
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2013-2015 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 #include "utest/utest.h" 00020 #include "unity/unity.h" 00021 00022 using namespace utest::v1; 00023 00024 static int call_counter(0); 00025 00026 // Continue Teardown Handler ------------------------------------------------------------------------------------------ 00027 void continue_case() 00028 { 00029 TEST_ASSERT_EQUAL(0, call_counter++); 00030 } 00031 utest::v1::status_t continue_case_teardown(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00032 { 00033 TEST_ASSERT_EQUAL(1, passed); 00034 TEST_ASSERT_EQUAL(0, failed); 00035 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00036 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00037 TEST_ASSERT_EQUAL(1, call_counter++); 00038 return greentea_case_teardown_handler(source, passed, failed, failure); 00039 } 00040 utest::v1::status_t continue_failure(const Case *const, const failure_t) 00041 { 00042 TEST_FAIL_MESSAGE("Failure handler should have never been called!"); 00043 return STATUS_CONTINUE; 00044 } 00045 00046 // Ignoring Teardown Handler ------------------------------------------------------------------------------------------ 00047 void ignore_case() 00048 { 00049 TEST_ASSERT_EQUAL(2, call_counter++); 00050 } 00051 utest::v1::status_t ignore_case_teardown(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00052 { 00053 TEST_ASSERT_EQUAL(1, passed); 00054 TEST_ASSERT_EQUAL(0, failed); 00055 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00056 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00057 TEST_ASSERT_EQUAL(3, call_counter++); 00058 greentea_case_teardown_handler(source, passed, failed, failure); 00059 return STATUS_ABORT; 00060 } 00061 utest::v1::status_t ignore_failure(const Case *const source, const failure_t failure) 00062 { 00063 TEST_ASSERT_EQUAL(4, call_counter++); 00064 TEST_ASSERT_EQUAL(REASON_CASE_TEARDOWN, failure.reason); 00065 TEST_ASSERT_EQUAL(LOCATION_CASE_TEARDOWN, failure.location); 00066 verbose_case_failure_handler(source, failure.ignored ()); 00067 return STATUS_IGNORE; 00068 } 00069 00070 // Aborting Teardown Handler ------------------------------------------------------------------------------------------ 00071 void abort_case() 00072 { 00073 TEST_ASSERT_EQUAL(5, call_counter++); 00074 } 00075 utest::v1::status_t abort_case_teardown(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00076 { 00077 TEST_ASSERT_EQUAL(1, passed); 00078 TEST_ASSERT_EQUAL(0, failed); 00079 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00080 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00081 TEST_ASSERT_EQUAL(6, call_counter++); 00082 greentea_case_teardown_handler(source, passed, failed, failure); 00083 return STATUS_ABORT; 00084 } 00085 utest::v1::status_t abort_failure(const Case *const source, const failure_t failure) 00086 { 00087 TEST_ASSERT_EQUAL(7, call_counter++); 00088 TEST_ASSERT_EQUAL(REASON_CASE_TEARDOWN, failure.reason); 00089 TEST_ASSERT_EQUAL(LOCATION_CASE_TEARDOWN, failure.location); 00090 return verbose_case_failure_handler(source, failure); 00091 } 00092 00093 // Cases -------------------------------------------------------------------------------------------------------------- 00094 Case cases[] = { 00095 Case("Teardown handler returns CONTINUE", continue_case, continue_case_teardown, continue_failure), 00096 Case("Teardown handler returns ABORT but is IGNORED", ignore_case, ignore_case_teardown, ignore_failure), 00097 Case("Teardown handler returns ABORT", abort_case, abort_case_teardown, abort_failure) 00098 }; 00099 00100 // Specification: Setup & Teardown ------------------------------------------------------------------------------------ 00101 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00102 { 00103 GREENTEA_SETUP(15, "default_auto"); 00104 00105 return greentea_test_setup_handler(number_of_cases); 00106 } 00107 00108 void greentea_teardown(const size_t passed, const size_t failed, const failure_t failure) 00109 { 00110 TEST_ASSERT_EQUAL(8, call_counter++); 00111 TEST_ASSERT_EQUAL(2, passed); 00112 TEST_ASSERT_EQUAL(1, failed); 00113 TEST_ASSERT_EQUAL(REASON_CASE_TEARDOWN, failure.reason); 00114 TEST_ASSERT_EQUAL(LOCATION_CASE_TEARDOWN, failure.location); 00115 00116 // pretend to greentea that the test was successful 00117 greentea_test_teardown_handler(3, 0, REASON_NONE); 00118 } 00119 00120 Specification specification(greentea_setup, cases, greentea_teardown, selftest_handlers); 00121 00122 int main() 00123 { 00124 // Run the specification only AFTER setting the custom scheduler(if required). 00125 Harness::run(specification); 00126 }
Generated on Sun Jul 17 2022 08:25:26 by
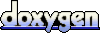