
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2013-2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBED_CONF_APP_CONNECT_STATEMENT 00019 #error [NOT_SUPPORTED] No network configuration found for this target. 00020 #endif 00021 00022 #ifndef MBED_EXTENDED_TESTS 00023 #error [NOT_SUPPORTED] Parallel tests are not supported by default 00024 #endif 00025 00026 #include "mbed.h" 00027 #include MBED_CONF_APP_HEADER_FILE 00028 #include "UDPSocket.h" 00029 #include "greentea-client/test_env.h" 00030 #include "unity/unity.h" 00031 #include "utest.h" 00032 00033 using namespace utest::v1; 00034 00035 00036 #ifndef MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE 00037 #define MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE 64 00038 #endif 00039 00040 #ifndef MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT 00041 #define MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT 500 00042 #endif 00043 00044 #ifndef MBED_CFG_UDP_CLIENT_ECHO_THREADS 00045 #define MBED_CFG_UDP_CLIENT_ECHO_THREADS 3 00046 #endif 00047 00048 #define STRINGIZE(x) STRINGIZE2(x) 00049 #define STRINGIZE2(x) #x 00050 00051 00052 const int ECHO_LOOPS = 16; 00053 NetworkInterface* net; 00054 SocketAddress udp_addr; 00055 Mutex iomutex; 00056 char uuid[48] = {0}; 00057 00058 // NOTE: assuming that "id" stays in the single digits 00059 void prep_buffer(int id, char *uuid, char *tx_buffer, size_t tx_size) { 00060 size_t i = 0; 00061 00062 tx_buffer[i++] = '0' + id; 00063 tx_buffer[i++] = ' '; 00064 00065 memcpy(tx_buffer+i, uuid, strlen(uuid)); 00066 i += strlen(uuid); 00067 00068 tx_buffer[i++] = ' '; 00069 00070 for (; i<tx_size; ++i) { 00071 tx_buffer[i] = (rand() % 10) + '0'; 00072 } 00073 } 00074 00075 00076 // Each echo class is in charge of one parallel transaction 00077 class Echo { 00078 private: 00079 char tx_buffer[MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE]; 00080 char rx_buffer[MBED_CFG_UDP_CLIENT_ECHO_BUFFER_SIZE]; 00081 00082 UDPSocket sock; 00083 Thread thread; 00084 bool result; 00085 int id; 00086 char *uuid; 00087 00088 public: 00089 // Limiting stack size to 1k 00090 Echo(): thread(osPriorityNormal, 1024), result(false) { 00091 } 00092 00093 void start(int id, char *uuid) { 00094 this->id = id; 00095 this->uuid = uuid; 00096 osStatus status = thread.start(callback(this, &Echo::echo)); 00097 } 00098 00099 void join() { 00100 osStatus status = thread.join(); 00101 TEST_ASSERT_EQUAL(osOK, status); 00102 } 00103 00104 void echo() { 00105 int success = 0; 00106 00107 int err = sock.open(net); 00108 TEST_ASSERT_EQUAL(0, err); 00109 00110 sock.set_timeout(MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT); 00111 00112 for (int i = 0; success < ECHO_LOOPS; i++) { 00113 prep_buffer(id, uuid, tx_buffer, sizeof(tx_buffer)); 00114 const int ret = sock.sendto(udp_addr, tx_buffer, sizeof(tx_buffer)); 00115 if (ret >= 0) { 00116 iomutex.lock(); 00117 printf("[ID:%01d][%02d] sent %d bytes - %.*s \n", id, i, ret, ret, tx_buffer); 00118 iomutex.unlock(); 00119 } else { 00120 iomutex.lock(); 00121 printf("[ID:%01d][%02d] Network error %d\n", id, i, ret); 00122 iomutex.unlock(); 00123 continue; 00124 } 00125 00126 SocketAddress temp_addr; 00127 const int n = sock.recvfrom(&temp_addr, rx_buffer, sizeof(rx_buffer)); 00128 if (n >= 0) { 00129 iomutex.lock(); 00130 printf("[ID:%01d][%02d] recv %d bytes - %.*s \n", id, i, n, n, tx_buffer); 00131 iomutex.unlock(); 00132 } else { 00133 iomutex.lock(); 00134 printf("[ID:%01d][%02d] Network error %d\n", id, i, n); 00135 iomutex.unlock(); 00136 continue; 00137 } 00138 00139 if ((temp_addr == udp_addr && 00140 n == sizeof(tx_buffer) && 00141 memcmp(rx_buffer, tx_buffer, sizeof(rx_buffer)) == 0)) { 00142 success += 1; 00143 iomutex.lock(); 00144 printf("[ID:%01d][%02d] success #%d\n", id, i, success); 00145 iomutex.unlock(); 00146 continue; 00147 } 00148 00149 // failed, clean out any remaining bad packets 00150 sock.set_timeout(0); 00151 while (true) { 00152 err = sock.recvfrom(NULL, NULL, 0); 00153 if (err == NSAPI_ERROR_WOULD_BLOCK ) { 00154 break; 00155 } 00156 } 00157 sock.set_timeout(MBED_CFG_UDP_CLIENT_ECHO_TIMEOUT); 00158 } 00159 00160 result = success == ECHO_LOOPS; 00161 00162 err = sock.close(); 00163 TEST_ASSERT_EQUAL(0, err); 00164 if (err) { 00165 result = false; 00166 } 00167 } 00168 00169 bool get_result() { 00170 return result; 00171 } 00172 }; 00173 00174 Echo *echoers[MBED_CFG_UDP_CLIENT_ECHO_THREADS]; 00175 00176 00177 void test_udp_echo_parallel() { 00178 net = MBED_CONF_APP_OBJECT_CONSTRUCTION; 00179 int err = MBED_CONF_APP_CONNECT_STATEMENT; 00180 TEST_ASSERT_EQUAL(0, err); 00181 00182 if (err) { 00183 printf("MBED: failed to connect with an error of %d\r\n", err); 00184 GREENTEA_TESTSUITE_RESULT(false); 00185 } else { 00186 printf("UDP client IP Address is %s\n", net->get_ip_address()); 00187 00188 udp_addr.set_ip_address(MBED_CONF_APP_ECHO_SERVER_ADDR); 00189 udp_addr.set_port(MBED_CONF_APP_ECHO_SERVER_PORT); 00190 00191 // Startup echo threads in parallel 00192 for (int i = 0; i < MBED_CFG_UDP_CLIENT_ECHO_THREADS; i++) { 00193 echoers[i] = new Echo; 00194 echoers[i]->start(i, uuid); 00195 } 00196 00197 bool result = true; 00198 00199 for (int i = 0; i < MBED_CFG_UDP_CLIENT_ECHO_THREADS; i++) { 00200 echoers[i]->join(); 00201 result = result && echoers[i]->get_result(); 00202 delete echoers[i]; 00203 } 00204 00205 net->disconnect(); 00206 TEST_ASSERT_EQUAL(true, result); 00207 } 00208 } 00209 00210 00211 // Test setup 00212 utest::v1::status_t test_setup(const size_t number_of_cases) { 00213 GREENTEA_SETUP(120, "udp_echo"); 00214 return verbose_test_setup_handler(number_of_cases); 00215 } 00216 00217 Case cases[] = { 00218 Case("UDP echo parallel", test_udp_echo_parallel), 00219 }; 00220 00221 Specification specification(test_setup, cases); 00222 00223 int main() { 00224 return !Harness::run(specification); 00225 }
Generated on Sun Jul 17 2022 08:25:27 by
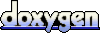