
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Interrupt table relocation test, based on the 'interrupt_in' test 00002 // It will test an interrupt pin before and after the interrupt table is relocated 00003 // Works only on LPC1768 00004 00005 #include "test_env.h" 00006 #include "cmsis_nvic.h" 00007 #include "mbed_toolchain.h" 00008 #include <string.h> 00009 00010 #if defined(TARGET_SAMR21G18A) || defined(TARGET_SAMD21J18A) || defined(TARGET_SAMD21G18A) 00011 #define PIN_IN (PB02) 00012 #define PIN_OUT (PB03) 00013 #define NUM_VECTORS (16+28) 00014 00015 #elif defined(TARGET_SAML21J18A) 00016 #define PIN_IN (PB00) 00017 #define PIN_OUT (PB01) 00018 #define NUM_VECTORS (16+29) 00019 00020 #elif defined(TARGET_SAMG55J19) 00021 #define PIN_IN (PA17) 00022 #define PIN_OUT (PA18) 00023 #define NUM_VECTORS (16+50) 00024 00025 #else 00026 #define PIN_IN (p5) 00027 #define PIN_OUT (p25) 00028 #define NUM_VECTORS (16+33) 00029 #endif 00030 00031 DigitalOut out(PIN_OUT); 00032 DigitalOut myled(LED1); 00033 00034 volatile int checks = 0; 00035 uint32_t int_table[NUM_VECTORS] MBED_ALIGN(256); 00036 00037 #define FALLING_EDGE_COUNT 5 00038 00039 void flipper() { 00040 for (int i = 0; i < FALLING_EDGE_COUNT; i++) { 00041 out = 1; 00042 wait(0.2); 00043 out = 0; 00044 wait(0.2); 00045 } 00046 } 00047 00048 void in_handler() { 00049 checks++; 00050 myled = !myled; 00051 } 00052 00053 static bool test_once() { 00054 InterruptIn in(PIN_IN); 00055 checks = 0; 00056 printf("Interrupt table location: 0x%08X\r\n", SCB->VTOR); 00057 in.rise(NULL); 00058 in.fall(in_handler); 00059 flipper(); 00060 in.fall(NULL); 00061 bool result = (checks == FALLING_EDGE_COUNT); 00062 printf("Falling edge checks counted: %d ... [%s]\r\n", checks, result ? "OK" : "FAIL"); 00063 return result; 00064 } 00065 00066 int main() { 00067 MBED_HOSTTEST_TIMEOUT(15); 00068 MBED_HOSTTEST_SELECT(default_auto); 00069 MBED_HOSTTEST_DESCRIPTION(Interrupt vector relocation); 00070 MBED_HOSTTEST_START("MBED_A18"); 00071 00072 // First test, no table reallocation 00073 { 00074 printf("Starting first test (interrupts not relocated).\r\n"); 00075 bool ret = test_once(); 00076 if (ret == false) { 00077 MBED_HOSTTEST_RESULT(false); 00078 } 00079 } 00080 00081 // Relocate interrupt table and test again 00082 { 00083 printf("Starting second test (interrupts relocated).\r\n"); 00084 memcpy(int_table, (void*)SCB->VTOR, sizeof(int_table)); 00085 SCB->VTOR = (uint32_t)int_table; 00086 00087 bool ret = test_once(); 00088 if (ret == false) { 00089 MBED_HOSTTEST_RESULT(false); 00090 } 00091 } 00092 00093 MBED_HOSTTEST_RESULT(true); 00094 }
Generated on Sun Jul 17 2022 08:25:26 by
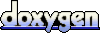