
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_des.c
00001 /* 00002 * FIPS-46-3 compliant Triple-DES implementation 00003 * 00004 * Based on XySSL: Copyright (C) 2006-2008 Christophe Devine 00005 * 00006 * Copyright (C) 2009 Paul Bakker <polarssl_maintainer at polarssl dot org> 00007 * 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without 00011 * modification, are permitted provided that the following conditions 00012 * are met: 00013 * 00014 * * Redistributions of source code must retain the above copyright 00015 * notice, this list of conditions and the following disclaimer. 00016 * * Redistributions in binary form must reproduce the above copyright 00017 * notice, this list of conditions and the following disclaimer in the 00018 * documentation and/or other materials provided with the distribution. 00019 * * Neither the names of PolarSSL or XySSL nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00024 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00025 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00026 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00027 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00028 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED 00029 * TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00030 * PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00031 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00032 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /* 00036 * DES, on which TDES is based, was originally designed by Horst Feistel 00037 * at IBM in 1974, and was adopted as a standard by NIST (formerly NBS). 00038 * 00039 * http://csrc.nist.gov/publications/fips/fips46-3/fips46-3.pdf 00040 */ 00041 00042 #include "netif/ppp/ppp_opts.h" 00043 #if PPP_SUPPORT && LWIP_INCLUDED_POLARSSL_DES 00044 00045 #include "netif/ppp/polarssl/des.h" 00046 00047 /* 00048 * 32-bit integer manipulation macros (big endian) 00049 */ 00050 #ifndef GET_ULONG_BE 00051 #define GET_ULONG_BE(n,b,i) \ 00052 { \ 00053 (n) = ( (unsigned long) (b)[(i) ] << 24 ) \ 00054 | ( (unsigned long) (b)[(i) + 1] << 16 ) \ 00055 | ( (unsigned long) (b)[(i) + 2] << 8 ) \ 00056 | ( (unsigned long) (b)[(i) + 3] ); \ 00057 } 00058 #endif 00059 00060 #ifndef PUT_ULONG_BE 00061 #define PUT_ULONG_BE(n,b,i) \ 00062 { \ 00063 (b)[(i) ] = (unsigned char) ( (n) >> 24 ); \ 00064 (b)[(i) + 1] = (unsigned char) ( (n) >> 16 ); \ 00065 (b)[(i) + 2] = (unsigned char) ( (n) >> 8 ); \ 00066 (b)[(i) + 3] = (unsigned char) ( (n) ); \ 00067 } 00068 #endif 00069 00070 /* 00071 * Expanded DES S-boxes 00072 */ 00073 static const unsigned long SB1[64] = 00074 { 00075 0x01010400, 0x00000000, 0x00010000, 0x01010404, 