
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <utility> // std::pair 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 00020 uint32_t test_64(uint64_t ticks) { 00021 ticks >>= 3; // divide by 8 00022 if (ticks > 0xFFFFFFFF) { 00023 ticks /= 3; 00024 } else { 00025 ticks = (ticks * 0x55555556) >> 32; // divide by 3 00026 } 00027 return (uint32_t)(0xFFFFFFFF & ticks); 00028 } 00029 00030 const char *result_str(bool result) { 00031 return result ? "[OK]" : "[FAIL]"; 00032 } 00033 00034 int main() { 00035 GREENTEA_SETUP(5, "default_auto"); 00036 00037 bool result = true; 00038 00039 { // 0xFFFFFFFF * 8 = 0x7fffffff8 00040 std::pair<uint32_t, uint64_t> values = std::make_pair(0x55555555, 0x7FFFFFFF8); 00041 uint32_t test_ret = test_64(values.second); 00042 bool test_res = values.first == test_ret; 00043 result = result && test_res; 00044 printf("64bit: 0x7FFFFFFF8: expected 0x%lX got 0x%lX ... %s\r\n", values.first, test_ret, result_str(test_res)); 00045 } 00046 00047 { // 0xFFFFFFFF * 24 = 0x17ffffffe8 00048 std::pair<uint32_t, uint64_t> values = std::make_pair(0xFFFFFFFF, 0x17FFFFFFE8); 00049 uint32_t test_ret = test_64(values.second); 00050 bool test_res = values.first == test_ret; 00051 result = result && test_res; 00052 printf("64bit: 0x17FFFFFFE8: expected 0x%lX got 0x%lX ... %s\r\n", values.first, test_ret, result_str(test_res)); 00053 } 00054 00055 GREENTEA_TESTSUITE_RESULT(result); 00056 }
Generated on Sun Jul 17 2022 08:25:27 by
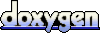