
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2013-2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBED_CONF_APP_CONNECT_STATEMENT 00019 #error [NOT_SUPPORTED] No network configuration found for this target. 00020 #endif 00021 00022 #include "mbed.h" 00023 #include "greentea-client/test_env.h" 00024 #include "unity.h" 00025 #include "utest.h" 00026 00027 using namespace utest::v1; 00028 00029 00030 // IP parsing verification 00031 void test_ip_accept(const char *string, nsapi_addr_t addr) { 00032 SocketAddress address; 00033 TEST_ASSERT(address.set_ip_address(string)); 00034 TEST_ASSERT(address == SocketAddress(addr)); 00035 } 00036 00037 template <const char *string> 00038 void test_ip_reject() { 00039 SocketAddress address; 00040 TEST_ASSERT(!address.set_ip_address(string)); 00041 TEST_ASSERT(!address); 00042 } 00043 00044 #define TEST_IP_ACCEPT(name, string, ...) \ 00045 void name() { \ 00046 nsapi_addr_t addr = __VA_ARGS__; \ 00047 test_ip_accept(string, addr); \ 00048 } 00049 00050 #define TEST_IP_REJECT(name, string) \ 00051 void name() { \ 00052 test_ip_reject(string); \ 00053 } 00054 00055 00056 // Test cases 00057 TEST_IP_ACCEPT(test_simple_ipv4_address, 00058 "12.34.56.78", 00059 {NSAPI_IPv4 ,{12,34,56,78}}) 00060 TEST_IP_ACCEPT(test_left_weighted_ipv4_address, 00061 "255.0.0.0", 00062 {NSAPI_IPv4 ,{255,0,0,0}}) 00063 TEST_IP_ACCEPT(test_right_weighted_ipv4_address, 00064 "0.0.0.255", 00065 {NSAPI_IPv4 ,{0,0,0,255}}) 00066 TEST_IP_ACCEPT(test_null_ipv4_address, 00067 "0.0.0.0", 00068 {NSAPI_IPv4 ,{0,0,0,0}}) 00069 00070 TEST_IP_ACCEPT(test_simple_ipv6_address, 00071 "1234:5678:9abc:def0:1234:5678:9abc:def0", 00072 {NSAPI_IPv6 ,{0x12,0x34,0x56,0x78,0x9a,0xbc,0xde,0xf0, 00073 0x12,0x34,0x56,0x78,0x9a,0xbc,0xde,0xf0}}) 00074 TEST_IP_ACCEPT(test_left_weighted_ipv6_address, 00075 "1234:5678::", 00076 {NSAPI_IPv6 ,{0x12,0x34,0x56,0x78,0x00,0x00,0x00,0x00, 00077 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00}}) 00078 TEST_IP_ACCEPT(test_right_weighted_ipv6_address, 00079 "::1234:5678", 00080 {NSAPI_IPv6 ,{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, 00081 0x00,0x00,0x00,0x00,0x12,0x34,0x56,0x78}}) 00082 TEST_IP_ACCEPT(test_hollowed_ipv6_address, 00083 "1234:5678::9abc:def8", 00084 {NSAPI_IPv6 ,{0x12,0x34,0x56,0x78,0x00,0x00,0x00,0x00, 00085 0x00,0x00,0x00,0x00,0x9a,0xbc,0xde,0xf8}}) 00086 TEST_IP_ACCEPT(test_null_ipv6_address, 00087 "::", 00088 {NSAPI_IPv6 ,{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, 00089 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00}}) 00090 00091 00092 // Test setup 00093 utest::v1::status_t test_setup(const size_t number_of_cases) { 00094 GREENTEA_SETUP(10, "default_auto"); 00095 return verbose_test_setup_handler(number_of_cases); 00096 } 00097 00098 Case cases[] = { 00099 Case("Simple IPv4 address", test_simple_ipv4_address), 00100 Case("Left-weighted IPv4 address", test_left_weighted_ipv4_address), 00101 Case("Right-weighted IPv4 address", test_right_weighted_ipv4_address), 00102 Case("Null IPv4 address", test_null_ipv4_address), 00103 00104 Case("Simple IPv6 address", test_simple_ipv6_address), 00105 Case("Left-weighted IPv6 address", test_left_weighted_ipv6_address), 00106 Case("Right-weighted IPv6 address", test_right_weighted_ipv6_address), 00107 Case("Hollowed IPv6 address", test_hollowed_ipv6_address), 00108 Case("Null IPv6 address", test_null_ipv6_address), 00109 }; 00110 00111 Specification specification(test_setup, cases); 00112 00113 int main() { 00114 return !Harness::run(specification); 00115 }
Generated on Sun Jul 17 2022 08:25:27 by
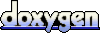