00076 0x01010004, 0x00010404, 0x00000004, 0x00010000, 00077 0x00000400, 0x01010400, 0x01010404, 0x00000400, 00078 0x01000404, 0x01010004, 0x01000000, 0x00000004, 00079 0x00000404, 0x01000400, 0x01000400, 0x00010400, 00080 0x00010400, 0x01010000, 0x01010000, 0x01000404, 00081 0x00010004, 0x01000004, 0x01000004, 0x00010004, 00082 0x00000000, 0x00000404, 0x00010404, 0x01000000, 00083 0x00010000, 0x01010404, 0x00000004, 0x01010000, 00084 0x01010400, 0x01000000, 0x01000000, 0x00000400, 00085 0x01010004, 0x00010000, 0x00010400, 0x01000004, 00086 0x00000400, 0x00000004, 0x01000404, 0x00010404, 00087 0x01010404, 0x00010004, 0x01010000, 0x01000404, 00088 0x01000004, 0x00000404, 0x00010404, 0x01010400, 00089 0x00000404, 0x01000400, 0x01000400, 0x00000000, 00090 0x00010004, 0x00010400, 0x00000000, 0x01010004 00091 }; 00092 00093 static const unsigned long SB2[64] = 00094 { 00095 0x80108020, 0x80008000, 0x00008000, 0x00108020, 00096 0x00100000, 0x00000020, 0x80100020, 0x80008020, 00097 0x80000020, 0x80108020, 0x80108000, 0x80000000, 00098 0x80008000, 0x00100000, 0x00000020, 0x80100020, 00099 0x00108000, 0x00100020, 0x80008020, 0x00000000, 00100 0x80000000, 0x00008000, 0x00108020, 0x80100000, 00101 0x00100020, 0x80000020, 0x00000000, 0x00108000, 00102 0x00008020, 0x80108000, 0x80100000, 0x00008020, 00103 0x00000000, 0x00108020, 0x80100020, 0x00100000, 00104 0x80008020, 0x80100000, 0x80108000, 0x00008000, 00105 0x80100000, 0x80008000, 0x00000020, 0x80108020, 00106 0x00108020, 0x00000020, 0x00008000, 0x80000000, 00107 0x00008020, 0x80108000, 0x00100000, 0x80000020, 00108 0x00100020, 0x80008020, 0x80000020, 0x00100020, 00109 0x00108000, 0x00000000, 0x80008000, 0x00008020, 00110 0x80000000, 0x80100020, 0x80108020, 0x00108000 00111 }; 00112 00113 static const unsigned long SB3[64] = 00114 { 00115 0x00000208, 0x08020200, 0x00000000, 0x08020008, 00116 0x08000200, 0x00000000, 0x00020208, 0x08000200, 00117 0x00020008, 0x08000008, 0x08000008, 0x00020000, 00118 0x08020208, 0x00020008, 0x08020000, 0x00000208, 00119 0x08000000, 0x00000008, 0x08020200, 0x00000200, 00120 0x00020200, 0x08020000, 0x08020008, 0x00020208, 00121 0x08000208, 0x00020200, 0x00020000, 0x08000208, 00122 0x00000008, 0x08020208, 0x00000200, 0x08000000, 00123 0x08020200, 0x08000000, 0x00020008, 0x00000208, 00124 0x00020000, 0x08020200, 0x08000200, 0x00000000, 00125 0x00000200, 0x00020008, 0x08020208, 0x08000200, 00126 0x08000008, 0x00000200, 0x00000000, 0x08020008, 00127 0x08000208, 0x00020000, 0x08000000, 0x08020208, 00128 0x00000008, 0x00020208, 0x00020200, 0x08000008, 00129 0x08020000, 0x08000208, 0x00000208, 0x08020000, 00130 0x00020208, 0x00000008, 0x08020008, 0x00020200 00131 }; 00132 