
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <stdio.h> 00018 #include "mbed-drivers/test_env.h" 00019 #include "atmel-rf-driver/driverRFPhy.h" // rf_device_register 00020 #include "mbed-mesh-api/MeshInterfaceFactory.h" 00021 #include "mbed-mesh-api/Mesh6LoWPAN_ND.h" 00022 #include "mbed-mesh-api/MeshThread.h" 00023 // For tracing we need to define flag, have include and define group 00024 #define HAVE_DEBUG 1 00025 #include "ns_trace.h" 00026 #define TRACE_GROUP "mesh_appl" 00027 00028 // Bootstrap mode, if undefined then 6LoWPAN-ND mode is used 00029 #define BOOTSTRAP_MODE_THREAD 00030 00031 // mesh network state 00032 static mesh_connection_status_t mesh_network_state = MESH_DISCONNECTED; 00033 00034 static AbstractMesh *meshApi; 00035 00036 // mesh network callback, called when network state changes 00037 void mesh_network_callback(mesh_connection_status_t mesh_state) 00038 { 00039 tr_info("mesh_network_callback() %d", mesh_state); 00040 mesh_network_state = mesh_state; 00041 if (mesh_network_state == MESH_CONNECTED) { 00042 tr_info("Connected to mesh network!"); 00043 // Once connected, disconnect from network 00044 meshApi->disconnect(); 00045 } else if (mesh_network_state == MESH_DISCONNECTED) { 00046 tr_info("Disconnected from mesh network!"); 00047 delete meshApi; 00048 tr_info("Mesh networking done!"); 00049 } else { 00050 tr_error("Networking error!"); 00051 } 00052 } 00053 00054 void app_start(int, char **) 00055 { 00056 mesh_error_t status; 00057 00058 // set tracing baud rate 00059 static Serial &pc = get_stdio_serial(); 00060 pc.baud(115200); 00061 00062 // create 6LoWPAN_ND interface 00063 #ifdef BOOTSTRAP_MODE_THREAD 00064 meshApi = MeshInterfaceFactory::createInterface(MESH_TYPE_THREAD); 00065 uint8_t eui64[8]; 00066 int8_t rf_device = rf_device_register(); 00067 rf_read_mac_address(eui64); 00068 char *pskd = (char *)"Secret password"; 00069 // Use tr_info traces and RF interface after MeshAPi has been created. 00070 // as interface initializes mesh system that RF device is using 00071 tr_info("Mesh API test application - Thread mode"); 00072 // initialize the interface with registered device and callback 00073 status = ((MeshThread *)meshApi)->init(rf_device, mesh_network_callback, eui64, pskd); 00074 #else 00075 meshApi = (Mesh6LoWPAN_ND *)MeshInterfaceFactory::createInterface(MESH_TYPE_6LOWPAN_ND); 00076 // Use tr_info traces and RF interface after MeshAPi has been created. 00077 // as interface initializes mesh system that RF device is using 00078 tr_info("Mesh API test application - 6LoWPAN-ND mode"); 00079 // initialize the interface with registered device and callback 00080 status = ((Mesh6LoWPAN_ND *)meshApi)->init(rf_device_register(), mesh_network_callback); 00081 #endif 00082 00083 // Set ns_trace configuration level 00084 // set_trace_config(TRACE_ACTIVE_LEVEL_INFO|TRACE_MODE_COLOR|TRACE_CARRIAGE_RETURN); 00085 00086 if (status != MESH_ERROR_NONE) { 00087 tr_error("Can't initialize mesh network"); 00088 return; 00089 } 00090 00091 // connect interface to the network 00092 status = meshApi->connect(); 00093 if (status != MESH_ERROR_NONE) { 00094 tr_error("Can't connect to Mesh network!"); 00095 return; 00096 } 00097 }
Generated on Sun Jul 17 2022 08:25:26 by
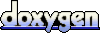