
Rtos API example
Sockets BSD-Like API module. More...
Go to the source code of this file.
Functions | |
static int | lwip_socket_register_membership (int s, const ip4_addr_t *if_addr, const ip4_addr_t *multi_addr) |
Register a new IGMP membership. | |
static void | lwip_socket_unregister_membership (int s, const ip4_addr_t *if_addr, const ip4_addr_t *multi_addr) |
Unregister a previously registered membership. | |
static void | lwip_socket_drop_registered_memberships (int s) |
Drop all memberships of a socket that were not dropped explicitly via setsockopt. | |
static void | event_callback (struct netconn *conn, enum netconn_evt evt, u16_t len) |
Callback registered in the netconn layer for each socket-netconn. | |
static void | lwip_getsockopt_callback (void *arg) |
lwip_getsockopt_callback: only used without CORE_LOCKING to get into the tcpip_thread | |
static void | lwip_setsockopt_callback (void *arg) |
lwip_setsockopt_callback: only used without CORE_LOCKING to get into the tcpip_thread | |
static u8_t | lwip_getsockopt_impl (int s, int level, int optname, void *optval, socklen_t *optlen) |
lwip_getsockopt_impl: the actual implementation of getsockopt: same argument as lwip_getsockopt, either called directly or through callback | |
static u8_t | lwip_setsockopt_impl (int s, int level, int optname, const void *optval, socklen_t optlen) |
lwip_setsockopt_impl: the actual implementation of setsockopt: same argument as lwip_setsockopt, either called directly or through callback | |
void | lwip_socket_thread_init (void) |
LWIP_NETCONN_SEM_PER_THREAD==1: initialize thread-local semaphore. | |
void | lwip_socket_thread_cleanup (void) |
LWIP_NETCONN_SEM_PER_THREAD==1: destroy thread-local semaphore. | |
static struct lwip_sock * | get_socket (int s) |
Map a externally used socket index to the internal socket representation. | |
static struct lwip_sock * | tryget_socket (int s) |
Same as get_socket but doesn't set errno. | |
static int | alloc_socket (struct netconn *newconn, int accepted) |
Allocate a new socket for a given netconn. | |
static void | free_socket (struct lwip_sock *sock, int is_tcp) |
Free a socket. | |
int | lwip_listen (int s, int backlog) |
Set a socket into listen mode. | |
static int | lwip_selscan (int maxfdp1, fd_set *readset_in, fd_set *writeset_in, fd_set *exceptset_in, fd_set *readset_out, fd_set *writeset_out, fd_set *exceptset_out) |
Go through the readset and writeset lists and see which socket of the sockets set in the sets has events. | |
int | lwip_shutdown (int s, int how) |
Close one end of a full-duplex connection. | |
int | lwip_fcntl (int s, int cmd, int val) |
A minimal implementation of fcntl. | |
Variables | |
static struct lwip_sock | sockets [NUM_SOCKETS] |
The global array of available sockets. | |
static struct lwip_select_cb * | select_cb_list |
The global list of tasks waiting for select. | |
static volatile int | select_cb_ctr |
This counter is increased from lwip_select when the list is changed and checked in event_callback to see if it has changed. |
Detailed Description
Sockets BSD-Like API module.
Definition in file lwip_sockets.c.
Function Documentation
static int alloc_socket | ( | struct netconn * | newconn, |
int | accepted | ||
) | [static] |
Allocate a new socket for a given netconn.
- Parameters:
-
newconn the netconn for which to allocate a socket accepted 1 if socket has been created by accept(), 0 if socket has been created by socket()
- Returns:
- the index of the new socket; -1 on error
Definition at line 401 of file lwip_sockets.c.
static void event_callback | ( | struct netconn * | conn, |
enum netconn_evt | evt, | ||
u16_t | len | ||
) | [static] |
Callback registered in the netconn layer for each socket-netconn.
Processes recvevent (data available) and wakes up tasks waiting for select.
Definition at line 1571 of file lwip_sockets.c.
static void free_socket | ( | struct lwip_sock * | sock, |
int | is_tcp | ||
) | [static] |
Free a socket.
The socket's netconn must have been delete before!
- Parameters:
-
sock the socket to free is_tcp != 0 for TCP sockets, used to free lastdata
Definition at line 437 of file lwip_sockets.c.
static struct lwip_sock* get_socket | ( | int | s ) | [static, read] |
Map a externally used socket index to the internal socket representation.
- Parameters:
-
s externally used socket index
- Returns:
- struct lwip_sock for the socket or NULL if not found
Definition at line 350 of file lwip_sockets.c.
int lwip_fcntl | ( | int | s, |
int | cmd, | ||
int | val | ||
) |
A minimal implementation of fcntl.
Currently only the commands F_GETFL and F_SETFL are implemented. Only the flag O_NONBLOCK is implemented.
