
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "greentea-client/test_env.h" 00018 #include "unity.h" 00019 #include "utest.h" 00020 00021 #include "HeapBlockDevice.h" 00022 #include "SlicingBlockDevice.h" 00023 #include "ChainingBlockDevice.h" 00024 #include "ProfilingBlockDevice.h" 00025 #include <stdlib.h> 00026 00027 using namespace utest::v1; 00028 00029 #define BLOCK_COUNT 16 00030 #define BLOCK_SIZE 512 00031 00032 00033 // Simple test which read/writes blocks on a sliced block device 00034 void test_slicing() { 00035 HeapBlockDevice bd(BLOCK_COUNT*BLOCK_SIZE, BLOCK_SIZE); 00036 uint8_t *write_block = new uint8_t[BLOCK_SIZE]; 00037 uint8_t *read_block = new uint8_t[BLOCK_SIZE]; 00038 00039 // Test with first slice of block device 00040 SlicingBlockDevice slice1(&bd, 0, (BLOCK_COUNT/2)*BLOCK_SIZE); 00041 00042 int err = slice1.init(); 00043 TEST_ASSERT_EQUAL(0, err); 00044 00045 TEST_ASSERT_EQUAL(BLOCK_SIZE, slice1.get_program_size()); 00046 TEST_ASSERT_EQUAL((BLOCK_COUNT/2)*BLOCK_SIZE, slice1.size()); 00047 00048 // Fill with random sequence 00049 srand(1); 00050 for (int i = 0; i < BLOCK_SIZE; i++) { 00051 write_block[i] = 0xff & rand(); 00052 } 00053 00054 // Write, sync, and read the block 00055 err = slice1.program(write_block, 0, BLOCK_SIZE); 00056 TEST_ASSERT_EQUAL(0, err); 00057 00058 err = slice1.read(read_block, 0, BLOCK_SIZE); 00059 TEST_ASSERT_EQUAL(0, err); 00060 00061 // Check that the data was unmodified 00062 srand(1); 00063 for (int i = 0; i < BLOCK_SIZE; i++) { 00064 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00065 } 00066 00067 // Check with original block device 00068 err = bd.read(read_block, 0, BLOCK_SIZE); 00069 TEST_ASSERT_EQUAL(0, err); 00070 00071 // Check that the data was unmodified 00072 srand(1); 00073 for (int i = 0; i < BLOCK_SIZE; i++) { 00074 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00075 } 00076 00077 err = slice1.deinit(); 00078 TEST_ASSERT_EQUAL(0, err); 00079 00080 00081 // Test with second slice of block device 00082 SlicingBlockDevice slice2(&bd, -(BLOCK_COUNT/2)*BLOCK_SIZE); 00083 00084 err = slice2.init(); 00085 TEST_ASSERT_EQUAL(0, err); 00086 00087 TEST_ASSERT_EQUAL(BLOCK_SIZE, slice2.get_program_size()); 00088 TEST_ASSERT_EQUAL((BLOCK_COUNT/2)*BLOCK_SIZE, slice2.size()); 00089 00090 // Fill with random sequence 00091 srand(1); 00092 for (int i = 0; i < BLOCK_SIZE; i++) { 00093 write_block[i] = 0xff & rand(); 00094 } 00095 00096 // Write, sync, and read the block 00097 err = slice2.program(write_block, 0, BLOCK_SIZE); 00098 TEST_ASSERT_EQUAL(0, err); 00099 00100 err = slice2.read(read_block, 0, BLOCK_SIZE); 00101 TEST_ASSERT_EQUAL(0, err); 00102 00103 // Check that the data was unmodified 00104 srand(1); 00105 for (int i = 0; i < BLOCK_SIZE; i++) { 00106 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00107 } 00108 00109 // Check with original block device 00110 err = bd.read(read_block, (BLOCK_COUNT/2)*BLOCK_SIZE, BLOCK_SIZE); 00111 TEST_ASSERT_EQUAL(0, err); 00112 00113 // Check that the data was unmodified 00114 srand(1); 00115 for (int i = 0; i < BLOCK_SIZE; i++) { 00116 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00117 } 00118 00119 delete[] write_block; 00120 delete[] read_block; 00121 err = slice2.deinit(); 00122 TEST_ASSERT_EQUAL(0, err); 00123 } 00124 00125 // Simple test which read/writes blocks on a chain of block devices 00126 void test_chaining() { 00127 HeapBlockDevice bd1((BLOCK_COUNT/2)*BLOCK_SIZE, BLOCK_SIZE); 00128 HeapBlockDevice bd2((BLOCK_COUNT/2)*BLOCK_SIZE, BLOCK_SIZE); 00129 uint8_t *write_block = new uint8_t[BLOCK_SIZE]; 00130 uint8_t *read_block = new uint8_t[BLOCK_SIZE]; 00131 00132 // Test with chain of block device 00133 BlockDevice *bds[] = {&bd1, &bd2}; 00134 ChainingBlockDevice chain(bds); 00135 00136 int err = chain.init(); 00137 TEST_ASSERT_EQUAL(0, err); 00138 00139 TEST_ASSERT_EQUAL(BLOCK_SIZE, chain.get_program_size()); 