
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2013-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 #include "utest/utest.h" 00020 #include "unity/unity.h" 00021 00022 00023 using namespace utest::v1; 00024 00025 static int call_counter(0); 00026 static bool executed_case_0 = false; 00027 static bool executed_case_1 = false; 00028 static bool executed_case_2 = false; 00029 00030 void handler_case_2() 00031 { 00032 executed_case_2 = true; 00033 } 00034 utest::v1::status_t teardown_case_2(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00035 { 00036 TEST_ASSERT_TRUE(executed_case_2); 00037 TEST_ASSERT_EQUAL(1, passed); 00038 TEST_ASSERT_EQUAL(0, failed); 00039 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00040 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00041 TEST_ASSERT_EQUAL(0, call_counter++); 00042 greentea_case_teardown_handler(source, passed, failed, failure); 00043 return utest::v1::status_t(0); 00044 } 00045 void handler_case_0() 00046 { 00047 executed_case_0 = true; 00048 } 00049 utest::v1::status_t teardown_case_0(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00050 { 00051 TEST_ASSERT_TRUE(executed_case_0); 00052 TEST_ASSERT_EQUAL(1, passed); 00053 TEST_ASSERT_EQUAL(0, failed); 00054 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00055 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00056 TEST_ASSERT_EQUAL(1, call_counter++); 00057 greentea_case_teardown_handler(source, passed, failed, failure); 00058 return utest::v1::status_t(1); 00059 } 00060 void handler_case_1() 00061 { 00062 executed_case_1 = true; 00063 } 00064 utest::v1::status_t teardown_case_1(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00065 { 00066 TEST_ASSERT_TRUE(executed_case_1); 00067 TEST_ASSERT_EQUAL(1, passed); 00068 TEST_ASSERT_EQUAL(0, failed); 00069 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00070 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00071 TEST_ASSERT_EQUAL(2, call_counter++); 00072 greentea_case_teardown_handler(source, passed, failed, failure); 00073 return utest::v1::status_t(3); 00074 } 00075 00076 Case cases[] = 00077 { 00078 Case("Case 1", handler_case_0, teardown_case_0), 00079 Case("Case 2", handler_case_1, teardown_case_1), 00080 Case("Case 3", handler_case_2, teardown_case_2) 00081 }; 00082 00083 utest::v1::status_t test_setup_handler(const size_t number_of_cases) 00084 { 00085 GREENTEA_SETUP(5, "default_auto"); 00086 00087 greentea_test_setup_handler(number_of_cases); 00088 return utest::v1::status_t(2); 00089 }; 00090 void test_teardown_handler(const size_t passed, const size_t failed, const failure_t failure) 00091 { 00092 TEST_ASSERT_EQUAL(3, passed); 00093 TEST_ASSERT_EQUAL(0, failed); 00094 TEST_ASSERT_EQUAL(REASON_NONE, failure.reason); 00095 TEST_ASSERT_EQUAL(LOCATION_NONE, failure.location); 00096 TEST_ASSERT_EQUAL(3, call_counter++); 00097 00098 greentea_test_teardown_handler(passed, failed, failure); 00099 }; 00100 00101 Specification specification(test_setup_handler, cases, test_teardown_handler, selftest_handlers); 00102 00103 int main() 00104 { 00105 // Run the specification only AFTER setting the custom scheduler(if required). 00106 Harness::run(specification); 00107 }
Generated on Sun Jul 17 2022 08:25:26 by
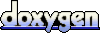