
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "cmsis_os.h" 00003 00004 #if defined(MBED_RTOS_SINGLE_THREAD) 00005 #error [NOT_SUPPORTED] test not supported 00006 #endif 00007 00008 typedef struct { 00009 float voltage; /* AD result of measured voltage */ 00010 float current; /* AD result of measured current */ 00011 uint32_t counter; /* A counter value */ 00012 } mail_t; 00013 00014 osMailQDef(mail_box, 16, mail_t); 00015 osMailQId mail_box; 00016 00017 void send_thread (void const *argument) { 00018 uint32_t i = 0; 00019 while (true) { 00020 i++; // fake data update 00021 mail_t *mail = (mail_t*)osMailAlloc(mail_box, osWaitForever); 00022 mail->voltage = (i * 0.1) * 33; 00023 mail->current = (i * 0.1) * 11; 00024 mail->counter = i; 00025 osMailPut(mail_box, mail); 00026 osDelay(1000); 00027 } 00028 } 00029 00030 osThreadDef(send_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00031 00032 int main (void) { 00033 mail_box = osMailCreate(osMailQ(mail_box), NULL); 00034 osThreadCreate(osThread(send_thread), NULL); 00035 00036 while (true) { 00037 osEvent evt = osMailGet(mail_box, osWaitForever); 00038 if (evt.status == osEventMail) { 00039 mail_t *mail = (mail_t*)evt.value.p; 00040 printf("\nVoltage: %.2f V\n\r" , mail->voltage); 00041 printf("Current: %.2f A\n\r" , mail->current); 00042 printf("Number of cycles: %u\n\r", mail->counter); 00043 00044 osMailFree(mail_box, mail); 00045 } 00046 } 00047 }
Generated on Sun Jul 17 2022 08:25:26 by
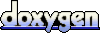