
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "greentea-client/test_env.h" 00018 #include "unity.h" 00019 #include "utest.h" 00020 00021 #include "HeapBlockDevice.h" 00022 #include <stdlib.h> 00023 00024 using namespace utest::v1; 00025 00026 #define TEST_BLOCK_SIZE 128 00027 #define TEST_BLOCK_DEVICE_SIZE 32*TEST_BLOCK_SIZE 00028 #define TEST_BLOCK_COUNT 10 00029 #define TEST_ERROR_MASK 16 00030 00031 const struct { 00032 const char *name; 00033 bd_size_t (BlockDevice::*method)() const; 00034 } ATTRS[] = { 00035 {"read size", &BlockDevice::get_read_size}, 00036 {"program size", &BlockDevice::get_program_size}, 00037 {"erase size", &BlockDevice::get_erase_size}, 00038 {"total size", &BlockDevice::size}, 00039 }; 00040 00041 00042 // Simple test that read/writes random set of blocks 00043 void test_read_write() { 00044 HeapBlockDevice bd(TEST_BLOCK_DEVICE_SIZE, TEST_BLOCK_SIZE); 00045 00046 int err = bd.init(); 00047 TEST_ASSERT_EQUAL(0, err); 00048 00049 for (unsigned a = 0; a < sizeof(ATTRS)/sizeof(ATTRS[0]); a++) { 00050 static const char *prefixes[] = {"", "k", "M", "G"}; 00051 for (int i = 3; i >= 0; i--) { 00052 bd_size_t size = (bd.*ATTRS[a].method)(); 00053 if (size >= (1ULL << 10*i)) { 00054 printf("%s: %llu%sbytes (%llubytes)\n", 00055 ATTRS[a].name, size >> 10*i, prefixes[i], size); 00056 break; 00057 } 00058 } 00059 } 00060 00061 bd_size_t block_size = bd.get_erase_size(); 00062 uint8_t *write_block = new uint8_t[block_size]; 00063 uint8_t *read_block = new uint8_t[block_size]; 00064 uint8_t *error_mask = new uint8_t[TEST_ERROR_MASK]; 00065 unsigned addrwidth = ceil(log(float(bd.size()-1)) / log(float(16)))+1; 00066 00067 for (int b = 0; b < TEST_BLOCK_COUNT; b++) { 00068 // Find a random block 00069 bd_addr_t block = (rand()*block_size) % bd.size(); 00070 00071 // Use next random number as temporary seed to keep 00072 // the address progressing in the pseudorandom sequence 00073 unsigned seed = rand(); 00074 00075 // Fill with random sequence 00076 srand(seed); 00077 for (bd_size_t i = 0; i < block_size; i++) { 00078 write_block[i] = 0xff & rand(); 00079 } 00080 00081 // erase, program, and read the block 00082 printf("test %0*llx:%llu...\n", addrwidth, block, block_size); 00083 00084 err = bd.erase(block, block_size); 00085 TEST_ASSERT_EQUAL(0, err); 00086 00087 err = bd.program(write_block, block, block_size); 00088 TEST_ASSERT_EQUAL(0, err); 00089 00090 printf("write %0*llx:%llu ", addrwidth, block, block_size); 00091 for (int i = 0; i < 16; i++) { 00092 printf("%02x", write_block[i]); 00093 } 00094 printf("...\n"); 00095 00096 err = bd.read(read_block, block, block_size); 00097 TEST_ASSERT_EQUAL(0, err); 00098 00099 printf("read %0*llx:%llu ", addrwidth, block, block_size); 00100 for (int i = 0; i < 16; i++) { 00101 printf("%02x", read_block[i]); 00102 } 00103 printf("...\n"); 00104 00105 // Find error mask for debugging 00106 memset(error_mask, 0, TEST_ERROR_MASK); 00107 bd_size_t error_scale = block_size / (TEST_ERROR_MASK*8); 00108 00109 srand(seed); 00110 for (bd_size_t i = 0; i < TEST_ERROR_MASK*8; i++) { 00111 for (bd_size_t j = 0; j < error_scale; j++) { 00112 if ((0xff & rand()) != read_block[i*error_scale + j]) { 00113 error_mask[i/8] |= 1 << (i%8); 00114 } 00115 } 00116 } 00117 00118 printf("error %0*llx:%llu ", addrwidth, block, block_size); 00119 for (int i = 0; i < 16; i++) { 00120 printf("%02x", error_mask[i]); 00121 } 00122 printf("\n"); 00123 00124 // Check that the data was unmodified 00125 srand(seed); 00126 for (bd_size_t i = 0; i < block_size; i++) { 00127 TEST_ASSERT_EQUAL(0xff & rand(), read_block[i]); 00128 } 00129 } 00130 00131 err = bd.deinit(); 00132 TEST_ASSERT_EQUAL(0, err); 00133 } 00134 00135 00136 // Test setup 00137 utest::v1::status_t test_setup(const size_t number_of_cases) { 00138 GREENTEA_SETUP(30, "default_auto"); 00139 return verbose_test_setup_handler(number_of_cases); 00140 } 00141 00142 Case cases[] = { 00143 Case("Testing read write random blocks", test_read_write), 00144 }; 00145 00146 Specification specification(test_setup, cases); 00147 00148 int main() { 00149 return !Harness::run(specification); 00150 }
Generated on Sun Jul 17 2022 08:25:26 by
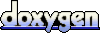