
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * To run this test program, link p9 to p10 so the Serial loops 00003 * back and receives characters it sends. 00004 */ 00005 #include "mbed.h" 00006 #include "MODSERIAL.h" 00007 00008 DigitalOut led1(LED1); 00009 DigitalOut led2(LED2); 00010 DigitalOut led3(LED3); 00011 DigitalOut led4(LED4); 00012 00013 MODSERIAL pc(USBTX, USBRX); 00014 00015 /* 00016 * As experiement, you can define MODSERIAL as show here and see what 00017 * effects it has on the LEDs. 00018 * 00019 * MODSERIAL uart(TX_PIN, RX_PIN, 512); 00020 * With this, the 512 characters sent can straight into the buffer 00021 * vary quickly. This means LED1 is only on briefly as the TX buffer 00022 * fills. 00023 * 00024 * MODSERIAL uart(TX_PIN, RX_PIN, 32); 00025 * With this, the buffer is smaller than the default 256 bytes and 00026 * therefore LED1 stays on much longer while the system waits for 00027 * room in the TX buffer. 00028 */ 00029 MODSERIAL uart(p9, p10); 00030 00031 // This function is called when a character goes from the TX buffer 00032 // to the Uart THR FIFO register. 00033 void txCallback(MODSERIAL_IRQ_INFO *q) { 00034 led2 = !led2; 00035 } 00036 00037 // This function is called when TX buffer goes empty 00038 void txEmpty(MODSERIAL_IRQ_INFO *q) { 00039 led2 = 0; 00040 pc.puts(" Done. "); 00041 } 00042 00043 // This function is called when a character goes into the RX buffer. 00044 void rxCallback(MODSERIAL_IRQ_INFO *q) { 00045 led3 = !led3; 00046 pc.putc(uart.getc()); 00047 } 00048 00049 int main() { 00050 int c = 'A'; 00051 00052 // Ensure the baud rate for the PC "USB" serial is much 00053 // higher than "uart" baud rate below. (default: 9600) 00054 // pc.baud(9600); 00055 00056 // Use a deliberatly slow baud to fill up the TX buffer 00057 uart.baud(1200); 00058 00059 uart.attach(&txCallback, MODSERIAL::ModTxIrq); 00060 uart.attach(&rxCallback, MODSERIAL::ModRxIrq); 00061 uart.attach(&txEmpty, MODSERIAL::ModTxEmpty); 00062 00063 // Loop sending characters. We send 512 00064 // which is twice the default TX/RX buffer size. 00065 00066 led1 = 1; // Show start of sending with LED1. 00067 00068 for (int loop = 0; loop < 512; loop++) { 00069 uart.printf("%c", c); 00070 c++; 00071 if (c > 'Z') c = 'A'; 00072 } 00073 00074 led1 = 0; // Show the end of sending by switching off LED1. 00075 00076 // End program. Flash LED4. Notice how LED 2 and 3 continue 00077 // to flash for a short period while the interrupt system 00078 // continues to send the characters left in the TX buffer. 00079 00080 while(1) { 00081 led4 = !led4; 00082 wait(0.25); 00083 } 00084 } 00085 00086 /* 00087 * Notes. Here is the sort of output you can expect on your PC/Mac/Linux host 00088 * machine that is connected to the "pc" USB serial port. 00089 * 00090 * ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUV 00091 * WXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQR 00092 * STUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMN 00093 * OPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJ 00094 * KLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEF 00095 * GHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZAB 00096 * CDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQ Done. R 00097 * 00098 * Of interest is that last "R" character after the system has said "Done." 00099 * This comes from the fact that the TxEmpty callback is made when the TX buffer 00100 * becomes empty. MODSERIAL makes use of the fact that the Uarts built into the 00101 * LPC17xx device use a 16 byte FIFO on both RX and TX channels. This means that 00102 * when the TxEmpty callback is made, the TX buffer is empty, but that just means 00103 * the "last few characters" were written to the TX FIFO. So although the TX 00104 * buffer has gone empty, the Uart's transmit system is still sending any remaining 00105 * characters from it's TX FIFO. If you want to be truely sure all the characters 00106 * you have sent have left the Mbed then call txIsBusy(); This function will 00107 * return true if characters are still being sent. If it returns false after 00108 * the Tx buffer is empty then all your characters have been sent. 00109 * 00110 * In a similar way, when characters are received into the RX FIFO, the entire 00111 * FIFO contents is moved to the RX buffer, assuming there is room left in the 00112 * RX buffer. If there is not, any remaining characters are left in the RX FIFO 00113 * and will be moved to the RX buffer on the next interrupt or when the running 00114 * program removes a character(s) from the RX buffer with the getc() method. 00115 */
Generated on Sun Jul 17 2022 08:25:26 by
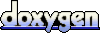