
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #if !DEVICE_SLEEP 00019 #error [NOT_SUPPORTED] Sleep not supported for this target 00020 #endif 00021 00022 #include "utest/utest.h" 00023 #include "unity/unity.h" 00024 #include "greentea-client/test_env.h" 00025 00026 #include "mbed.h" 00027 00028 using namespace utest::v1; 00029 00030 void deep_sleep_lock_lock_test() 00031 { 00032 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00033 { 00034 // Check basic usage works 00035 DeepSleepLock lock; 00036 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00037 } 00038 00039 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00040 { 00041 // Check that unlock and lock change can deep sleep as expected 00042 DeepSleepLock lock; 00043 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00044 lock.unlock(); 00045 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00046 lock.lock(); 00047 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00048 } 00049 00050 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00051 { 00052 // Check that unlock releases sleep based on count 00053 DeepSleepLock lock; 00054 lock.lock(); 00055 lock.lock(); 00056 lock.unlock(); 00057 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00058 } 00059 00060 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00061 { 00062 // Check that unbalanced locks do not leave deep sleep locked 00063 DeepSleepLock lock; 00064 lock.lock(); 00065 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00066 } 00067 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00068 00069 } 00070 00071 void timer_lock_test() 00072 { 00073 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00074 { 00075 // Just creating a timer object does not lock sleep 00076 Timer timer; 00077 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00078 } 00079 00080 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00081 { 00082 // Starting a timer does lock sleep 00083 Timer timer; 00084 timer.start(); 00085 TEST_ASSERT_EQUAL(false, sleep_manager_can_deep_sleep()); 00086 } 00087 00088 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00089 { 00090 // Stopping a timer after starting it allows sleep 00091 Timer timer; 00092 timer.start(); 00093 timer.stop(); 00094 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00095 } 00096 00097 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00098 { 00099 // Starting a timer multiple times still lets you sleep 00100 Timer timer; 00101 timer.start(); 00102 timer.start(); 00103 } 00104 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00105 00106 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00107 { 00108 // Stopping a timer multiple times still lets you sleep 00109 Timer timer; 00110 timer.start(); 00111 timer.stop(); 00112 timer.stop(); 00113 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00114 } 00115 TEST_ASSERT_EQUAL(true, sleep_manager_can_deep_sleep()); 00116 } 00117 00118 Case cases[] = { 00119 Case("DeepSleepLock lock test", deep_sleep_lock_lock_test), 00120 Case("timer lock test", timer_lock_test), 00121 }; 00122 00123 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) { 00124 GREENTEA_SETUP(20, "default_auto"); 00125 return greentea_test_setup_handler(number_of_cases); 00126 } 00127 00128 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00129 00130 int main() { 00131 Harness::run(specification); 00132 }
Generated on Sun Jul 17 2022 08:25:27 by
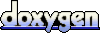