
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); 00004 00005 #if defined(TARGET_LPC4088) 00006 Serial uart(P4_22, P4_23); 00007 #elif defined(TARGET_MAXWSNENV) 00008 Serial uart(P0_1, P0_0); 00009 #elif defined(TARGET_SAMR21G18A) || defined(TARGET_SAMD21J18A) || defined(TARGET_SAMD21G18A) 00010 Serial uart(PA16, PA17); 00011 #elif defined(TARGET_SAMG55J19) 00012 Serial uart(PB10, PB11); 00013 #else 00014 Serial uart(p9, p10); 00015 #endif 00016 00017 DigitalOut led1(LED1); 00018 DigitalOut led2(LED2); 00019 00020 // This function is called when a character goes into the RX buffer. 00021 void rxCallback(void) { 00022 led1 = !led1; 00023 pc.putc(uart.getc()); 00024 } 00025 00026 00027 int main() { 00028 // Use a deliberatly slow baud to fill up the TX buffer 00029 uart.baud(1200); 00030 uart.attach(&rxCallback, Serial::RxIrq); 00031 00032 printf("Starting test loop:\n"); 00033 wait(1); 00034 00035 int c = 'A'; 00036 for (int loop = 0; loop < 512; loop++) { 00037 uart.printf("%c", c); 00038 c++; 00039 if (c > 'Z') c = 'A'; 00040 } 00041 00042 while (true) { 00043 led2 = !led2; 00044 wait(1); 00045 } 00046 }
Generated on Sun Jul 17 2022 08:25:26 by
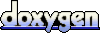