
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "test_env.h" 00002 #include "mbed.h" 00003 #include "spifi_rom_api.h" 00004 00005 __attribute__((section("SPIFI_MEM"))) const unsigned char cube_image[] = { 00006 137,80,78,71,13,10,26,10,0,0,0,13,73,72,68,82, 00007 0,0,0,150,0,0,0,200,8,2,0,0,0,133,231,143, 00008 50,0,0,0,1,115,82,71,66,0,174,206,28,233,0,0, 00009 0,4,103,65,77,65,0,0,177,143,11,252,97,5,0,0, 00010 0,9,112,72,89,115,0,0,14,195,0,0,14,195,1,199, 00011 111,168,100,0,0,156,193,73,68,65,84,120,94,236,253,103, 00012 124,27,233,125,54,140,14,48,24,0,51,24,244,94,9,246, 00013 0,0,0,0,73,69,78,68,174,66,96,130}; 00014 00015 int cube_image_sz = sizeof(cube_image); 00016 00017 const unsigned char cube_image_ref[] = { 00018 137,80,78,71,13,10,26,10,0,0,0,13,73,72,68,82, 00019 0,0,0,150,0,0,0,200,8,2,0,0,0,133,231,143, 00020 50,0,0,0,1,115,82,71,66,0,174,206,28,233,0,0, 00021 0,4,103,65,77,65,0,0,177,143,11,252,97,5,0,0, 00022 0,9,112,72,89,115,0,0,14,195,0,0,14,195,1,199, 00023 111,168,100,0,0,156,193,73,68,65,84,120,94,236,253,103, 00024 124,27,233,125,54,140,14,48,24,0,51,24,244,94,9,246, 00025 0,0,0,0,73,69,78,68,174,66,96,130}; 00026 00027 int cube_image_ref_sz = sizeof(cube_image_ref); 00028 00029 /* 00030 * The SPIFI_ROM_PTR (0x1FFF1FF8) points to an area where the pointers to 00031 * different drivers in ROM are stored. 00032 */ 00033 typedef struct { 00034 /*const*/ unsigned p_usbd; // USBROMD 00035 /*const*/ unsigned p_clib; 00036 /*const*/ unsigned p_cand; 00037 /*const*/ unsigned p_pwrd; // PWRROMD 00038 /*const*/ unsigned p_promd; // DIVROMD 00039 /*const*/ SPIFI_RTNS *pSPIFID; // SPIFIROMD 00040 /*const*/ unsigned p_dev3; 00041 /*const*/ unsigned p_dev4; 00042 } ROM; 00043 00044 #define ROM_DRIVERS_PTR ((ROM *)(*((unsigned int *)SPIFI_ROM_PTR))) 00045 #define IS_ADDR_IN_SPIFI(__addr) ( (((uint32_t)(__addr)) & 0xff000000) == SPIFI_MEM_BASE ) 00046 #define IS_ADDR_IN_IFLASH(__addr) ( (((uint32_t)(__addr)) & 0xff000000) == 0x10000000 ) 00047 00048 static void initialize_spifi(void) 00049 { 00050 SPIFIobj* obj = (SPIFIobj*)malloc(sizeof(SPIFIobj)); 00051 if (obj == NULL) { 00052 // Failed to allocate memory for ROM data 00053 notify_completion(false); 00054 } 00055 00056 // Turn on SPIFI block as it is disabled on reset 00057 LPC_SC->PCONP |= 0x00010000; 00058 00059 // pinsel for SPIFI 00060 LPC_IOCON->P2_7 = 5; /* SPIFI_CSN @ P2.7 */ 00061 LPC_IOCON->P0_22 = 5; /* SPIFI_CLK @ P0.22 */ 00062 LPC_IOCON->P0_15 = 5; /* SPIFI_IO2 @ P0.15 */ 00063 LPC_IOCON->P0_16 = 5; /* SPIFI_IO3 @ P0.16 */ 00064 LPC_IOCON->P0_17 = 5; /* SPIFI_IO1 @ P0.17 */ 00065 LPC_IOCON->P0_18 = 5; /* SPIFI_IO0 @ P0.18 */ 00066 00067 uint32_t spifi_clk_div = (*((volatile uint32_t*)0x400FC1B4)) & 0x1f; 00068 uint32_t spifi_clk_mhz = (SystemCoreClock / spifi_clk_div) / 1000000; 00069 00070 const SPIFI_RTNS* _spifi = ROM_DRIVERS_PTR->pSPIFID; 00071 00072 /* Typical time tCS is 20 ns min, we give 200 ns to be on safer side */ 00073 int rc = _spifi->spifi_init (obj, spifi_clk_mhz/5, S_FULLCLK+S_RCVCLK, spifi_clk_mhz); 00074 if (rc) { 00075 // Failed to initialize SPIFI 00076 notify_completion(false); 00077 } 00078 } 00079 00080 int main() { 00081 00082 initialize_spifi(); 00083 00084 // Make sure that cube_image is placed in SPIFI 00085 if (!IS_ADDR_IN_SPIFI(cube_image)) { 00086 notify_completion(false); 00087 } 00088 00089 // Make sure that cube_image_ref is in IFLASH 00090 if (IS_ADDR_IN_SPIFI(cube_image_ref)) { 00091 notify_completion(false); 00092 } 00093 00094 // Compare content 00095 if (cube_image_sz != cube_image_ref_sz) { 00096 notify_completion(false); 00097 } else { 00098 int i = 0; 00099 for (; i < cube_image_sz; i++) { 00100 if (cube_image[i] != cube_image_ref[i]) { 00101 notify_completion(false); 00102 } 00103 } 00104 } 00105 00106 notify_completion(true); 00107 }
Generated on Sun Jul 17 2022 08:25:26 by
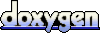