
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2013-2014 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 00020 class DevNull : public Stream { 00021 public: 00022 DevNull(const char *name = NULL) : Stream(name) {} 00023 00024 protected: 00025 virtual int _getc() { 00026 return 0; 00027 } 00028 virtual int _putc(int c) { 00029 return c; 00030 } 00031 }; 00032 00033 DevNull null("null"); 00034 00035 int main() { 00036 GREENTEA_SETUP(2, "dev_null_auto"); 00037 00038 printf("MBED: before re-routing stdout to /null\n"); // This shouldn't appear 00039 greentea_send_kv("to_stdout", "re-routing stdout to /null"); 00040 00041 if (freopen("/null", "w", stdout)) { 00042 // This shouldn't appear on serial 00043 // We should use pure printf here to send KV 00044 printf("{{to_null;printf redirected to /null}}\n"); 00045 printf("MBED: this printf is already redirected to /null\n"); 00046 } 00047 00048 while(1) { 00049 // Success is determined by the host test at this point, so busy wait 00050 } 00051 }
Generated on Sun Jul 17 2022 08:25:27 by
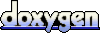