Definition at line 2712 of file lwip_sockets.c.
static void lwip_getsockopt_callback | ( | void * | arg ) | [static] |
lwip_getsockopt_callback: only used without CORE_LOCKING to get into the tcpip_thread
Definition at line 1865 of file lwip_sockets.c.
static u8_t lwip_getsockopt_impl | ( | int | s, |
int | level, | ||
int | optname, | ||
void * | optval, | ||
socklen_t * | optlen | ||
) | [static] |
lwip_getsockopt_impl: the actual implementation of getsockopt: same argument as lwip_getsockopt, either called directly or through callback
Definition at line 1887 of file lwip_sockets.c.
int lwip_listen | ( | int | s, |
int | backlog | ||
) |
Set a socket into listen mode.
The socket may not have been used for another connection previously.
- Parameters:
-
s the socket to set to listening mode backlog (ATTENTION: needs TCP_LISTEN_BACKLOG=1)
- Returns:
- 0 on success, non-zero on failure
Definition at line 709 of file lwip_sockets.c.
static int lwip_selscan | ( | int | maxfdp1, |
fd_set * | readset_in, | ||
fd_set * | writeset_in, | ||
fd_set * | exceptset_in, | ||
fd_set * | readset_out, | ||
fd_set * | writeset_out, | ||
fd_set * | exceptset_out | ||
) | [static] |
Go through the readset and writeset lists and see which socket of the sockets set in the sets has events.
On return, readset, writeset and exceptset have the sockets enabled that had events.
- Parameters:
-
maxfdp1 the highest socket index in the sets readset_in set of sockets to check for read events writeset_in set of sockets to check for write events exceptset_in set of sockets to check for error events readset_out set of sockets that had read events writeset_out set of sockets that had write events exceptset_out set os sockets that had error events
- Returns:
- number of sockets that had events (read/write/exception) (>= 0)
Definition at line 1306 of file lwip_sockets.c.
static void lwip_setsockopt_callback | ( | void * | arg ) | [static] |
lwip_setsockopt_callback: only used without CORE_LOCKING to get into the tcpip_thread
Definition at line 2266 of file lwip_sockets.c.
static u8_t lwip_setsockopt_impl | ( | int | s, |
int | level, | ||
int | optname, | ||
const void * | optval, | ||
socklen_t | optlen | ||
) | [static] |
lwip_setsockopt_impl: the actual implementation of setsockopt: same argument as lwip_setsockopt, either called directly or through callback
Definition at line 2288 of file lwip_sockets.c.
int lwip_shutdown | ( | int | s, |
int | how | ||
) |
Close one end of a full-duplex connection.
Definition at line 1691 of file lwip_sockets.c.
static void lwip_socket_drop_registered_memberships | ( | int | s ) | [static] |
Drop all memberships of a socket that were not dropped explicitly via setsockopt.
ATTENTION: this function is NOT called from tcpip_thread (or under CORE_LOCK).
Definition at line 2804 of file lwip_sockets.c.
static int lwip_socket_register_membership | ( | int | s, |
const ip4_addr_t * | if_addr, | ||
const ip4_addr_t * | multi_addr | ||
) | [static] |
Register a new IGMP membership.
On socket close, the membership is dropped automatically.
ATTENTION: this function is called from tcpip_thread (or under CORE_LOCK).
- Returns:
- 1 on success, 0 on failure
Definition at line 2752 of file lwip_sockets.c.
void lwip_socket_thread_cleanup | ( | void | ) |
LWIP_NETCONN_SEM_PER_THREAD==1: destroy thread-local semaphore.
Definition at line 338 of file lwip_sockets.c.
void lwip_socket_thread_init | ( | void | ) |
LWIP_NETCONN_SEM_PER_THREAD==1: initialize thread-local semaphore.
Definition at line 331 of file lwip_sockets.c.
static void lwip_socket_unregister_membership | ( | int | s, |
const ip4_addr_t * | if_addr, | ||
const ip4_addr_t * | multi_addr | ||
) | [static] |
Unregister a previously registered membership.
This prevents dropping the membership on socket close.
ATTENTION: this function is called from tcpip_thread (or under CORE_LOCK).
Definition at line 2778 of file lwip_sockets.c.
static struct lwip_sock* tryget_socket | ( | int | s ) | [static, read] |
Same as get_socket but doesn't set errno.
- Parameters:
-
s externally used socket index
- Returns:
- struct lwip_sock for the socket or NULL if not found
Definition at line 380 of file lwip_sockets.c.
Variable Documentation
volatile int select_cb_ctr [static] |
This counter is increased from lwip_select when the list is changed and checked in event_callback to see if it has changed.
Definition at line 290 of file lwip_sockets.c.
struct lwip_select_cb* select_cb_list [static] |
The global list of tasks waiting for select.
Definition at line 287 of file lwip_sockets.c.
struct lwip_sock sockets[NUM_SOCKETS] [static] |
The global array of available sockets.
Definition at line 285 of file lwip_sockets.c.
Generated on Sun Jul 17 2022 08:25:35 by
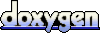