00133 static const unsigned long SB4[64] = 00134 { 00135 0x00802001, 0x00002081, 0x00002081, 0x00000080, 00136 0x00802080, 0x00800081, 0x00800001, 0x00002001, 00137 0x00000000, 0x00802000, 0x00802000, 0x00802081, 00138 0x00000081, 0x00000000, 0x00800080, 0x00800001, 00139 0x00000001, 0x00002000, 0x00800000, 0x00802001, 00140 0x00000080, 0x00800000, 0x00002001, 0x00002080, 00141 0x00800081, 0x00000001, 0x00002080, 0x00800080, 00142 0x00002000, 0x00802080, 0x00802081, 0x00000081, 00143 0x00800080, 0x00800001, 0x00802000, 0x00802081, 00144 0x00000081, 0x00000000, 0x00000000, 0x00802000, 00145 0x00002080, 0x00800080, 0x00800081, 0x00000001, 00146 0x00802001, 0x00002081, 0x00002081, 0x00000080, 00147 0x00802081, 0x00000081, 0x00000001, 0x00002000, 00148 0x00800001, 0x00002001, 0x00802080, 0x00800081, 00149 0x00002001, 0x00002080, 0x00800000, 0x00802001, 00150 0x00000080, 0x00800000, 0x00002000, 0x00802080 00151 }; 00152 00153 static const unsigned long SB5[64] = 00154 { 00155 0x00000100, 0x02080100, 0x02080000, 0x42000100, 00156 0x00080000, 0x00000100, 0x40000000, 0x02080000, 00157 0x40080100, 0x00080000, 0x02000100, 0x40080100, 00158 0x42000100, 0x42080000, 0x00080100, 0x40000000, 00159 0x02000000, 0x40080000, 0x40080000, 0x00000000, 00160 0x40000100, 0x42080100, 0x42080100, 0x02000100, 00161 0x42080000, 0x40000100, 0x00000000, 0x42000000, 00162 0x02080100, 0x02000000, 0x42000000, 0x00080100, 00163 0x00080000, 0x42000100, 0x00000100, 0x02000000, 00164 0x40000000, 0x02080000, 0x42000100, 0x40080100, 00165 0x02000100, 0x40000000, 0x42080000, 0x02080100, 00166 0x40080100, 0x00000100, 0x02000000, 0x42080000, 00167 0x42080100, 0x00080100, 0x42000000, 0x42080100, 00168 0x02080000, 0x00000000, 0x40080000, 0x42000000, 00169 0x00080100, 0x02000100, 0x40000100, 0x00080000, 00170 0x00000000, 0x40080000, 0x02080100, 0x40000100 00171 }; 00172 00173 static const unsigned long SB6[64] = 00174 { 00175 0x20000010, 0x20400000, 0x00004000, 0x20404010, 00176 0x20400000, 0x00000010, 0x20404010, 0x00400000, 00177 0x20004000, 0x00404010, 0x00400000, 0x20000010, 00178 0x00400010, 0x20004000, 0x20000000, 0x00004010, 00179 0x00000000, 0x00400010, 0x20004010, 0x00004000, 00180 0x00404000, 0x20004010, 0x00000010, 0x20400010, 00181 0x20400010, 0x00000000, 0x00404010, 0x20404000, 00182 0x00004010, 0x00404000, 0x20404000, 0x20000000, 00183 0x20004000, 0x00000010, 0x20400010, 0x00404000, 00184 0x20404010, 0x00400000, 0x00004010, 0x20000010, 00185 0x00400000, 0x20004000, 0x20000000, 0x00004010, 00186 0x20000010, 0x20404010, 0x00404000, 0x20400000, 00187 0x00404010, 0x20404000, 0x00000000, 0x20400010, 00188 0x00000010, 0x00004000, 0x20400000, 0x00404010, 00189 0x00004000, 