
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Ticker tick; 00004 DigitalOut led(LED1); 00005 00006 namespace { 00007 const int MS_INTERVALS = 1000; 00008 } 00009 00010 class TickerHandler { 00011 public: 00012 TickerHandler(const int _ms_intervals) : m_ticker_count(0), m_ms_intervals(_ms_intervals) { 00013 } 00014 00015 void print_char(char c = '*') 00016 { 00017 printf("%c", c); 00018 fflush(stdout); 00019 } 00020 00021 void togglePin(void) 00022 { 00023 if (ticker_count >= MS_INTERVALS) { 00024 print_char(); 00025 ticker_count = 0; 00026 led = !led; // Blink 00027 } 00028 ticker_count++; 00029 } 00030 00031 protected: 00032 int m_ticker_count; 00033 }; 00034 00035 int main() 00036 { 00037 TickerHandler th; 00038 00039 tick.attach_us(th, TickerHandler::togglePin, 1000); 00040 while (1); 00041 }
Generated on Sun Jul 17 2022 08:25:26 by
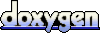