
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "mbed-mesh-api/Mesh6LoWPAN_ND.h" 00019 #include "mbed-mesh-api/MeshThread.h" 00020 #include "mbed-mesh-api/MeshInterfaceFactory.h" 00021 #include "atmel-rf-driver/driverRFPhy.h" // rf_device_register 00022 #include "test_cases.h" 00023 #include "mbed-drivers/test_env.h" 00024 #include "core-util/FunctionPointer.h" 00025 #define HAVE_DEBUG 1 00026 #include "ns_trace.h" 00027 00028 #define TRACE_GROUP "main" // for traces 00029 00030 using namespace mbed::util; 00031 00032 /** 00033 * Test with 6LoWPAN ND or Thread bootstrap 00034 * If TEST_THREAD_BOOTSTRAP is not defined then 6LoWPAN ND bootstrap is used. 00035 */ 00036 #define TEST_THREAD_BOOTSTRAP 00037 00038 class TestMeshApi; 00039 TestMeshApi *testMeshApi; 00040 00041 static int8_t rf_device_id = -1; 00042 00043 class TestMeshApi 00044 { 00045 private: 00046 int testId; 00047 int tests_pass; 00048 int loopCount; 00049 00050 public: 00051 TestMeshApi() : testId(0), tests_pass(1), loopCount(0) { 00052 tr_info("TestMeshApi"); 00053 } 00054 00055 void runTests() { 00056 switch (testId) { 00057 case 0: 00058 test_mesh_api_init(rf_device_id); 00059 break; 00060 case 1: 00061 test_mesh_api_init_thread(rf_device_id); 00062 break; 00063 case 2: 00064 test_mesh_api_disconnect(rf_device_id, MESH_TYPE_6LOWPAN_ND); 00065 break; 00066 case 3: 00067 test_mesh_api_disconnect(rf_device_id, MESH_TYPE_THREAD); 00068 break; 00069 #ifndef TEST_THREAD_BOOTSTRAP 00070 case 4: 00071 test_mesh_api_connect(rf_device_id, MESH_TYPE_6LOWPAN_ND); 00072 break; 00073 case 5: 00074 test_mesh_api_delete_connected(rf_device_id, MESH_TYPE_6LOWPAN_ND); 00075 break; 00076 case 6: 00077 test_mesh_api_connect_disconnect(rf_device_id, MESH_TYPE_6LOWPAN_ND); 00078 break; 00079 #else // TEST_THREAD_BOOTSTRAP 00080 case 4: 00081 test_mesh_api_connect_disconnect(rf_device_id, MESH_TYPE_THREAD); 00082 break; 00083 case 5: 00084 test_mesh_api_delete_connected(rf_device_id, MESH_TYPE_THREAD); 00085 break; 00086 case 6: 00087 test_mesh_api_connect(rf_device_id, MESH_TYPE_THREAD); 00088 break; 00089 case 7: 00090 test_mesh_api_data_poll_rate_set_thread(rf_device_id); 00091 break; 00092 #endif // TEST_THREAD_BOOTSTRAP 00093 default: 00094 endTest(tests_pass); 00095 break; 00096 } 00097 00098 testId++; 00099 } 00100 00101 void testResult(int status) { 00102 tests_pass = tests_pass && status; 00103 } 00104 00105 void endTest(bool status) { 00106 if (loopCount > 0) { 00107 printf("\r\n#####\r\n"); 00108 printf("\r\nTest loops left %d, current result=%d\r\n", loopCount, tests_pass); 00109 printf("\r\n#####\r\n"); 00110 loopCount--; 00111 testId = 0; 00112 /* Schedule tests run again */ 00113 FunctionPointer0<void> fpNextTest(testMeshApi, &TestMeshApi::runTests); 00114 minar::Scheduler::postCallback(fpNextTest.bind()).delay(minar::milliseconds(3000)); 00115 } else { 00116 MBED_HOSTTEST_RESULT(status); 00117 } 00118 } 00119 }; 00120 00121 00122 void test_result_notify(int result, AbstractMesh *meshAPI) 00123 { 00124 if (meshAPI != NULL) { 00125 delete meshAPI; 00126 } 00127 /* inform results to main class */ 00128 FunctionPointer1<void, int> fpResult(testMeshApi, &TestMeshApi::testResult); 00129 minar::Scheduler::postCallback(fpResult.bind(result)); 00130 00131 /* Schedule next test to run */ 00132 FunctionPointer0<void> fpNextTest(testMeshApi, &TestMeshApi::runTests); 00133 minar::Scheduler::postCallback(fpNextTest.bind()).delay(minar::milliseconds(1000)); 00134 } 00135 00136 00137 void app_start(int, char **) 00138 { 00139 MBED_HOSTTEST_TIMEOUT(5); 00140 MBED_HOSTTEST_SELECT(default); 00141 MBED_HOSTTEST_DESCRIPTION(Mesh network API tests); 00142 MBED_HOSTTEST_START("mbed-mesh-api"); 00143 00144 // set tracing baud rate 00145 static Serial &pc = get_stdio_serial(); 00146 pc.baud(115200); 00147 00148 // before registering RF device mesh network needs to be created 00149 Mesh6LoWPAN_ND *mesh_api = (Mesh6LoWPAN_ND *)MeshInterfaceFactory::createInterface(MESH_TYPE_6LOWPAN_ND); 00150 delete mesh_api; 00151 rf_device_id = rf_device_register(); 00152 00153 testMeshApi = new TestMeshApi; 00154 00155 FunctionPointer0<void> fp(testMeshApi, &TestMeshApi::runTests); 00156 minar::Scheduler::postCallback(fp.bind()); 00157 }
Generated on Sun Jul 17 2022 08:25:26 by
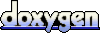