
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "test_env.h" 00002 00003 #if !DEVICE_PORTINOUT 00004 #error [NOT_SUPPORTED] PortInOut is not supported 00005 #endif 00006 00007 #if defined(TARGET_K64F) 00008 #define P1_1 (1 << 16) 00009 #define P1_2 (1 << 17) 00010 #define PORT_1 PortC 00011 00012 #define P2_1 (1 << 2) 00013 #define P2_2 (1 << 3) 00014 #define PORT_2 PortC 00015 00016 #elif defined(TARGET_K66F) 00017 #define P1_1 (1 << 3) 00018 #define P1_2 (1 << 4) 00019 #define PORT_1 PortC 00020 00021 #define P2_1 (1 << 5) 00022 #define P2_2 (1 << 2) 00023 #define PORT_2 PortC 00024 00025 #elif defined(TARGET_LPC11U24) 00026 #define P1_1 (1 << 9) // p0.9 00027 #define P1_2 (1 << 8) // p0.8 00028 #define PORT_1 Port0 00029 00030 #define P2_1 (1 << 24) // p1.24 00031 #define P2_2 (1 << 25) // p1.25 00032 #define PORT_2 Port1 00033 00034 #elif defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00035 #define P1_1 (1 << 9) // p0.9 -> p5 00036 #define P1_2 (1 << 8) // p0.8 -> p6 00037 #define PORT_1 Port0 00038 00039 #define P2_1 (1 << 1) // p2.1 -> p25 00040 #define P2_2 (1 << 0) // p2.0 -> p26 00041 #define PORT_2 Port2 00042 00043 #elif defined(TARGET_LPC4088) 00044 #define P1_1 (1 << 7) // p0.7 -> p13 00045 #define P1_2 (1 << 6) // p0.6 -> p14 00046 #define PORT_1 Port0 00047 00048 #define P2_1 (1 << 2) // p1.2 -> p30 00049 #define P2_2 (1 << 3) // p1.3 -> p29 00050 #define PORT_2 Port1 00051 00052 #elif defined(TARGET_LPC1114) 00053 #define P1_1 (1 << 9) // p0.9 00054 #define P1_2 (1 << 8) // p0.8 00055 #define PORT_1 Port0 00056 00057 #define P2_1 (1 << 1) // p1.1 00058 #define P2_2 (1 << 0) // p1.0 00059 #define PORT_2 Port1 00060 00061 #elif defined(TARGET_KL25Z) 00062 #define P1_1 (1 << 4) // PTA4 00063 #define P1_2 (1 << 5) // PTA5 00064 #define PORT_1 PortA 00065 00066 #define P2_1 (1 << 5) // PTC5 00067 #define P2_2 (1 << 6) // PTC6 00068 #define PORT_2 PortC 00069 00070 #elif defined(TARGET_nRF51822) 00071 #define P1_1 (1 << 4) // p4 00072 #define P1_2 (1 << 5) // p5 00073 #define PORT_1 Port0 00074 00075 #define P2_1 (1 << 24) // p24 00076 #define P2_2 (1 << 25) // p25 00077 #define PORT_2 Port0 00078 00079 #elif defined(TARGET_MAXWSNENV) 00080 #define P1_1 (1 << 0) 00081 #define P1_2 (1 << 1) 00082 #define PORT_1 Port0 00083 00084 #define P2_1 (1 << 0) 00085 #define P2_2 (1 << 1) 00086 #define PORT_2 Port1 00087 00088 #elif defined(TARGET_MAX32600MBED) 00089 #define P1_1 (1 << 0) // P1_0 00090 #define P1_2 (1 << 1) // P1_1 00091 #define PORT_1 Port1 00092 00093 #define P2_1 (1 << 7) // P4_7 00094 #define P2_2 (1 << 6) // P4_6 00095 #define PORT_2 Port4 00096 00097 #elif defined(TARGET_NUCLEO_F030R8) || \ 00098 defined(TARGET_NUCLEO_F070RB) || \ 00099 defined(TARGET_NUCLEO_F072RB) || \ 00100 defined(TARGET_NUCLEO_F091RC) || \ 00101 defined(TARGET_NUCLEO_F103RB) || \ 00102 defined(TARGET_NUCLEO_F302R8) || \ 00103 defined(TARGET_NUCLEO_F303RE) || \ 00104 defined(TARGET_NUCLEO_F334R8) || \ 00105 defined(TARGET_NUCLEO_F401RE) || \ 00106 defined(TARGET_NUCLEO_F410RB) || \ 00107 defined(TARGET_NUCLEO_F411RE) || \ 00108 defined(TARGET_NUCLEO_L053R8) || \ 00109 defined(TARGET_NUCLEO_L073RZ) || \ 00110 defined(TARGET_NUCLEO_L476RG) || \ 00111 defined(TARGET_NUCLEO_F446RE) || \ 00112 defined(TARGET_NUCLEO_L152RE) 00113 #define P1_1 (1 << 3) // PB_3 (D3) 00114 #define P1_2 (1 << 10) // PB_10 (D6) 00115 #define PORT_1 PortB 00116 00117 #define P2_1 (1 << 10) // PA_10 (D2) 00118 #define P2_2 (1 << 8) // PA_8 (D7) 00119 #define PORT_2 PortA 00120 00121 #elif defined(TARGET_NUCLEO_F767ZI) || \ 00122 defined(TARGET_NUCLEO_F303ZE) || \ 00123 defined(TARGET_NUCLEO_F207ZG) || \ 