00140 TEST_ASSERT_EQUAL(BLOCK_COUNT*BLOCK_SIZE, chain.size()); 00141 00142 // Fill with random sequence 00143 srand(1); 00144 for (int i = 0; i < BLOCK_SIZE; i++) { 00145 write_block[i] = 0xff & rand(); 00146 } 00147 00148 // Write, sync, and read the block 00149 err = chain.program(write_block, 0, BLOCK_SIZE); 00150 TEST_ASSERT_EQUAL(0, err); 00151 00152 err = chain.read(read_block, 0, BLOCK_SIZE); 00153 TEST_ASSERT_EQUAL(0, err); 00154 00155 // Check that the data was unmodified 00156 srand(1); 00157 for (int i = 0; i < BLOCK_SIZE; i++) { 00158 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00159 } 00160 00161 // Write, sync, and read the block 00162 err = chain.program(write_block, (BLOCK_COUNT/2)*BLOCK_SIZE, BLOCK_SIZE); 00163 TEST_ASSERT_EQUAL(0, err); 00164 00165 err = chain.read(read_block, (BLOCK_COUNT/2)*BLOCK_SIZE, BLOCK_SIZE); 00166 TEST_ASSERT_EQUAL(0, err); 00167 00168 // Check that the data was unmodified 00169 srand(1); 00170 for (int i = 0; i < BLOCK_SIZE; i++) { 00171 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00172 } 00173 00174 delete[] write_block; 00175 delete[] read_block; 00176 err = chain.deinit(); 00177 TEST_ASSERT_EQUAL(0, err); 00178 } 00179 00180 // Simple test which read/writes blocks on a chain of block devices 00181 void test_profiling() { 00182 HeapBlockDevice bd(BLOCK_COUNT*BLOCK_SIZE, BLOCK_SIZE); 00183 uint8_t *write_block = new uint8_t[BLOCK_SIZE]; 00184 uint8_t *read_block = new uint8_t[BLOCK_SIZE]; 00185 00186 // Test under profiling 00187 ProfilingBlockDevice profiler(&bd); 00188 00189 int err = profiler.init(); 00190 TEST_ASSERT_EQUAL(0, err); 00191 00192 TEST_ASSERT_EQUAL(BLOCK_SIZE, profiler.get_erase_size()); 00193 TEST_ASSERT_EQUAL(BLOCK_COUNT*BLOCK_SIZE, profiler.size()); 00194 00195 // Fill with random sequence 00196 srand(1); 00197 for (int i = 0; i < BLOCK_SIZE; i++) { 00198 write_block[i] = 0xff & rand(); 00199 } 00200 00201 // Write, sync, and read the block 00202 err = profiler.erase(0, BLOCK_SIZE); 00203 TEST_ASSERT_EQUAL(0, err); 00204 00205 err = profiler.program(write_block, 0, BLOCK_SIZE); 00206 TEST_ASSERT_EQUAL(0, err); 00207 00208 err = profiler.read(read_block, 0, BLOCK_SIZE); 00209 TEST_ASSERT_EQUAL(0, err); 00210 00211 // Check that the data was unmodified 00212 srand(1); 00213 for (int i = 0; i < BLOCK_SIZE; i++) { 00214 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00215 } 00216 00217 // Check with original block device 00218 err = bd.read(read_block, 0, BLOCK_SIZE); 00219 TEST_ASSERT_EQUAL(0, err); 00220 00221 // Check that the data was unmodified 00222 srand(1); 00223 for (int i = 0; i < BLOCK_SIZE; i++) { 00224 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00225 } 00226 00227 delete[] write_block; 00228 delete[] read_block; 00229 err = profiler.deinit(); 00230 TEST_ASSERT_EQUAL(0, err); 00231 00232 // Check that profiled operations match expectations 00233 bd_size_t read_count = profiler.get_read_count(); 00234 TEST_ASSERT_EQUAL(BLOCK_SIZE, read_count); 00235 bd_size_t program_count = profiler.get_program_count(); 00236 TEST_ASSERT_EQUAL(BLOCK_SIZE, program_count); 00237 bd_size_t erase_count = profiler.get_erase_count(); 00238 TEST_ASSERT_EQUAL(BLOCK_SIZE, erase_count); 00239 } 00240 00241 00242 // Test setup 00243 utest::v1::status_t test_setup(const size_t number_of_cases) { 00244 GREENTEA_SETUP(10, "default_auto"); 00245 return verbose_test_setup_handler(number_of_cases); 00246 } 00247 00248 Case cases[] = { 00249 Case("Testing slicing of a block device", test_slicing), 00250 Case("Testing chaining of block devices", test_chaining), 00251 Case("Testing profiling of block devices", test_profiling), 00252 }; 00253 00254 Specification specification(test_setup, cases); 00255 00256 int main() { 00257 return !Harness::run(specification); 00258 }
Generated on Sun Jul 17 2022 08:25:26 by
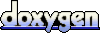