
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_ethernet.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Ethernet protocol definitions 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 #ifndef LWIP_HDR_PROT_ETHERNET_H 00038 #define LWIP_HDR_PROT_ETHERNET_H 00039 00040 #include "lwip/arch.h" 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 #ifndef ETH_HWADDR_LEN 00047 #ifdef ETHARP_HWADDR_LEN 00048 #define ETH_HWADDR_LEN ETHARP_HWADDR_LEN /* compatibility mode */ 00049 #else 00050 #define ETH_HWADDR_LEN 6 00051 #endif 00052 #endif 00053 00054 #ifdef PACK_STRUCT_USE_INCLUDES 00055 # include "arch/bpstruct.h" 00056 #endif 00057 PACK_STRUCT_BEGIN 00058 struct eth_addr { 00059 PACK_STRUCT_FLD_8(u8_t addr[ETH_HWADDR_LEN]); 00060 } PACK_STRUCT_STRUCT; 00061 PACK_STRUCT_END 00062 #ifdef PACK_STRUCT_USE_INCLUDES 00063 # include "arch/epstruct.h" 00064 #endif 00065 00066 #ifdef PACK_STRUCT_USE_INCLUDES 00067 # include "arch/bpstruct.h" 00068 #endif 00069 PACK_STRUCT_BEGIN 00070 /** Ethernet header */ 00071 struct eth_hdr { 00072 #if ETH_PAD_SIZE 00073 PACK_STRUCT_FLD_8(u8_t padding[ETH_PAD_SIZE]); 00074 #endif 00075 PACK_STRUCT_FLD_S(struct eth_addr dest); 00076 PACK_STRUCT_FLD_S(struct eth_addr src); 00077 PACK_STRUCT_FIELD(u16_t type); 00078 } PACK_STRUCT_STRUCT; 00079 PACK_STRUCT_END 00080 #ifdef PACK_STRUCT_USE_INCLUDES 00081 # include "arch/epstruct.h" 00082 #endif 00083 00084 #define SIZEOF_ETH_HDR (14 + ETH_PAD_SIZE) 00085 00086 #ifdef PACK_STRUCT_USE_INCLUDES 00087 # include "arch/bpstruct.h" 00088 #endif 00089 PACK_STRUCT_BEGIN 00090 /** VLAN header inserted between ethernet header and payload 00091 * if 'type' in ethernet header is ETHTYPE_VLAN. 00092 * See IEEE802.Q */ 00093 struct eth_vlan_hdr { 00094 PACK_STRUCT_FIELD(u16_t prio_vid); 00095 PACK_STRUCT_FIELD(u16_t tpid); 00096 } PACK_STRUCT_STRUCT; 00097 PACK_STRUCT_END 00098 #ifdef PACK_STRUCT_USE_INCLUDES 00099 # include "arch/epstruct.h" 00100 #endif 00101 00102 #define SIZEOF_VLAN_HDR 4 00103 #define VLAN_ID(vlan_hdr) (lwip_htons((vlan_hdr)->prio_vid) & 0xFFF) 00104 00105 /** 00106 * @ingroup ethernet 00107 * A list of often ethtypes (although lwIP does not use all of them): */ 00108 enum eth_type { 00109 /** Internet protocol v4 */ 00110 ETHTYPE_IP = 0x0800U, 00111 /** Address resolution protocol */ 00112 ETHTYPE_ARP = 0x0806U, 00113 /** Wake on lan */ 00114 ETHTYPE_WOL = 0x0842U, 00115 /** RARP */ 00116 ETHTYPE_RARP = 0x8035U, 00117 /** Virtual local area network */ 00118 ETHTYPE_VLAN = 0x8100U, 00119 /** Internet protocol v6 */ 00120 ETHTYPE_IPV6 = 0x86DDU, 00121 /** PPP Over Ethernet Discovery Stage */ 00122 ETHTYPE_PPPOEDISC = 0x8863U, 00123 /** PPP Over Ethernet Session Stage */ 00124 ETHTYPE_PPPOE = 0x8864U, 00125 /** Jumbo Frames */ 00126 ETHTYPE_JUMBO = 0x8870U, 00127 /** Process field network */ 00128 ETHTYPE_PROFINET = 0x8892U, 00129 /** Ethernet for control automation technology */ 00130 ETHTYPE_ETHERCAT = 0x88A4U, 00131 /** Link layer discovery protocol */ 00132 ETHTYPE_LLDP = 0x88CCU, 00133 /** Serial real-time communication system */ 00134 ETHTYPE_SERCOS = 0x88CDU, 00135 /** Media redundancy protocol */ 00136 ETHTYPE_MRP = 0x88E3U, 00137 /** Precision time protocol */ 00138 ETHTYPE_PTP = 0x88F7U, 00139 /** Q-in-Q, 802.1ad */ 00140 ETHTYPE_QINQ = 0x9100U 00141 }; 00142 00143 /** The 24-bit IANA IPv4-multicast OUI is 01-00-5e: */ 00144 #define LL_IP4_MULTICAST_ADDR_0 0x01 00145 #define LL_IP4_MULTICAST_ADDR_1 0x00 00146 #define LL_IP4_MULTICAST_ADDR_2 0x5e 00147 00148 /** IPv6 multicast uses this prefix */ 00149 #define LL_IP6_MULTICAST_ADDR_0 0x33 00150 #define LL_IP6_MULTICAST_ADDR_1 0x33 00151 00152 /** MEMCPY-like macro to copy to/from struct eth_addr's that are local variables 00153 * or known to be 32-bit aligned within the protocol header. */ 00154 #ifndef ETHADDR32_COPY 00155 #define ETHADDR32_COPY(dst, src) SMEMCPY(dst, src, ETH_HWADDR_LEN) 00156 #endif 00157 00158 /** MEMCPY-like macro to copy to/from struct eth_addr's that are no local 00159 * variables and known to be 16-bit aligned within the protocol header. */ 00160 #ifndef ETHADDR16_COPY 00161 #define ETHADDR16_COPY(dst, src) SMEMCPY(dst, src, ETH_HWADDR_LEN) 00162 #endif 00163 00164 #define eth_addr_cmp(addr1, addr2) (memcmp((addr1)->addr, (addr2)->addr, ETH_HWADDR_LEN) == 0) 00165 00166 #ifdef __cplusplus 00167 } 00168 #endif 00169 00170 #endif /* LWIP_HDR_PROT_ETHERNET_H */
Generated on Sun Jul 17 2022 08:25:24 by
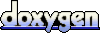