
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mac_filter_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file mac_filter_api.h 00017 * \brief API to allow filtering of packets based upon link quality and received power levels. 00018 * 00019 * \section app-mac-fil Applying a MAC filter 00020 * - mac_filter_start(), Starts a MAC level filter. 00021 * - mac_filter_add_short(), Adds a filter using short MAC address. 00022 * - mac_filter_add_long(), Adds a filter using long MAC address. 00023 * 00024 * \section rem-mac-fil Removing a MAC filter 00025 * - mac_filter_stop(), Stops MAC level filter and clears all defaults. 00026 * - mac_filter_clear(), Stops MAC level filter and leaves the default link configuration. 00027 * - mac_filter_delete_short(), Deletes filter for a device specified by short MAC address. 00028 * - mac_filter_delete_long(), Deletes filter for a device specified by long MAC address. 00029 * 00030 * \section reg-mac-fil Setting up address mapping for filter 00031 * - mac_filter_set_address_mapping(), Registers address mapping functions. 00032 * 00033 * \section macr-help Helper macros 00034 * - mac_filter_start(interface_id, MAC_FILTER_BLOCKED), Black list filter. 00035 * - mac_filter_start(interface_id, MAC_FILTER_ALLOWED), White list filter not modifying the qualities. 00036 * - mac_filter_start(interface_id, MAC_FILTER_FIXED(0x01, -80)), Fixed value for default link quality (poor quality). 00037 * - mac_filter_start(interface_id, MAC_FILTER_FIXED(0xff, -20)), Fixed value for default link quality (good quality). 00038 * - mac_filter_add_short(interface_id, 0x0001, MAC_FILTER_BLOCKED) 00039 * - mac_filter_add_short(interface_id, 0x0001, MAC_FILTER_ALLOWED) 00040 * - mac_filter_add_short(interface_id, 0x0001, MAC_FILTER_FIXED(0xff,-30)) 00041 * - mac_filter_add_long(interface_id, mac64, MAC_FILTER_BLOCKED) 00042 * - mac_filter_add_long(interface_id, mac64, MAC_FILTER_ALLOWED) 00043 * - mac_filter_add_long(interface_id, mac64, MAC_FILTER_FIXED(0x7f, -60)) 00044 */ 00045 00046 00047 #ifndef MAC_FILTER_API_H_ 00048 #define MAC_FILTER_API_H_ 00049 00050 #include "ns_types.h" 00051 00052 #ifdef __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 /** 00057 * \brief Start MAC level filter. 00058 * This function can be called again if default values are modified. 00059 * 00060 * 00061 * \param interface_id Network interface ID. 00062 * \param lqi_m LQI multiplier (8.8 Fixed point multiplier). 00063 * \param lqi_add Value added to LQI. 00064 * \param dbm_m DBM multiplier (8.8 Fixed point multiplier). 00065 * \param dbm_add Value added to DBM. 00066 * 00067 * \return 0, OK. 00068 * \return <0 Not OK. 00069 */ 00070 00071 int_fast8_t mac_filter_start(int8_t interface_id, int16_t lqi_m, int16_t lqi_add, int16_t dbm_m, int16_t dbm_add); 00072 00073 /** 00074 * \brief Stop MAC level filter. 00075 * 00076 * Stops the filtering module and clears the default settings and all the filterings made. 00077 * 00078 * \param interface_id Network interface ID. 00079 * 00080 * \return 0, OK. 00081 * \return <0 Not OK. 00082 */ 00083 00084 void mac_filter_stop(int8_t interface_id); 00085 00086 /** White list filter not modifying the qualities.*/ 00087 #define MAC_FILTER_ALLOWED 0x100, 0, 0x100, 0 00088 /** Black list filter.*/ 00089 #define MAC_FILTER_BLOCKED 0, 0, 0, 0 00090 /** Fixed value for default link quality.*/ 00091 #define MAC_FILTER_FIXED(lqi,dbm) 0, lqi, 0, dbm 00092 00093 /** 00094 * \brief Delete all filters. 00095 * 00096 * Leaves the default link configuration. 00097 * 00098 * \param interface_id Network interface ID. 00099 * 00100 * \return 0, OK. 00101 * \return <0 Not OK. 00102 */ 00103 int_fast8_t mac_filter_clear(int8_t interface_id); 00104 00105 /** 00106 * \brief Map the extended address to the short address. 