
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "test_env.h" 00003 00004 #if defined(TARGET_VK_RZ_A1H) 00005 #define LOOPCNT 1 00006 namespace { 00007 BusOut bus_out(LED1); 00008 PinName led_pins[1] = {LED1}; // Temp, used to map pins in bus_out 00009 } 00010 #elif defined(TARGET_DISCO_F429ZI) 00011 #define LOOPCNT 2 00012 namespace { 00013 BusOut bus_out(LED1, LED2); 00014 PinName led_pins[2] = {LED1, LED2}; // Temp, used to map pins in bus_out 00015 } 00016 #else 00017 #define LOOPCNT 4 00018 namespace { 00019 BusOut bus_out(LED1, LED2, LED3, LED4); 00020 PinName led_pins[4] = {LED1, LED2, LED3, LED4}; // Temp, used to map pins in bus_out 00021 } 00022 #endif 00023 00024 int main() 00025 { 00026 notify_start(); 00027 00028 bool result = false; 00029 00030 for (;;) { 00031 const int mask = bus_out.mask(); 00032 int led_mask = 0x00; 00033 if (LED1 != NC) led_mask |= 0x01; 00034 #if !defined(TARGET_VK_RZ_A1H) 00035 if (LED2 != NC) led_mask |= 0x02; 00036 #if !defined(TARGET_DISCO_F429ZI) 00037 if (LED3 != NC) led_mask |= 0x04; 00038 if (LED4 != NC) led_mask |= 0x08; 00039 #endif 00040 #endif 00041 00042 printf("MBED: BusIn mask: 0x%X\r\n", mask); 00043 printf("MBED: BusIn LED mask: 0x%X\r\n", led_mask); 00044 00045 // Let's check bus's connected pins mask 00046 if (mask != led_mask) { 00047 break; 00048 } 00049 00050 // Checking if DigitalOut is correctly set as connected 00051 for (int i=0; i < LOOPCNT; i++) { 00052 printf("MBED: BusOut.bit[%d] is %s\r\n", 00053 i, 00054 (led_pins[i] != NC && bus_out[i].is_connected()) 00055 ? "connected" 00056 : "not connected"); 00057 } 00058 00059 for (int i=0; i < LOOPCNT; i++) { 00060 if (led_pins[i] != NC && bus_out[0].is_connected() == 0) { 00061 break; 00062 } 00063 } 00064 00065 // Write mask all LEDs 00066 bus_out.write(mask); // Set all LED's pins in high state 00067 if (bus_out.read() != mask) { 00068 break; 00069 } 00070 // Zero all LEDs and see if mask is correctly cleared on all bits 00071 bus_out.write(~mask); 00072 if (bus_out.read() != 0x00) { 00073 break; 00074 } 00075 00076 result = true; 00077 break; 00078 } 00079 00080 printf("MBED: Blinking LEDs: \r\n"); 00081 00082 // Just a quick LED blinking... 00083 for (int i=0; i<LOOPCNT; i++) { 00084 if (led_pins[i] != NC && bus_out[i].is_connected()) { 00085 bus_out[i] = 1; 00086 printf("%c", 'A' + i); 00087 } else { 00088 printf("."); 00089 } 00090 wait(0.2); 00091 if (led_pins[i] != NC && bus_out[i].is_connected()) { 00092 bus_out[i] = 0; 00093 printf("%c", 'a' + i); 00094 } else { 00095 printf("."); 00096 } 00097 } 00098 printf("\r\n"); 00099 00100 notify_completion(result); 00101 }
Generated on Sun Jul 17 2022 08:25:26 by
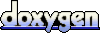