
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mac_mcps.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file mac_mcps.h 00016 * \brief MAC Common Part Sublayer API 00017 */ 00018 00019 #ifndef MAC_MCPS_H 00020 #define MAC_MCPS_H 00021 00022 #include <inttypes.h> 00023 #include "mac_common_defines.h" 00024 00025 /** 00026 * @brief struct mcps_data_req_t Data request structure 00027 * 00028 * See IEEE standard 802.15.4-2006 (table 41) for more details 00029 */ 00030 typedef struct mcps_data_req_s { 00031 unsigned SrcAddrMode:2; /**< Source address mode */ 00032 unsigned DstAddrMode:2; /**< Destination address mode */ 00033 uint16_t DstPANId; /**< Destination PAN ID */ 00034 uint8_t DstAddr[8]; /**< Destination address */ 00035 uint16_t msduLength; /**< Service data unit length */ 00036 uint8_t *msdu; /**< Service data unit */ 00037 uint8_t msduHandle; /**< Handle associated with MSDU */ 00038 bool TxAckReq: 1; /**< Specifies whether ACK is needed or not */ 00039 bool InDirectTx:1; /**< Specifies whether indirect or direct transmission is used */ 00040 bool PendingBit: 1; /**< Specifies whether more fragments are to be sent or not */ 00041 mlme_security_t Key; /**< Security key */ 00042 } mcps_data_req_t; 00043 00044 /** 00045 * @brief struct mcps_data_conf_t Data confirm structure 00046 * 00047 * See IEEE standard 802.15.4-2006 (table 42) for more details 00048 */ 00049 typedef struct mcps_data_conf_s { 00050 uint8_t msduHandle; /**< Handle associated with MSDU */ 00051 uint8_t status; /**< Status of the last MSDU transmission */ 00052 uint32_t timestamp; /**< Time, in symbols, at which the data were transmitted */ 00053 //Non-standard extension 00054 uint8_t cca_retries; /**< Number of CCA retries used during sending */ 00055 uint8_t tx_retries; /**< Number of retries done during sending, 0 means no retries */ 00056 } mcps_data_conf_t; 00057 00058 /** 00059 * @brief struct mcps_data_ind_t Data indication structure 00060 * 00061 * See IEEE standard 802.15.4-2006 (table 43) for more details 00062 */ 00063 typedef struct mcps_data_ind_s { 00064 unsigned SrcAddrMode:2; /**< 0x00 = no address 0x01 = reserved 0x02 = 16-bit short address 0x03 = 64-bit extended address */ 00065 uint16_t SrcPANId; /**< Source PAN ID */ 00066 uint8_t SrcAddr[8]; /**< Source address */ 00067 unsigned DstAddrMode:2; /**< Destination address mode */ 00068 uint16_t DstPANId; /**< Destination PAN ID */ 00069 uint8_t DstAddr[8]; /**< Destination address */ 00070 uint8_t mpduLinkQuality; /**< LQI value measured during reception of the MPDU */ 00071 int8_t signal_dbm; /**< This extension for normal IEEE 802.15.4 Data indication */ 00072 uint32_t timestamp; /**< The time, in symbols, at which the data were received */ 00073 uint8_t DSN; /**< Data sequence number */ 00074 mlme_security_t Key; /**< Security key */ 00075 uint16_t msduLength; /**< Data unit length */ 00076 uint8_t *msdu_ptr; /**< Data unit */ 00077 } mcps_data_ind_t; 00078 00079 /** 00080 * @brief struct mcps_purge_t Purge request structure 00081 * 00082 * See IEEE standard 802.15.4-2006 (table 44) for more details 00083 */ 00084 typedef struct mcps_purge_s { 00085 uint8_t msduHandle; /**< Handle associated with MSDU */ 00086 } mcps_purge_t; 00087 00088 /** 00089 * @brief struct mcps_purge_conf_t Purge confirm structure 00090 * 00091 * See IEEE standard 802.15.4-2006 (table 45) for more details 00092 */ 00093 typedef struct mcps_purge_conf_s { 00094 uint8_t msduHandle; /**< Handle associated with MSDU */ 00095 uint8_t status; /**< Status of the purge performed */ 00096 } mcps_purge_conf_t; 00097 00098 00099 #endif // MAC_MCPS_H
Generated on Sun Jul 17 2022 08:25:25 by
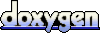