0x00400010, 0x20004010, 0x00000000, 00190 0x20404000, 0x20000000, 0x00400010, 0x20004010 00191 }; 00192 00193 static const unsigned long SB7[64] = 00194 { 00195 0x00200000, 0x04200002, 0x04000802, 0x00000000, 00196 0x00000800, 0x04000802, 0x00200802, 0x04200800, 00197 0x04200802, 0x00200000, 0x00000000, 0x04000002, 00198 0x00000002, 0x04000000, 0x04200002, 0x00000802, 00199 0x04000800, 0x00200802, 0x00200002, 0x04000800, 00200 0x04000002, 0x04200000, 0x04200800, 0x00200002, 00201 0x04200000, 0x00000800, 0x00000802, 0x04200802, 00202 0x00200800, 0x00000002, 0x04000000, 0x00200800, 00203 0x04000000, 0x00200800, 0x00200000, 0x04000802, 00204 0x04000802, 0x04200002, 0x04200002, 0x00000002, 00205 0x00200002, 0x04000000, 0x04000800, 0x00200000, 00206 0x04200800, 0x00000802, 0x00200802, 0x04200800, 00207 0x00000802, 0x04000002, 0x04200802, 0x04200000, 00208 0x00200800, 0x00000000, 0x00000002, 0x04200802, 00209 0x00000000, 0x00200802, 0x04200000, 0x00000800, 00210 0x04000002, 0x04000800, 0x00000800, 0x00200002 00211 }; 00212 00213 static const unsigned long SB8[64] = 00214 { 00215 0x10001040, 0x00001000, 0x00040000, 0x10041040, 00216 0x10000000, 0x10001040, 0x00000040, 0x10000000, 00217 0x00040040, 0x10040000, 0x10041040, 0x00041000, 00218 0x10041000, 0x00041040, 0x00001000, 0x00000040, 00219 0x10040000, 0x10000040, 0x10001000, 0x00001040, 00220 0x00041000, 0x00040040, 0x10040040, 0x10041000, 00221 0x00001040, 0x00000000, 0x00000000, 0x10040040, 00222 0x10000040, 0x10001000, 0x00041040, 0x00040000, 00223 0x00041040, 0x00040000, 0x10041000, 0x00001000, 00224 0x00000040, 0x10040040, 0x00001000, 0x00041040, 00225 0x10001000, 0x00000040, 0x10000040, 0x10040000, 00226 0x10040040, 0x10000000, 0x00040000, 0x10001040, 00227 0x00000000, 0x10041040, 0x00040040, 0x10000040, 00228 0x10040000, 0x10001000, 0x10001040, 0x00000000, 00229 0x10041040, 0x00041000, 0x00041000, 0x00001040, 00230 0x00001040, 0x00040040, 0x10000000, 0x10041000 00231 }; 00232 00233 /* 00234 * PC1: left and right halves bit-swap 00235 */ 00236 static const unsigned long LHs[16] = 00237 { 00238 0x00000000, 0x00000001, 0x00000100, 0x00000101, 00239 0x00010000, 0x00010001, 0x00010100, 0x00010101, 00240 0x01000000, 0x01000001, 0x01000100, 0x01000101, 00241 0x01010000, 0x01010001, 0x01010100, 0x01010101 00242 }; 00243 00244 static const unsigned long RHs[16] = 00245 { 00246 0x00000000, 0x01000000, 0x00010000, 0x01010000, 00247 0x00000100, 0x01000100, 0x00010100, 0x01010100, 00248 0x00000001, 0x01000001, 0x00010001, 0x01010001, 00249 0x00000101, 0x01000101, 0x00010101, 0x01010101, 00250 }; 00251 00252 /* 00253 * Initial Permutation macro 00254 */ 00255 #define DES_IP(X,Y) \ 00256 { \ 00257 T = ((X >> 4) ^ Y) & 0x0F0F0F0F; Y ^= T; X ^= (T << 4); \ 00258 T = ((X >> 16) ^ Y) & 0x0000FFFF; Y ^= T; X ^= (T << 16); \ 00259 T = ((Y >> 2) ^ X) & 0x33333333; X ^= T; Y ^= (T << 2); \ 00260 T = ((Y >> 8) ^ X) & 0x00FF00FF; X ^= T; Y ^= (T << 8); \ 00261 Y = ((Y << 1) | (Y >> 31)) & 0xFFFFFFFF; \ 00262 T = (X ^ Y) & 0xAAAAAAAA; Y ^= T; X ^= T; \ 00263 X = ((X << 1) | (X >> 31)) & 0xFFFFFFFF; \ 00264 } 00265 00266 /* 00267 * Final Permutation macro 00268 */ 00269 #define DES_FP(X,Y) \ 00270 { \ 00271 X = ((X << 31) | (X >> 1)) & 0xFFFFFFFF; \ 00272 T = (X ^ Y) & 0xAAAAAAAA; X ^= T; Y ^= T; \ 00273 Y = ((Y << 31) | (Y >> 1)) & 0xFFFFFFFF; \ 00274 T = ((Y >> 8) ^ X) & 0x00FF00FF; X ^= T; Y ^= (T << 8); \ 00275 T = ((Y >> 2) ^ X) & 0x33333333; X ^= T; Y ^= (T << 2); \ 00276 T = ((X >> 16) ^ Y) & 0x0000FFFF; Y ^= T; X ^= (T << 16); \ 00277 T = ((X >> 4) ^ Y) & 0x0F0F0F0F; Y ^= T; X ^= (T << 4); \ 00278 } 00279 00280 /* 00281 * DES round macro 00282 */ 00283 #define DES_ROUND(X,Y) \ 00284 { \ 00285 T = *SK++ ^ X; \ 00286 Y ^= SB8[ (T ) & 0x3F ] ^ \ 00287 SB6[ (T >> 8) & 0x3F ] ^ \ 00288 SB4[ (T >> 16) & 0x3F ] ^ \ 00289 SB2[ (T >> 24) & 0x3F ]; \ 00290 \ 00291 T = *SK++ ^ ((X << 28) | (X >> 4)); \ 00292 Y ^= SB7[ (T ) & 0x3F ] ^ \ 00293 SB5[ (T >> 8) & 0x3F ] ^ \ 00294 SB3[ (T >> 16) & 0x3F ] ^ \ 00295 SB1[ (T >> 24) & 0x3F ]; \ 00296 } 00297 00298 #define SWAP(a,b) { unsigned long t = a; a = b; b = t; t = 0; } 00299 00300 static void des_setkey( unsigned long SK[32], unsigned char key[8] ) 00301 { 00302 int i; 00303 unsigned long X, Y, T; 00304 00305 GET_ULONG_BE( X, key, 0 ); 00306 GET_ULONG_BE( Y, key, 4 ); 00307 00308 /* 00309 * Permuted Choice 1 00310 */ 00311 T = ((Y >> 4) ^ X) & 0x0F0F0F0F; X ^= T; Y ^= (T << 4); 00312 T = ((Y ) ^ X) & 0x10101010; X ^= T; Y ^= (T ); 00313 00314 X = (LHs[ (X ) & 0xF] << 3) | (LHs[ (X >> 8) & 0xF ] << 2) 00315 | (LHs[ (X >> 16) & 0xF] << 1) | (LHs[ (X >> 24) & 0xF ] ) 00316 | (LHs[ (X >> 5) & 0xF] << 7) | (LHs[ (X >> 13) & 0xF ] << 6) 00317 | (LHs[ (X >> 21) & 0xF] << 5) | (LHs[ (X >> 29) & 0xF ] << 4); 00318 00319 Y = (RHs[ (Y >> 1) & 0xF] << 3) | (RHs[ (Y >> 9) & 0xF ] << 2) 00320 | (RHs[ (Y >> 17) & 0xF] << 1) | (RHs[ (Y >> 25) & 0xF ] ) 00321 | (RHs[ (Y >> 4) & 0xF] << 7) | (RHs[ (Y >> 12) & 0xF ] << 6) 00322 | (RHs[ (Y >> 20) & 0xF] << 5) | (RHs[ (Y >> 28) & 0xF ] << 4); 00323 00324 X &= 0x0FFFFFFF; 00325 Y &= 0x0FFFFFFF; 00326 00327 /* 00328 * calculate subkeys 00329 */ 00330 for( i = 0; i < 16; i++ ) 00331 { 00332 if( i < 2 || i == 8 || i == 15 ) 00333 { 00334 X = ((X << 1) | (X >> 27)) & 0x0FFFFFFF; 00335 Y = ((Y << 1) | (Y >> 27)) & 0x0FFFFFFF; 00336 } 00337 else 00338 { 00339 X = ((X << 2) | (X >> 