00124 defined(TARGET_NUCLEO_F746ZG) 00125 #define P1_1 (1 << 13) // PE_13 (D3) 00126 #define P1_2 (1 << 11) // PE_11 (D5) 00127 #define PORT_1 PortE 00128 00129 #define P2_1 (1 << 15) // PF_15 (D2) 00130 #define P2_2 (1 << 14) // PF_14 (D4) 00131 #define PORT_2 PortF 00132 00133 #elif defined(TARGET_EFM32LG_STK3600) || defined(TARGET_EFM32GG_STK3700) || defined(TARGET_EFM32WG_STK3800) 00134 #define P1_1 (1 << 0) // PD0 00135 #define P1_2 (1 << 1) // PD1 00136 #define PORT_1 PortD 00137 00138 #define P2_1 (1 << 3) // PC3 00139 #define P2_2 (1 << 4) // PC4 00140 #define PORT_2 PortC 00141 00142 #elif defined(TARGET_EFM32ZG_STK3200) 00143 #define P1_1 (1 << 7) // PD7 00144 #define P1_2 (1 << 6) // PD6 00145 #define PORT_1 PortD 00146 00147 #define P2_1 (1 << 1) // PC1 00148 #define P2_2 (1 << 2) // PC2 00149 #define PORT_2 PortC 00150 00151 #elif defined(TARGET_EFM32HG_STK3400) 00152 #define P1_1 (1 << 10) // PE10 00153 #define P1_2 (1 << 11) // PE11 00154 #define PORT_1 PortE 00155 00156 #define P2_1 (1 << 1) // PC1 00157 #define P2_2 (1 << 2) // PC2 00158 #define PORT_2 PortC 00159 00160 #elif defined(TARGET_EFM32PG_STK3401) 00161 #define P1_1 (1 << 6) // PC6 00162 #define P1_2 (1 << 7) // PC7 00163 #define PORT_1 PortC 00164 00165 #define P2_1 (1 << 3) // PA3 00166 #define P2_2 (1 << 4) // PA4 00167 #define PORT_2 PortA 00168 00169 #elif defined(TARGET_SAMR21G18A) || defined(TARGET_SAMD21J18A) 00170 #define P1_1 (1 << 6) /*PA06*/ 00171 #define P1_2 (1 << 7) /*PA07*/ 00172 #define PORT_1 PortA 00173 00174 #define P2_1 (1 << 2) /*PB02*/ 00175 #define P2_2 (1 << 3) /*PB03*/ 00176 #define PORT_2 PortB 00177 00178 #elif defined(TARGET_SAMD21G18A) 00179 #define P1_1 (1 << 2) /*PA02*/ 00180 #define P1_2 (1 << 3) /*PA03*/ 00181 #define PORT_1 PortA 00182 00183 #define P2_1 (1 << 2) /*PB02*/ 00184 #define P2_2 (1 << 3) /*PB03*/ 00185 #define PORT_2 PortB 00186 00187 #elif defined(TARGET_SAML21J18A) 00188 #define P1_1 (1 << 4) /*PA04*/ 00189 #define P1_2 (1 << 5) /*PA05*/ 00190 #define PORT_1 PortA 00191 00192 #define P2_1 (1 << 0) /*PB00*/ 00193 #define P2_2 (1 << 1) /*PB01*/ 00194 #define PORT_2 PortB 00195 00196 #elif defined(TARGET_SAMG55J19) 00197 #define P1_1 (1 << 17) /*PA17*/ 00198 #define P1_2 (1 << 18) /*PA18*/ 00199 #define PORT_1 PortA 00200 00201 #define P2_1 (1 << 10) /*PB10*/ 00202 #define P2_2 (1 << 11) /*PB11*/ 00203 #define PORT_2 PortB 00204 00205 #elif defined(TARGET_VK_RZ_A1H) 00206 #define P1_1 (1 << 2) /*P3_2*/ 00207 #define P1_2 (1 << 7) /*P3_7*/ 00208 #define PORT_1 Port3 00209 00210 #define P2_1 (1 << 6) /*P5_6*/ 00211 #define P2_2 (1 << 1) /*P5_1*/ 00212 #define PORT_2 Port5 00213 00214 #else 00215 #error [NOT_SUPPORTED] This test is not defined on this target 00216 #endif 00217 00218 #define MASK_1 (P1_1 | P1_2) 00219 #define MASK_2 (P2_1 | P2_2) 00220 00221 PortInOut port1(PORT_1, MASK_1); 00222 PortInOut port2(PORT_2, MASK_2); 00223 00224 int main() { 00225 MBED_HOSTTEST_TIMEOUT(20); 00226 MBED_HOSTTEST_SELECT(default_auto); 00227 MBED_HOSTTEST_DESCRIPTION(PortInOut); 00228 MBED_HOSTTEST_START("MBED_A11"); 00229 00230 bool check = true; 00231 00232 port1.output(); 00233 port2.input(); 00234 00235 port1 = MASK_1; wait(0.1); 00236 if (port2 != MASK_2) check = false; 00237 00238 port1 = 0; wait(0.1); 00239 if (port2 != 0) check = false; 00240 00241 port1.input(); 00242 port2.output(); 00243 00244 port2 = MASK_2; wait(0.1); 00245 if (port1 != MASK_1) check = false; 00246 00247 port2 = 0; wait(0.1); 00248 if (port1 != 0) check = false; 00249 00250 MBED_HOSTTEST_RESULT(check); 00251 }
Generated on Sun Jul 17 2022 08:25:26 by
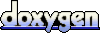