
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #if !DEVICE_FLASH 00019 #error [NOT_SUPPORTED] Flash API not supported for this target 00020 #endif 00021 00022 #include "utest/utest.h" 00023 #include "unity/unity.h" 00024 #include "greentea-client/test_env.h" 00025 00026 #include "mbed.h" 00027 00028 using namespace utest::v1; 00029 00030 void flashiap_init_test() 00031 { 00032 FlashIAP flash_device; 00033 uint32_t ret = flash_device.init(); 00034 TEST_ASSERT_EQUAL_INT32(0, ret); 00035 ret = flash_device.deinit(); 00036 TEST_ASSERT_EQUAL_INT32(0, ret); 00037 } 00038 00039 void flashiap_program_test() 00040 { 00041 FlashIAP flash_device; 00042 uint32_t ret = flash_device.init(); 00043 TEST_ASSERT_EQUAL_INT32(0, ret); 00044 00045 // get the last sector size (flash size - 1) 00046 uint32_t sector_size = flash_device.get_sector_size(flash_device.get_flash_start() + flash_device.get_flash_size() - 1UL); 00047 uint32_t page_size = flash_device.get_page_size(); 00048 TEST_ASSERT_NOT_EQUAL(0, sector_size); 00049 TEST_ASSERT_NOT_EQUAL(0, page_size); 00050 TEST_ASSERT_TRUE(sector_size % page_size == 0); 00051 const uint8_t test_value = 0xCE; 00052 uint8_t *data = new uint8_t[page_size]; 00053 for (uint32_t i = 0; i < page_size; i++) { 00054 data[i] = test_value; 00055 } 00056 00057 // the one before the last sector in the system 00058 uint32_t address = (flash_device.get_flash_start() + flash_device.get_flash_size()) - (sector_size); 00059 TEST_ASSERT_TRUE(address != 0UL); 00060 ret = flash_device.erase(address, sector_size); 00061 TEST_ASSERT_EQUAL_INT32(0, ret); 00062 00063 00064 for (uint32_t i = 0; i < sector_size / page_size; i++) { 00065 uint32_t page_addr = address + i * page_size; 00066 ret = flash_device.program(data, page_addr, page_size); 00067 TEST_ASSERT_EQUAL_INT32(0, ret); 00068 } 00069 00070 uint8_t *data_flashed = new uint8_t[page_size]; 00071 for (uint32_t i = 0; i < sector_size / page_size; i++) { 00072 uint32_t page_addr = address + i * page_size; 00073 ret = flash_device.read(data_flashed, page_addr, page_size); 00074 TEST_ASSERT_EQUAL_INT32(0, ret); 00075 TEST_ASSERT_EQUAL_UINT8_ARRAY(data, data_flashed, page_size); 00076 } 00077 delete[] data; 00078 delete[] data_flashed; 00079 00080 ret = flash_device.deinit(); 00081 TEST_ASSERT_EQUAL_INT32(0, ret); 00082 } 00083 00084 void flashiap_program_error_test() 00085 { 00086 FlashIAP flash_device; 00087 uint32_t ret = flash_device.init(); 00088 TEST_ASSERT_EQUAL_INT32(0, ret); 00089 00090 // get the last sector size (flash size - 1) 00091 uint32_t sector_size = flash_device.get_sector_size(flash_device.get_flash_start() + flash_device.get_flash_size() - 1UL); 00092 uint32_t page_size = flash_device.get_page_size(); 00093 TEST_ASSERT_NOT_EQUAL(0, sector_size); 00094 TEST_ASSERT_NOT_EQUAL(0, page_size); 00095 TEST_ASSERT_TRUE(sector_size % page_size == 0); 00096 const uint8_t test_value = 0xCE; 00097 uint8_t *data = new uint8_t[page_size]; 00098 for (uint32_t i = 0; i < page_size; i++) { 00099 data[i] = test_value; 00100 } 00101 00102 // the one before the last page in the system 00103 uint32_t address = (flash_device.get_flash_start() + flash_device.get_flash_size()) - (sector_size); 00104 TEST_ASSERT_TRUE(address != 0UL); 00105 00106 // unaligned address 00107 ret = flash_device.erase(address + 1, sector_size); 00108 TEST_ASSERT_EQUAL_INT32(-1, ret); 00109 if (flash_device.get_page_size() > 1) { 00110 ret = flash_device.program(data, address + 1, page_size); 00111 TEST_ASSERT_EQUAL_INT32(-1, ret); 00112 } 00113 00114 if (flash_device.get_page_size() > 1) { 00115 // unaligned page size 00116 ret = flash_device.program(data, address, page_size + 1); 00117 TEST_ASSERT_EQUAL_INT32(-1, ret); 00118 } 00119 00120 delete[] data; 00121 00122 ret = flash_device.deinit(); 00123 TEST_ASSERT_EQUAL_INT32(0, ret); 00124 } 00125 00126 Case cases[] = { 00127 Case("FlashIAP - init", flashiap_init_test), 00128 Case("FlashIAP - program", flashiap_program_test), 00129 Case("FlashIAP - program errors", flashiap_program_error_test), 00130 }; 00131 00132 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) { 00133 GREENTEA_SETUP(20, "default_auto"); 00134 return greentea_test_setup_handler(number_of_cases); 00135 } 00136 00137 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00138 00139 int main() { 00140 Harness::run(specification); 00141 }
Generated on Sun Jul 17 2022 08:25:27 by
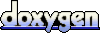