
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #if !DEVICE_LOCALFILESYSTEM 00004 #error [NOT_SUPPORTED] LocalFileSystem not supported 00005 #endif 00006 00007 void led_blink(PinName led) { 00008 DigitalOut myled(led); 00009 while (1) { 00010 myled = !myled; 00011 wait(1.0); 00012 } 00013 } 00014 00015 void notify_completion(bool success) { 00016 if (success) { 00017 printf("{success}\n"); 00018 } else { 00019 printf("{failure}\n"); 00020 } 00021 00022 printf("{end}\n"); 00023 led_blink(success ? LED1 : LED4); 00024 } 00025 00026 #define TEST_STRING "Hello World!" 00027 00028 FILE* test_open(char* path, const char* mode) { 00029 FILE *f; 00030 f = fopen(path, mode); 00031 if (f == NULL) { 00032 printf("Error opening file\n"); 00033 notify_completion(false); 00034 } 00035 00036 return f; 00037 } 00038 00039 void test_write(FILE* f, const char* str) { 00040 int n = fprintf(f, str); 00041 if (n != strlen(str)) { 00042 printf("Error writing file\n"); 00043 notify_completion(false); 00044 } 00045 } 00046 00047 void test_close(FILE* f) { 00048 int rc = fclose(f); 00049 if (rc != 0) { 00050 printf("Error closing file\n"); 00051 notify_completion(false); 00052 } 00053 } 00054 00055 int main() { 00056 LocalFileSystem local("local"); 00057 00058 FILE *f; 00059 char* str = TEST_STRING; 00060 char* buffer = (char*) malloc(sizeof(unsigned char)*strlen(TEST_STRING)); 00061 int str_len = strlen(TEST_STRING); 00062 00063 printf("Write files\n"); 00064 char filename[32]; 00065 for (int i=0; i<10; i++) { 00066 sprintf(filename, "/local/test_%d.txt", i); 00067 printf("Creating file: %s\n", filename); 00068 f = test_open(filename, "w"); 00069 test_write(f, str); 00070 test_close(f); 00071 } 00072 00073 printf("List files:\n"); 00074 DIR *d = opendir("/local"); 00075 struct dirent *p; 00076 while((p = readdir(d)) != NULL) { 00077 printf("%s\n", p->d_name); 00078 } 00079 closedir(d); 00080 00081 notify_completion(true); 00082 }
Generated on Sun Jul 17 2022 08:25:26 by
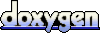