00107 * 00108 * \param interface_id Network Interface. 00109 * \param mac64 Extended address. 00110 * \param mac16 Return the short address. 00111 * 00112 * \return 0, address resolved. 00113 * \return <0 No mapping found. 00114 */ 00115 typedef int_fast8_t (mac_filter_map_extented_to_short_cb)(int8_t interface_id, uint8_t mac64[8], uint16_t *mac16); 00116 00117 /** 00118 * \brief Map short address to extended address. 00119 * 00120 * \param interface_id Network interface ID. 00121 * \param mac64[out] Return buffer for the extended address. 00122 * \param mac16 Short address. 00123 * 00124 * return 0, address resolved. 00125 * return <0 no mapping found. 00126 */ 00127 typedef int_fast8_t (mac_filter_map_short_to_extended_cb)(int8_t interface_id, uint8_t mac64[8], uint16_t mac16); 00128 00129 /** 00130 * \brief Register address mapping functions. 00131 * 00132 * This function should be added for layer in stack that keeps up the list of address mapping functions. 00133 * If filters are enabled these functions are called if no match from filters was found. 00134 * 00135 * When this service is no longer provided call this function with NULL pointers. 00136 * 00137 * \param interface_id Network interface ID. 00138 * \param long_cb Address mapping to resolve long address from short address. 00139 * \param short_cb Address mapping to resolve short address from long address. 00140 * 00141 * \return 0, OK. 00142 * \return <0 Not OK. 00143 */ 00144 int_fast8_t mac_filter_set_address_mapping(int8_t interface_id, mac_filter_map_short_to_extended_cb *long_cb, mac_filter_map_extented_to_short_cb *short_cb); 00145 00146 /** 00147 * \brief Delete filter for device 00148 * 00149 * \param interface_id Network interface ID. 00150 * \param mac16 Short address. 00151 * 00152 * \return 0, OK. 00153 * \return <0 Not OK. 00154 */ 00155 int_fast8_t mac_filter_delete_short(int8_t interface_id, uint16_t mac16); 00156 00157 /** 00158 * \brief Delete filter for device. 00159 * 00160 * \param interface_id Network interface ID. 00161 * \param mac64 Long address. 00162 * 00163 * \return 0, OK 00164 * \return <0 Not OK 00165 */ 00166 int_fast8_t mac_filter_delete_long(int8_t interface_id, uint8_t mac64[8]); 00167 00168 /** 00169 * \brief Add MAC short address filter. 00170 * 00171 * \param interface_id Network interface ID. 00172 * \param mac16 Short address. 00173 * \param lqi_m LQI multiplier (8.8 Fixed point multiplier). 00174 * \param lqi_add Value added to LQI. 00175 * \param dbm_m DBM multiplier (8.8 Fixed point multiplier). 00176 * \param dbm_add Value added to DBM. 00177 * 00178 * \return 0, OK. 00179 * \return <0 Not OK. 00180 */ 00181 int_fast8_t mac_filter_add_short(int8_t interface_id, uint16_t mac16, int16_t lqi_m, int16_t lqi_add, int16_t dbm_m, int16_t dbm_add); 00182 00183 /** 00184 * \brief Add MAC long address filter. 00185 * 00186 * \param interface_id Network interface ID. 00187 * \param mac64 Long address. 00188 * \param lqi_m LQI multiplier (8.8 Fixed point multiplier). 00189 * \param lqi_add Value added to LQI. 00190 * \param dbm_m DBM multiplier (8.8 Fixed point multiplier). 00191 * \param dbm_add Value added to DBM. 00192 * 00193 * \return 0, OK. 00194 * \return <0 Not OK. 00195 */ 00196 int_fast8_t mac_filter_add_long(int8_t interface_id, uint8_t mac64[8], int16_t lqi_m, int16_t lqi_add, int16_t dbm_m, int16_t dbm_add); 00197 00198 #ifdef __cplusplus 00199 } 00200 #endif 00201 00202 #endif /* MAC_FILTER_API_H_ */
Generated on Sun Jul 17 2022 08:25:25 by
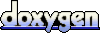