
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mac_common_defines.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file mac_common_defines.h 00016 * \brief Common definitions for MAC layer. 00017 */ 00018 00019 #ifndef MAC_COMMON_DEFINES_H_ 00020 #define MAC_COMMON_DEFINES_H_ 00021 00022 #include "ns_types.h" 00023 00024 #define MAC_ADDR_MODE_NONE 0 /**< Address mode for no address defined */ 00025 #define MAC_ADDR_MODE_16_BIT 2 /**< Address mode for 16-bit addresses */ 00026 #define MAC_ADDR_MODE_64_BIT 3 /**< Address mode for 64-bit addresses */ 00027 00028 #define MAC_FRAME_VERSION_2003 0 /**< FCF - IEEE 802.15.4-2003 compatible */ 00029 #define MAC_FRAME_VERSION_2006 1 /**< FCF - IEEE 802.15.4-2006 (big payload or new security) */ 00030 00031 //See IEEE standard 802.15.4-2006 (table 96) for more details about identifiers 00032 #define MAC_KEY_ID_MODE_IMPLICIT 0 /**< Key identifier mode implicit */ 00033 #define MAC_KEY_ID_MODE_IDX 1 /**< Key identifier mode for 1-octet key index */ 00034 #define MAC_KEY_ID_MODE_SRC4_IDX 2 /**< Key identifier mode for combined 4-octet key source and 1-octet key index */ 00035 #define MAC_KEY_ID_MODE_SRC8_IDX 3 /**< Key identifier mode for combined 8-octet key source and 1-octet key index */ 00036 00037 /* IEEE 802.15.4 constants */ 00038 #define MAC_IEEE_802_15_4_MAX_MPDU_UNSECURED_OVERHEAD 25 /**< Maximum for MAC protocol data unit unsecured overhead */ 00039 #define MAC_IEEE_802_15_4_MIN_MPDU_OVERHEAD 9 /**< Minimum overhead added by MAC to MPDU */ 00040 #define MAC_IEEE_802_15_4_MAX_BEACON_OVERHEAD 75 /**< Maximum overhead which is added by the MAC for beacon */ 00041 #define MAC_IEEE_802_15_4_MAX_PHY_PACKET_SIZE 127 /**< Maximum number of octets PHY layer is able to receive */ 00042 00043 #define MAC_IEEE_802_15_4_MAX_BEACON_PAYLOAD_LENGTH \ 00044 (MAC_IEEE_802_15_4_MAX_PHY_PACKET_SIZE - MAC_IEEE_802_15_4_MAX_BEACON_OVERHEAD) /**< Maximum size of beacon payload */ 00045 00046 /** Maximum number of bytes which can be transmitted in the MAC payload of an unsecured MAC frame */ 00047 #define MAC_IEEE_802_15_4_MAX_MAC_SAFE_PAYLOAD_SIZE \ 00048 (MAC_IEEE_802_15_4_MAX_PHY_PACKET_SIZE - MAC_IEEE_802_15_4_MAX_MPDU_UNSECURED_OVERHEAD) 00049 00050 #define mac_unsecured_2003_compatibility false /**< For IEEE 802.15.4-2003 MAC compatibility, force max size to never exceed MAC_IEEE_802_15_4_MAX_MAC_SAFE_PAYLOAD_SIZE */ 00051 00052 /** 00053 * @brief struct mlme_security_t MLME/MCPS security structure 00054 * This structure encapsulates security related variables, 00055 * which are always used together if SecurityLevel > 0. 00056 * 00057 * See IEEE standard 802.15.4-2006 (e.g end of table 41) for more details 00058 */ 00059 typedef struct mlme_security_s { 00060 unsigned SecurityLevel:3; /**< Security level */ 00061 unsigned KeyIdMode:2; /**< 2-bit value which define key source and ID use case */ 00062 uint8_t KeyIndex; /**< Key index */ 00063 uint8_t Keysource[8]; /**< Key source */ 00064 } mlme_security_t; 00065 00066 #endif /* MAC_COMMON_DEFINES_H_ */
Generated on Sun Jul 17 2022 08:25:25 by
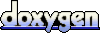