
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #if !DEVICE_PORTOUT 00004 #error [NOT_SUPPORTED] PortOut is not supported 00005 #endif 00006 00007 # if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) || defined(TARGET_LPC4088) || defined(TARGET_LPC2460) 00008 # define LED_PORT Port1 00009 # define LED1 (1 << 18) // P1.18 00010 # define LED2 (1 << 20) // P1.20 00011 # define LED3 (1 << 21) // P1.21 00012 # define LED4 (1 << 23) // P1.23 00013 # elif defined(TARGET_LPC11U24) || defined(TARGET_LPC1114) 00014 # define LED_PORT Port1 00015 # define LED1 (1 << 8) // P1.8 00016 # define LED2 (1 << 9) // P1.9 00017 # define LED3 (1 << 10) // P1.10 00018 # define LED4 (1 << 11) // P1.11 00019 # elif defined(TARGET_MAXWSNENV) 00020 # define LED_PORT Port1 00021 # define LED1 (1 << 4) // P1.4 00022 # define LED2 (1 << 6) // P1.6 00023 # define LED3 (1 << 7) // P1.7 00024 # define LED4 0 00025 # elif defined(TARGET_MAX32600MBED) 00026 # define LED_PORT Port7 00027 # define LED1 (1 << 0) // P7.0 00028 # define LED2 (1 << 6) // P7.6 00029 # define LED3 (1 << 4) // P7.4 00030 # define LED4 0 00031 # elif defined(TARGET_SAMR21G18A) 00032 # define LED_PORT PortA 00033 # define LED1 (1 << 19) /*PA19*/ 00034 # define LED2 0 00035 # define LED3 0 00036 # define LED4 0 00037 # elif defined(TARGET_SAMD21J18A) 00038 # define LED_PORT PortB 00039 # define LED1 (1 << 30) /*PB30*/ 00040 # define LED2 0 00041 # define LED3 0 00042 # define LED4 0 00043 # elif defined(TARGET_SAMD21G18A) 00044 # define LED_PORT PortA 00045 # define LED1 (1 << 23) /*PA23*/ 00046 # define LED2 0 00047 # define LED3 0 00048 # define LED4 0 00049 # elif defined(TARGET_SAML21J18A) 00050 # define LED_PORT PortB 00051 # define LED1 (1 << 10) /*PB10*/ 00052 # define LED2 0 00053 # define LED3 0 00054 # define LED4 0 00055 # elif defined(TARGET_SAMG55J19) 00056 # define LED_PORT PortA 00057 # define LED1 (1 << 6) /*PA06*/ 00058 # define LED2 0 00059 # define LED3 0 00060 # define LED4 0 00061 # else 00062 #error [NOT_SUPPORTED] This test can't be ran on this target 00063 #endif 00064 00065 00066 #define LED_MASK (LED1|LED2|LED3|LED4) 00067 00068 int mask[4] = { 00069 (LED1 | LED3), 00070 (LED2 | LED4), 00071 (LED1 | LED2), 00072 (LED3 | LED4) 00073 }; 00074 00075 PortOut ledport(LED_PORT, LED_MASK); 00076 00077 int main() { 00078 while (1) { 00079 for (int i=0; i<4; i++) { 00080 ledport = mask[i]; 00081 wait(1); 00082 } 00083 } 00084 }
Generated on Sun Jul 17 2022 08:25:26 by
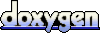