26)) & 0x0FFFFFFF; 00340 Y = ((Y << 2) | (Y >> 26)) & 0x0FFFFFFF; 00341 } 00342 00343 *SK++ = ((X << 4) & 0x24000000) | ((X << 28) & 0x10000000) 00344 | ((X << 14) & 0x08000000) | ((X << 18) & 0x02080000) 00345 | ((X << 6) & 0x01000000) | ((X << 9) & 0x00200000) 00346 | ((X >> 1) & 0x00100000) | ((X << 10) & 0x00040000) 00347 | ((X << 2) & 0x00020000) | ((X >> 10) & 0x00010000) 00348 | ((Y >> 13) & 0x00002000) | ((Y >> 4) & 0x00001000) 00349 | ((Y << 6) & 0x00000800) | ((Y >> 1) & 0x00000400) 00350 | ((Y >> 14) & 0x00000200) | ((Y ) & 0x00000100) 00351 | ((Y >> 5) & 0x00000020) | ((Y >> 10) & 0x00000010) 00352 | ((Y >> 3) & 0x00000008) | ((Y >> 18) & 0x00000004) 00353 | ((Y >> 26) & 0x00000002) | ((Y >> 24) & 0x00000001); 00354 00355 *SK++ = ((X << 15) & 0x20000000) | ((X << 17) & 0x10000000) 00356 | ((X << 10) & 0x08000000) | ((X << 22) & 0x04000000) 00357 | ((X >> 2) & 0x02000000) | ((X << 1) & 0x01000000) 00358 | ((X << 16) & 0x00200000) | ((X << 11) & 0x00100000) 00359 | ((X << 3) & 0x00080000) | ((X >> 6) & 0x00040000) 00360 | ((X << 15) & 0x00020000) | ((X >> 4) & 0x00010000) 00361 | ((Y >> 2) & 0x00002000) | ((Y << 8) & 0x00001000) 00362 | ((Y >> 14) & 0x00000808) | ((Y >> 9) & 0x00000400) 00363 | ((Y ) & 0x00000200) | ((Y << 7) & 0x00000100) 00364 | ((Y >> 7) & 0x00000020) | ((Y >> 3) & 0x00000011) 00365 | ((Y << 2) & 0x00000004) | ((Y >> 21) & 0x00000002); 00366 } 00367 } 00368 00369 /* 00370 * DES key schedule (56-bit, encryption) 00371 */ 00372 void des_setkey_enc( des_context *ctx, unsigned char key[8] ) 00373 { 00374 des_setkey( ctx->sk , key ); 00375 } 00376 00377 /* 00378 * DES key schedule (56-bit, decryption) 00379 */ 00380 void des_setkey_dec( des_context *ctx, unsigned char key[8] ) 00381 { 00382 int i; 00383 00384 des_setkey( ctx->sk , key ); 00385 00386 for( i = 0; i < 16; i += 2 ) 00387 { 00388 SWAP( ctx->sk [i ], ctx->sk [30 - i] ); 00389 SWAP( ctx->sk [i + 1], ctx->sk [31 - i] ); 00390 } 00391 } 00392 00393 /* 00394 * DES-ECB block encryption/decryption 00395 */ 00396 void des_crypt_ecb( des_context *ctx, 00397 const unsigned char input[8], 00398 unsigned char output[8] ) 00399 { 00400 int i; 00401 unsigned long X, Y, T, *SK; 00402 00403 SK = ctx->sk ; 00404 00405 GET_ULONG_BE( X, input, 0 ); 00406 GET_ULONG_BE( Y, input, 4 ); 00407 00408 DES_IP( X, Y ); 00409 00410 for( i = 0; i < 8; i++ ) 00411 { 00412 DES_ROUND( Y, X ); 00413 DES_ROUND( X, Y ); 00414 } 00415 00416 DES_FP( Y, X ); 00417 00418 PUT_ULONG_BE( Y, output, 0 ); 00419 PUT_ULONG_BE( X, output, 4 ); 00420 } 00421 00422 #endif /* PPP_SUPPORT && LWIP_INCLUDED_POLARSSL_DES */
Generated on Sun Jul 17 2022 08:25:24 by
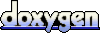