZE 100 00013 00014 namespace { 00015 // Allocate data buffers 00016 uint8_t data_written[SIZE] = { 0 }; 00017 uint8_t data_read[SIZE] = { 0 }; 00018 } 00019 00020 void sd_thread(void const *argument) 00021 { 00022 const char *FILE_NAME = "/sd/rtos9_test.txt"; 00023 00024 #if defined(TARGET_KL25Z) 00025 SDFileSystem sd(PTD2, PTD3, PTD1, PTD0, "sd"); 00026 00027 #elif defined(TARGET_KL46Z) || defined(TARGET_KL43Z) || defined(TARGET_KL82Z) 00028 SDFileSystem sd(PTD6, PTD7, PTD5, PTD4, "sd"); 00029 00030 #elif defined(TARGET_K64F) || defined(TARGET_K66F) 00031 SDFileSystem sd(PTD2, PTD3, PTD1, PTD0, "sd"); 00032 00033 #elif defined(TARGET_RZ_A1H) 00034 SDFileSystem sd(P8_5, P8_6, P8_3, P8_4, "sd"); 00035 00036 #elif defined(TARGET_NUMAKER_PFM_NUC472) 00037 SDFileSystem sd(PF_0, PD_15, PD_14, PD_13, "sd"); 00038 00039 #elif defined(TARGET_NUMAKER_PFM_M453) 00040 SDFileSystem sd(PD_13, PD_14, PD_15, PD_12, "sd"); 00041 00042 #elif defined(TARGET_NUMAKER_PFM_M487) 00043 SDFileSystem sd(D11, D12, D13, D10, "sd"); 00044 00045 #else 00046 SDFileSystem sd(p11, p12, p13, p14, "sd"); 00047 #endif 00048 00049 { 00050 // fill data_written buffer with random data 00051 FILE *f = fopen(FILE_NAME, "w+"); 00052 if (f) { 00053 // write these data into the file 00054 printf("Writing %d bytes to file:" NL, SIZE); 00055 for (int i = 0; i < SIZE; i++) { 00056 data_written[i] = rand() % 0xff; 00057 fprintf(f, "%c", data_written[i]); 00058 printf("%02X ", data_written[i]); 00059 if (i && ((i % 20) == 19)) 00060 printf(NL); 00061 } 00062 fclose(f); 00063 printf("MBED: Done" NL); 00064 } else { 00065 printf("MBED: Can't open '%s'" NL, FILE_NAME); 00066 MBED_HOSTTEST_RESULT(false); 00067 } 00068 } 00069 00070 printf(NL); 00071 00072 { 00073 // read back the data from the file and store them in data_read 00074 FILE *f = fopen(FILE_NAME, "r"); 00075 if (f) { 00076 printf("MBED: Reading %d bytes from file:" NL, SIZE); 00077 for (int i = 0; i < SIZE; i++) { 00078 data_read[i] = fgetc(f); 00079 printf("%02X ", data_read[i]); 00080 if (i && ((i % 20) == 19)) 00081 printf(NL); 00082 } 00083 fclose(f); 00084 printf("MBED: Done\r\n"); 00085 } else { 00086 printf("MBED: Can't open '%s'" NL, FILE_NAME); 00087 MBED_HOSTTEST_RESULT(false); 00088 } 00089 } 00090 00091 // check that the data written == data read 00092 for (int i = 0; i < SIZE; i++) { 00093 if (data_written[i] != data_read[i]) { 00094 printf("MBED: Data index=%d: w[0x%02X] != r[0x%02X]" NL, i, data_written[i], data_read[i]); 00095 MBED_HOSTTEST_RESULT(false); 00096 } 00097 } 00098 MBED_HOSTTEST_RESULT(true); 00099 } 00100 00101 int main() { 00102 MBED_HOSTTEST_TIMEOUT(20); 00103 MBED_HOSTTEST_SELECT(default_auto); 00104 MBED_HOSTTEST_DESCRIPTION(SD File write read); 00105 MBED_HOSTTEST_START("RTOS_9"); 00106 00107 Thread t(sd_thread, NULL, osPriorityNormal, (DEFAULT_STACK_SIZE * 2.25)); 00108 00109 while (true) { 00110 led2 = !led2; 00111 Thread::wait(1000); 00112 } 00113 }
Generated on Sun Jul 17 2022 08:25:26 by
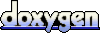