
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 #include "unity/unity.h" 00020 #include "utest/utest.h" 00021 00022 using namespace utest::v1; 00023 00024 #define CUSTOM_TIME 1256729737 00025 00026 void test_case_rtc_strftime() { 00027 greentea_send_kv("timestamp", CUSTOM_TIME); 00028 00029 char buffer[32] = {0}; 00030 char kv_buff[64] = {0}; 00031 set_time(CUSTOM_TIME); // Set RTC time to Wed, 28 Oct 2009 11:35:37 00032 00033 for (int i=0; i<10; ++i) { 00034 time_t seconds = time(NULL); 00035 sprintf(kv_buff, "[%ld] ", seconds); 00036 strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S %p", localtime(&seconds)); 00037 strcat(kv_buff, buffer); 00038 greentea_send_kv("rtc", kv_buff); 00039 wait(1); 00040 } 00041 } 00042 00043 Case cases[] = { 00044 Case("RTC strftime", test_case_rtc_strftime), 00045 }; 00046 00047 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) { 00048 GREENTEA_SETUP(20, "rtc_auto"); 00049 return greentea_test_setup_handler(number_of_cases); 00050 } 00051 00052 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00053 00054 int main() { 00055 Harness::run(specification); 00056 }
Generated on Sun Jul 17 2022 08:25:27 by
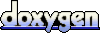