
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "test_env.h" 00003 #include "rtos.h" 00004 00005 #if defined(MBED_RTOS_SINGLE_THREAD) 00006 #error [NOT_SUPPORTED] test not supported 00007 #endif 00008 00009 /* 00010 * The stack size is defined in cmsis_os.h mainly dependent on the underlying toolchain and 00011 * the C standard library. For GCC, ARM_STD and IAR it is defined with a size of 2048 bytes 00012 * and for ARM_MICRO 512. Because of reduce RAM size some targets need a reduced stacksize. 00013 */ 00014 #if defined(TARGET_STM32F070RB) || defined(TARGET_STM32F072RB) 00015 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00016 #elif (defined(TARGET_EFM32HG_STK3400)) && !defined(TOOLCHAIN_ARM_MICRO) 00017 #define STACK_SIZE 512 00018 #elif (defined(TARGET_EFM32LG_STK3600) || defined(TARGET_EFM32WG_STK3800) || defined(TARGET_EFM32PG_STK3401)) && !defined(TOOLCHAIN_ARM_MICRO) 00019 #define STACK_SIZE 768 00020 #elif (defined(TARGET_EFM32GG_STK3700)) && !defined(TOOLCHAIN_ARM_MICRO) 00021 #define STACK_SIZE 1536 00022 #elif defined(TARGET_MCU_NRF51822) 00023 #define STACK_SIZE 768 00024 #else 00025 #define STACK_SIZE DEFAULT_STACK_SIZE 00026 #endif 00027 00028 void print_char(char c = '*') { 00029 printf("%c", c); 00030 fflush(stdout); 00031 } 00032 00033 DigitalOut led1(LED1); 00034 DigitalOut led2(LED2); 00035 00036 void led2_thread(void const *argument) { 00037 while (true) { 00038 led2 = !led2; 00039 Thread::wait(1000); 00040 print_char(); 00041 } 00042 } 00043 00044 int main() { 00045 MBED_HOSTTEST_TIMEOUT(15); 00046 MBED_HOSTTEST_SELECT(wait_us_auto); 00047 MBED_HOSTTEST_DESCRIPTION(Basic thread); 00048 MBED_HOSTTEST_START("RTOS_1"); 00049 00050 Thread thread(led2_thread, NULL, osPriorityNormal, STACK_SIZE); 00051 00052 while (true) { 00053 led1 = !led1; 00054 Thread::wait(500); 00055 } 00056 }
Generated on Sun Jul 17 2022 08:25:26 by
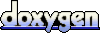